Are you working with multiple devices like iPhone, Android and Web then take a look at this post that explains you how to develop a RESTful API in PHP. Representational state transfer (REST) is a software system for distributing the data to different kind of applications. The web service system produce status code response in JSON or XML format.
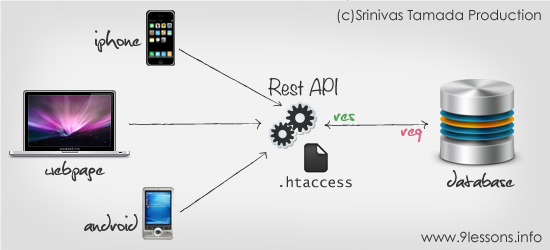

New Tutorial: Create a RESTful services using Slim PHP Framework
Developer
Database
Sample database users table columns user_id, user_fullname, user_email, user_password and user_status.
CREATE TABLE IF NOT EXISTS `users`
(
`user_id` int(11) NOT NULL AUTO_INCREMENT,
`user_fullname` varchar(25) NOT NULL,
`user_email` varchar(50) NOT NULL,
`user_password` varchar(50) NOT NULL,
`user_status` tinyint(1) NOT NULL DEFAULT '0',
PRIMARY KEY (`user_id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
(
`user_id` int(11) NOT NULL AUTO_INCREMENT,
`user_fullname` varchar(25) NOT NULL,
`user_email` varchar(50) NOT NULL,
`user_password` varchar(50) NOT NULL,
`user_status` tinyint(1) NOT NULL DEFAULT '0',
PRIMARY KEY (`user_id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
Rest API Class: api.php
Contains simple PHP code, here you have to modify database configuration details like database name, username and password.
<?php
require_once("Rest.inc.php");
class API extends REST
{
public $data = "";
const DB_SERVER = "localhost";
const DB_USER = "Database_Username";
const DB_PASSWORD = "Database_Password";
const DB = "Database_Name";
private $db = NULL;
public function __construct()
{
parent::__construct();// Init parent contructor
$this->dbConnect();// Initiate Database connection
}
//Database connection
private function dbConnect()
{
$this->db = mysql_connect(self::DB_SERVER,self::DB_USER,self::DB_PASSWORD);
if($this->db)
mysql_select_db(self::DB,$this->db);
}
//Public method for access api.
//This method dynmically call the method based on the query string
public function processApi()
{
$func = strtolower(trim(str_replace("/","",$_REQUEST['rquest'])));
if((int)method_exists($this,$func) > 0)
$this->$func();
else
$this->response('',404);
// If the method not exist with in this class, response would be "Page not found".
}
private function login()
{
..............
}
private function users()
{
..............
}
private function deleteUser()
{
.............
}
//Encode array into JSON
private function json($data)
{
if(is_array($data)){
return json_encode($data);
}
}
}
// Initiiate Library
$api = new API;
$api->processApi();
?>
require_once("Rest.inc.php");
class API extends REST
{
public $data = "";
const DB_SERVER = "localhost";
const DB_USER = "Database_Username";
const DB_PASSWORD = "Database_Password";
const DB = "Database_Name";
private $db = NULL;
public function __construct()
{
parent::__construct();// Init parent contructor
$this->dbConnect();// Initiate Database connection
}
//Database connection
private function dbConnect()
{
$this->db = mysql_connect(self::DB_SERVER,self::DB_USER,self::DB_PASSWORD);
if($this->db)
mysql_select_db(self::DB,$this->db);
}
//Public method for access api.
//This method dynmically call the method based on the query string
public function processApi()
{
$func = strtolower(trim(str_replace("/","",$_REQUEST['rquest'])));
if((int)method_exists($this,$func) > 0)
$this->$func();
else
$this->response('',404);
// If the method not exist with in this class, response would be "Page not found".
}
private function login()
{
..............
}
private function users()
{
..............
}
private function deleteUser()
{
.............
}
//Encode array into JSON
private function json($data)
{
if(is_array($data)){
return json_encode($data);
}
}
}
// Initiiate Library
$api = new API;
$api->processApi();
?>
Login POST
Displaying users records from the users table Rest API URL http://localhost/rest/login/. This Restful API login status works with status codes if status code 200 login success else status code 204 shows fail message. For more status code information check Rest.inc.php in download script.
private function login()
{
// Cross validation if the request method is POST else it will return "Not Acceptable" status
if($this->get_request_method() != "POST")
{
$this->response('',406);
}
$email = $this->_request['email'];
$password = $this->_request['pwd'];
// Input validations
if(!empty($email) and !empty($password))
{
if(filter_var($email, FILTER_VALIDATE_EMAIL)){
$sql = mysql_query("SELECT user_id, user_fullname, user_email FROM users WHERE user_email = '$email' AND user_password = '".md5($password)."' LIMIT 1", $this->db);
if(mysql_num_rows($sql) > 0){
$result = mysql_fetch_array($sql,MYSQL_ASSOC);
// If success everythig is good send header as "OK" and user details
$this->response($this->json($result), 200);
}
$this->response('', 204); // If no records "No Content" status
}
}
// If invalid inputs "Bad Request" status message and reason
$error = array('status' => "Failed", "msg" => "Invalid Email address or Password");
$this->response($this->json($error), 400);
}
{
// Cross validation if the request method is POST else it will return "Not Acceptable" status
if($this->get_request_method() != "POST")
{
$this->response('',406);
}
$email = $this->_request['email'];
$password = $this->_request['pwd'];
// Input validations
if(!empty($email) and !empty($password))
{
if(filter_var($email, FILTER_VALIDATE_EMAIL)){
$sql = mysql_query("SELECT user_id, user_fullname, user_email FROM users WHERE user_email = '$email' AND user_password = '".md5($password)."' LIMIT 1", $this->db);
if(mysql_num_rows($sql) > 0){
$result = mysql_fetch_array($sql,MYSQL_ASSOC);
// If success everythig is good send header as "OK" and user details
$this->response($this->json($result), 200);
}
$this->response('', 204); // If no records "No Content" status
}
}
// If invalid inputs "Bad Request" status message and reason
$error = array('status' => "Failed", "msg" => "Invalid Email address or Password");
$this->response($this->json($error), 400);
}
Users GET
Displaying users records from the users table Rest API URL http://localhost/rest/users/
private function users()
{
// Cross validation if the request method is GET else it will return "Not Acceptable" status
if($this->get_request_method() != "GET")
{
$this->response('',406);
}
$sql = mysql_query("SELECT user_id, user_fullname, user_email FROM users WHERE user_status = 1", $this->db);
if(mysql_num_rows($sql) > 0)
{
$result = array();
while($rlt = mysql_fetch_array($sql,MYSQL_ASSOC))
{
$result[] = $rlt;
}
// If success everythig is good send header as "OK" and return list of users in JSON format
$this->response($this->json($result), 200);
}
$this->response('',204); // If no records "No Content" status
}
{
// Cross validation if the request method is GET else it will return "Not Acceptable" status
if($this->get_request_method() != "GET")
{
$this->response('',406);
}
$sql = mysql_query("SELECT user_id, user_fullname, user_email FROM users WHERE user_status = 1", $this->db);
if(mysql_num_rows($sql) > 0)
{
$result = array();
while($rlt = mysql_fetch_array($sql,MYSQL_ASSOC))
{
$result[] = $rlt;
}
// If success everythig is good send header as "OK" and return list of users in JSON format
$this->response($this->json($result), 200);
}
$this->response('',204); // If no records "No Content" status
}
DeleteUser
Delete user function based on the user_id value deleting the particular record from the users table Rest API URL http://localhost/rest/deleteUser/
private function deleteUser()
{
if($this->get_request_method() != "DELETE"){
$this->response('',406);
}
$id = (int)$this->_request['id'];
if($id > 0)
{
mysql_query("DELETE FROM users WHERE user_id = $id");
$success = array('status' => "Success", "msg" => "Successfully one record deleted.");
$this->response($this->json($success),200);
}
else
{
$this->response('',204); // If no records "No Content" status
}
}
{
if($this->get_request_method() != "DELETE"){
$this->response('',406);
}
$id = (int)$this->_request['id'];
if($id > 0)
{
mysql_query("DELETE FROM users WHERE user_id = $id");
$success = array('status' => "Success", "msg" => "Successfully one record deleted.");
$this->response($this->json($success),200);
}
else
{
$this->response('',204); // If no records "No Content" status
}
}
Chrome Extention
A Extention for testing PHP restful API response download here Advanced REST client Application
.htaccess code
Rewriting code for friendly URLs. In the download code you just modify htaccess.txt to .htaccess
<IfModule mod_rewrite.c>
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-d
RewriteCond %{REQUEST_FILENAME} !-s
RewriteRule ^(.*)$ api.php?rquest=$1 [QSA,NC,L]
RewriteCond %{REQUEST_FILENAME} -d
RewriteRule ^(.*)$ api.php [QSA,NC,L]
RewriteCond %{REQUEST_FILENAME} -s
RewriteRule ^(.*)$ api.php [QSA,NC,L]
</IfModule>
RewriteEngine On
RewriteCond %{REQUEST_FILENAME} !-d
RewriteCond %{REQUEST_FILENAME} !-s
RewriteRule ^(.*)$ api.php?rquest=$1 [QSA,NC,L]
RewriteCond %{REQUEST_FILENAME} -d
RewriteRule ^(.*)$ api.php [QSA,NC,L]
RewriteCond %{REQUEST_FILENAME} -s
RewriteRule ^(.*)$ api.php [QSA,NC,L]
</IfModule>
pls post more about api i want to learn that
ReplyDeleteIt's very nice , what about XML RPC
ReplyDeletereally usefull to me.. thanks a lot :)
ReplyDeleteNice. 'll give it a try
ReplyDeletethanks for the article :)
ReplyDeleteThat a very well but I want some more example & declaration pls provide this.Thank u
ReplyDeleteKool man nice work .. i have used this one
ReplyDeleteGood job ! But it would be much better if you indent the Code with Tabs, as the code above is little difficult to understand
ReplyDeleteI will definitely try it out to develop demo API by myself and will try the same API for the android app development.
ReplyDeleteThank for sharing detailed article.
awesome article....!
ReplyDeleteamazing!!
ReplyDeleteVery good tutorial! Thanks a lot!
ReplyDeleteMuito bom cara! Parabéns.
ReplyDeleteVery good post as usual! Good work, Arun! :)
ReplyDeleteCould you explain how to get data? As I see in this script, in URL you send a name, that name is the name on the function. Further more _request is set to array. So you wrap everything in an array?? But then, how to extract, so you get correct function?? IM CONFUSED! And where to extend this so I can claim and API key ?
ReplyDelete@KFllash32 : This is little bit tricky but more user friendly, api(api.php) demo class wrote like this way query string as a function. But you can write your own class insteed of api.php. Validating api key or headers and all up to you.
ReplyDeleteI have some experiences in this, so I figures out a way, but the Login function in the api.php will not work for most users because you also need email and username input. And something strange.
ReplyDeleteYou write this: $this->response('',204). And this will return nothing at all, because first param is '' (empty) and in the function you return data (data is the first param left empty). So what is the point with this one?
Good..article
ReplyDeleteEvery nice ... big thanks if i can have more tutorial
ReplyDeletehow to highlight your codes?
ReplyDeleteyeah looks nice dude
ReplyDeleteYour diagrams are impressive, what tool you use for that?
ReplyDeleteI heard PHP is not good for applying RESTfull because it does not integrate natively query functionality DELETE and PUT
ReplyDeleteIt's really?
Well, that upto you what you think. But PHP is the best option for HTTP request in SSS.
DeleteHello Kumar, I appreciate your comment and contribution on this article. Please if one need to pass a parameter to the login function in this article, how do I do that through the url? Assume you are calling the service from an android application and you need to pass the email and password to the url as parameter. I will appreciate a respond to this question please.
Deletethank this blog always saves me.I love your jobs and i stay tune.I ll try this API because i have a simillar projet and this will help i think so
ReplyDeleteawesome
ReplyDeletewell nice post keep it up for us to learn more...
ReplyDeleteCan any one explain this line in Sample database "users"
ReplyDeleteENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ;
What did you use in making the illustration:
ReplyDeletehttps://lh6.googleusercontent.com/-u9hFxEK0OS8/T6a9yHaniHI/AAAAAAAAF_Y/prEsvdWrNtI/s550/rest.png
Adobe illustration
ReplyDeleteWhat about security :))?
ReplyDeleteWrite is you can how to use also with REST OAuth.
Also do you know why some API uses such url
site.com/api/create.json
why the use dot?
nice sir ji
ReplyDeletePost more information regarding the REST modules and I want to integrate it with my application
ReplyDeletegood one
ReplyDeletenice sir.. Thank you for sharing always your ideas to us ..
ReplyDeletevery nice. thanks..
ReplyDeletei am having a problem getting Params such as email and Password for the sample Post function login()
ReplyDeletecould it be htaccess?
email and password keep coming in as null
any suggestions? I have Sample Code
Well, this example ios really nice and working so far. I just wanna know if it's possible to request for a special user this way:
ReplyDeletehttp://localhost/rest/users/14
GET: List info for user with ID of 14
How do I manage it with your code? Is that possible?
how to pass request for deleteuser and login in url..
ReplyDeletepls explain someone
This is really nice and easy plugin. Can u plz tell me how to call 'login' function with POST menthod. Plz it's urgent. It wil be really great if you reply ASAP
ReplyDeleteThis tutorial is not in detail for the person who is new to API. I was trying to create an API that inserts records to my db. I know i could use this, But i'm struggling
ReplyDeleteHi,
ReplyDeleteCan anyone tell how to use this api? Do I need to call it from other application? If yes, then how?
I always see Get request. How to change it to POST ?
ReplyDeleteCould you please post an example for inserting data using POST.
ReplyDeletecould you please post insert & fetch data using xml with rest
ReplyDeleteCan you explain the post methods. Means how can i post email and password
ReplyDeletethanks
thanks.... its enough to start with rest api for begginers
ReplyDeleteHelpful !!
ReplyDeleteHello,
ReplyDeleteI am new to php, how to execute this program ?
Can any one help.
what are de .ds_store and .htacces files for? regards!
ReplyDeleteTo execute the program you must have php installed, and a web server.
ReplyDeleteI'd suggest you look for tutorials for beginning php first. Then when you are comfortable, and know what REST is, then come back
not working how to see result of http://localhost/rest/users/??
ReplyDeleteHow to call it for testing...
ReplyDeletehi!
ReplyDeletecan u explain me how use credential for calling REST resources after login?
Nice job Arun :D
ReplyDeletebut are you sure that the returns in json?
for example, i use your Rest.inc.php file for construct an api, and this file return that's
string(508) " object(Api)#1 (8) { ["data"]=> string(0) "" ["db":"Api":private]=> resource(4) of type (mysql link) ["_allow"]=> array(0) { } ["_content_type"]=> string(16) "application/json" ["_request"]=> array(3) { ["rquest"]=> string(5) "login" ["Email"]=> string(15) "[email protected]" ["Password"]=> string(5) "12345" } ["_resp"]=> array(1) { ["Id"]=> string(1) "1" } ["_method":"REST":private]=> string(0) "" ["_code":"REST":private]=> int(200) } "
is json?
Very helpful... I can test it in php and working perfectly. But unable to call it in windows phone.
ReplyDeleteSo, Can you tell me how can I call it in Windows Phone apps.
Helpful for beginners. Great!!!
ReplyDeleteMake sure to enable mod_rewrite in httpd.conf
ReplyDeleteI am getting this error:
ReplyDeleteNotice: Undefined index: rquest in C:\server\www\jrserver.dev\public_html\rest\api.php on line 69
Thanks for the info !
ReplyDelete@eureckou: you should pass the parameter rquest and value to that.
ReplyDeletefor example. "http://localhost/rest/api.php?rquest=users" so that your rquest parameter will be passed and the value will be accessed in processApi(). Then the action will be taken according to your request.
Hi, thanks for that good.. it took me a while to find any good and easy to use examples..
ReplyDeleteOne question.. When I use to Chrome extension to test the web service, the login and deleteUser function is not working.. During the debbuging I found out that $_GET and $_POST is just empty.. any idea why that happens?
plz tell me form where we can post the value and how can get post value within function....
ReplyDeleteGood tutorial but you know that the POST implementation is not working, right? There are a lot of people asking for help in the comments but I guess the author forgot about this post... It's a shame.
ReplyDeletecan anyone help me on how to access the service methods from the client code.
ReplyDeleteThere is no impementation of any methods to add a user so to use the API you need to first add data into your database's 'users' table. Use an online md5 generator to create the password and make sure to have the field 'user-status' set to 1 if you want to get the data. To login (/login) use POST and variables 'pwd' and 'email' in Payload, to see users (/users use GET. To delete, no idea. Seems like dELETE is not accepted on my system (Chrome/Windows). I hope this will help some of you.
ReplyDeleteTo delete users, there is an error: in Rest.inc.php, within the inputs() method, DELETE must be treated as PUT, not as GET as it is currently set.
ReplyDeleteReally helpful dude..thanks a lot.. :)
ReplyDeletecurl testing
ReplyDelete$ch = curl_init('http://api.local/deleteUser?id=2');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
echo $result = curl_exec($ch);
curl testing
ReplyDelete$ch = curl_init('http://api.local/deleteUser?id=2');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
echo $result = curl_exec($ch);
curl testing
ReplyDelete$ch = curl_init('http://api.local/deleteUser?id=2');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "DELETE");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
echo $result = curl_exec($ch);
Good I'd like to know how to call the api from jquery mobile application
ReplyDeleteHi,
ReplyDeleteCan you tell me please where from download Rest.inc.php?
To use it without problems make sure you have:
ReplyDeleteapt-get install php5-curl
require_once("Rest.inc.php");
ReplyDeletefrom where i get it?
Not served in WAMPSERVER gives me a problem in the require_once ("Rest.inc.php");
ReplyDeleteVery helpful tutorial, thanks very much!
ReplyDeletevery helpful
ReplyDeleteI am new to SOAP and REST which do you recommend me to learn and is easy to learn.
ReplyDeleteHow do I use the private function login() section. If i have 2 inputs for username and password and I post the email and password to api.php how can I log that user in through private function login()?
ReplyDeleteGetting a 404 Not Found?
ReplyDeleteMake sure to enable mod_rewrite in httpd.conf
we want to create api for prepaid mobile recharges & other kindly provide suggession to get it
ReplyDeleteHi,
ReplyDeleteI am trying to use this code and actually i am getting an notice like..
Notice: Undefined index: rquest in C:\xampp\htdocs\rest\api.php on line 69
Can you please explain what the problem..
nice post... Very helpful
ReplyDeletehi guys. i am new to PHP. here i am using this api.php for webservice consuming to mobiles.can any one please tell me how can i use POST method for login function.
ReplyDeletemy table name: user
column names : email , password
please urgent please.
thanks in advance.
u can also use this to call with the style /users instead of api.php?rquest=users
ReplyDelete$func = trim(str_replace("/","",$_SERVER['REQUEST_URI']));
$func = trim(str_replace("restcomicapp","",$func));
$func = trim(explode("?",$func)[0]);
cool guy !
ReplyDeletehave a nice year !
ben
You dont kwown with means API REST.
ReplyDeleteExample, with same URL:
/resource/333
diferent HTTP VERB (DELETE, POST, PUT, GET), Delete, create, update or GET de resource
hi am getting an error like
ReplyDeleteNotice: Undefined index: rquest in C:\xampp\htdocs\rest\api.php on line 69
What should I do
Nice tutorial.Thanks.Keep up the good work
ReplyDeleteHi, thanks alot but i am new beginer with restful, can you help me how test demo?
ReplyDeleteThank you for this! Simple, but it works for my little project.
ReplyDeletecan you explain me why do you get function by $func = strtolower(trim(str_replace("/","",$_REQUEST['rquest'])));
ReplyDeleteI don't find any rquest variable.
how to upload byte array of image in php?
ReplyDeletethaks,
srinivas
i'm new to php.here is api.php is a client code & Rest.cin.php is a server code? wt is the use of htaccess.txt and how to run?
ReplyDeletehi its ok how to i run this script in my localhost
ReplyDeletethanks for simple lessons
ReplyDeletenice...very useful
ReplyDeletethis is awesome tutorial for learning api web application.it is very useful for developing android application.
ReplyDeleteWe can make this without using REST keyword, So how we can ensure that its used REST API. Is there have any specific keyword for REST ? Please someone explain this.
ReplyDeletehello I am not able to get response , plz help me
ReplyDeleteplz help me i am not geting response
ReplyDeletemy web project name = rest
request = http://localhost/rest/users
htaccess = file same in example
Thanks a lot Arun, it really helped a lot.
ReplyDeleteCleraed my basic confusion about rest api and solved my purpose too.
Keep it up.
Nice Tutorial
ReplyDeletenot working..
ReplyDeleteHello... Thanks for the tutorial...
ReplyDeleteplease how do i get to test what you have explained in this tutorial?
please i have tried implementing this but i i have not been able to consume the api in a client file.
ReplyDeleteLogin is not worked for me. But i changed something like following...Now its working fine..
ReplyDeletePlease use $_REQUEST to get params,
if(isset($_REQUEST['email']) && isset($_REQUEST['pwd'])){
$email = $_REQUEST['email'];
$password = $_REQUEST['pwd'];
// Input validations
if(!empty($email) and !empty($password)){
//keep all queries
}
}
ultimate tuts dude..keep it up.thnx alot
ReplyDeletegud tut..
ReplyDeletecan u help how to send response in json/xml
if user send url as format/json or format/xml
nice post to learn
ReplyDeleteSo this is what REST does. Thank you!
ReplyDeleteHi I just Use you Rest API and also using rest client but every time I getting Response does not contain any data. Please Help
ReplyDeleteclient side code for login:
ReplyDelete$user_data = array();
$user_data['email'] = '[email protected]';
$user_data['pwd'] = md5('test123');
// cURL code
$ch = curl_init('http://localhost/rest/api.php?request=login');
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, $user_data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, TRUE);
$response = curl_exec($ch);
echo $response;
$http_code = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
echo $http_code;
HI , This is a very nice tutorial. GET request is working fine. But POST request is not working properly. Here is the solution,I tested this in mozilla rest client addon. Please enter json format data in request body [{"email":"[email protected]","pwd":"123456"}].
ReplyDeleteFor reading that json code we need to add few lines in api.php.
$request_body = file_get_contents('php://input');
$json = json_decode($request_body);
$email = $json[0]->email;
$password = $json[0]->pwd;
Thats all. In mozilla rest client addon at the time of executing pls select method type POST.
Thanks..
Thanks for the lesson!
ReplyDeleteIn advance rest client->chrom i tried,
http://localhost/rest/login/, to post the inputs, i face 400 Bad Request error when inputing the following json information:
{
"email":"[email protected]",
"password":"test123"
}
i wonder to know the way json should be inputed for posting the login information.
Hi, is really http://localhost/rest/deleteUser/ even rest?
ReplyDeleteShouldn't be somthing like DELETE http://localhost/rest/users/21 to be able to call it Rest?
Where is the Uniform Interface in all this?
You really should read this carefully: http://en.wikipedia.org/wiki/Representational_state_transfer
problem with your userID???
ReplyDeletetry this:
http://localhost/rest/users?id=2
don't use:
http://localhost/rest/users/2
try with:
ReplyDeletehttp://localhost/rest/users?id=2
don't use:
http://localhost/rest/users/2
it's work
Hi, it was nice work for me Thanks
ReplyDeletei am happy to see your new design
ReplyDeleteHello! just start with this the rest api, I saw your code and if I understand but as implemented on a small website.
ReplyDeleteThis error code is too generic. Can you give more info, a complete description of the error message, a picture, something more?
ReplyDeleteWithout more info, I won't be able to help you
Thanks mate
how to run dis application?
ReplyDeletethankyou very much for your sharing ! ^_^
ReplyDeletecan you explain to me how htaccess file works?
Hi,
ReplyDeleteI am getting
Notice: Undefined index: email in D:\XMAPP\xampp\htdocs\rest\api.php on line 92
Notice: Undefined index: pwd in D:\XMAPP\xampp\htdocs\rest\api.php on line 93
{"status":"Failed","msg":"Invalid Email address or Password"}
When i using http://localhost/rest/login API with post method
Good Article.
ReplyDeleteanything to insert data in to database???
ReplyDeletenice post, so how to access this rest on Andorid with java code?? would you mind to give an example?? i had a code with web service server side on php then client side on java. but i didnt apply API REST to my code. im just a newbie,
ReplyDeletecan u please explain for beginners
ReplyDeleteHow to use html form to post data to the function
ReplyDeleteThanks for the great tutorial!
ReplyDeleteI like this tutorial. How to use this on web app?
ReplyDeletethanks in advance
How to use html form to post data to the function.Thanks for the great tutorial!
ReplyDeleteIt's very nice , what about XML RPC
ReplyDeleteThanks
nice tutorial really helpfull
ReplyDeletewhy are we using htaccess configuration ? im a newbie
ReplyDeleteI created one record in the users table and then tried to run the api "localhost/rest/api.php?rquest=1 but I got a blank page. if I want to retrieve the data from the users database, how do I do it?
ReplyDeleteme too, i received the blank page please need someone to help.
Deleteme too i receieved a blank page for this tutorial please someone to help
Deletethank you
user httpRequester or postman to post,get,delete,put data, rquest is ment to find the request method and it need not be in the url.
DeleteHi i run the above script but i am getting an error, 'Fatal error: Class 'Rest' not found in C:\xampp\htdocs\rest\api.php on line 4 '. Please help me to fix this, thanks in advance :)
ReplyDeleteMr Srinvas Tamada. Are you aware the deleteUser function doest function? Whenever a request is made (using the above recommended API client for chrome, nothing happens. It just returns a 204 no content error. I found out that the $id assignment doesn't work because $this->_request['Id] is blank. A searched the web and found out that this has to do with a complication with most web servers not allowing Delete requests, or a complication with PHP and delete requests, either way the delete request is the problem. I confirmed this by simply switch the request method for the deleteuser function to POST instead of delete and it worked. I'm not sure what you did to you Dev environment for it to work, or what environment you're using (I'm using the lateset XAMPP, BTW). Would you please correct me or your tutorial?
ReplyDeleteThanks for the great tutorial nonetheless.
THanks!!! very very good!
ReplyDeleteIt really helps a lot. Thanks!
ReplyDeleteNice tutorial for REST beginners, thanks!
ReplyDeleteHow to pass parmater for insert data
ReplyDeleteNice tutorial for beginners, Thanks.
ReplyDeleteI am using same method for my api but stuck now.
i have two urls
1. api/users - to get all users which is done already
2. api/users/search/id - to search user with id ( any keyword )
can someone guide me on how to get it done ?
How to create a a function for url like api/users/edit/1 ?
ReplyDeleteit simply update the user with id 1, values will be passed by POST
Its Realy Usefull api
ReplyDeleteSuppose : i want to access data from a server through REST api System and the server link is www.test.com
ReplyDeleteI do have the username and password .
Now how to fetch the data...
I tried using the firefox Add-On RESTclient 2.0.3 and it works...but i can't write the code of my own...
i am trying since last week but unfortunately still unsuccessful :(
Can you please help me....i haven't had much knowledge about REST..beginner you ca say.
Thank you
ReplyDeleteI ll try this
How to Delete user from this
ReplyDeletehttp://localhost/CeederOn/rest/api.php?rquest=delete
how to pass id actual i wanna ask
Nice Tutorial
ReplyDeleteHow can I call it with curl can you please help me?
$func = strtolower(trim(str_replace("/","",$_REQUEST['rquest'])));
ReplyDeleteCan Some one help me in understanding, what this code line above does, cz am getting an error when ever I hit the api method
the error is undefined index : rquest
thanks
can you please explain how this api or urls will call in mobiles.what will be the connection?
ReplyDeleteplease explain how the connection was built between api and android or ios or anything
ReplyDeletehow the urls are called from mobiles and connect to mysql and get the results?
ReplyDeletegreat post , well explained and super understandable ... thank you very much !!!
ReplyDeletefrom Paraguay
It does not work for GET http://localhost/services/customer/1 (This is actual REST calls)
ReplyDeleteBut it works with http://localhost/services/customer?id=1
What shall we do to make it work with such URL?
can you explain using CURL..
ReplyDeletehow can we do that without using Chrome exetention
Simply, fantastic! Thanks
ReplyDeleteHi,
ReplyDeletePlease tell me anyone,
get is working.
how to test delete using chrome extension?
how to test login in chrome
ReplyDeletegood job
ReplyDeleteSomething that worth reading... Thanx for the lesson
ReplyDeletethanks for the tut it worked properly... but i have a prblm with .htaccess files i just want to add a new file like api.php to .htaccess. Can you please help me with these...
ReplyDeleteThanks
ReplyDeletehow to get youtube api and show in our web ?
ReplyDeletehow can i use this service with post key value parameter using poster
ReplyDeleteHere is the add user(insert):
ReplyDeleteprivate function adduser(){
// Cross validation if the request method is GET else it will return "Not Acceptable" status
if($this->get_request_method() != "POST"){
$this->response('',406);
}
$name = isset($_POST['name']) ? mysql_real_escape_string($_POST['name']) : "";
$email = isset($_POST['email']) ? mysql_real_escape_string($_POST['email']) : "";
$password = isset($_POST['pwd']) ? mysql_real_escape_string($_POST['pwd']) : "";
$status = isset($_POST['status']) ? mysql_real_escape_string($_POST['status']) : "";
$sql = mysql_query("INSERT INTO `users` (`user_id`, `user_fullname`, `user_email`, `user_password`, `user_status`) VALUES (NULL, '$name', '$email', '$password', '$status');", $this->db);
if($sql){
$success = array('status' => "Success", "msg" => "Successfully inserted");
$this->response($this->json($success),200);
}else{
$this->response('',204); // If no records "No Content" status
}
301 error when i calling api.php?request=users
ReplyDeleteHow can i create a page except api.php? I create a page mails.php but it is not working due to some htaccess issue. How can i solve it. I want to use another page for data insertion using cron job on the server. Please let me know, how can i execute the another page?
ReplyDeleteVery useful tutorial. But I have a problem in the login POST method - the email and password parameters are being passed empty as it is not entering in the IF condition.... and I am using Google Advanced Rest Client. Any help please?
ReplyDeleteI have a problem with delete method. When i tested using advanced rest client addon in chrome i cant get the id in request in php. Is there any problem with api.php.
ReplyDeleteWhy does the POST method only work using x-www-form-urlencoded? Is it possible to send the parameters in JSON? I tried using JSON format but the parameters were sent empty. Any help please?
ReplyDeleteThanks, it works for me, and do help me a lot.
ReplyDeleteplease provide the rest api code using codeigniter.
ReplyDeleteThanks
ReplyDeleteCan some provide the NGINX configuration for this API.
ReplyDeleteI m running on nginx server
I am getting the error Undefined index: email ,pwd on line 90,91
ReplyDelete$email="[email protected]";
$pwd="abcd";
$strPost= 'email='.$email.'&pwd='.$pwd;
in curl I am passing values like this
curl_setopt($ch, CURLOPT_POSTFIELDS,$strPost);
please help me
How we test the API?
ReplyDeletehttp://localhost/rest/users/ is referring me anything
Hi,
ReplyDeleteI am trying to use this code and actually i am getting an notice like..
Notice: Undefined index: rquest in C:\xampp\htdocs\rest\api.php on line 69
Can you please explain what the problem..
Hi,
ReplyDeleteI am trying to use this code and actually i am getting an notice like..
Notice: Undefined index: rquest in C:\xampp\htdocs\rest\api.php on line 69
Can you please explain what the problem..
good nice tutorial
ReplyDelete
ReplyDeleteNotice: Undefined index: rquest in C:\xampp\htdocs\rest\api.php on line 69
iam getting this error how to handle it
ReplyDeleteNotice: Undefined index: email in C:\xampp\htdocs\rest\api.php on line 89
Notice: Undefined index: pwd in C:\xampp\htdocs\rest\api.php on line 90
{"status":"Failed","msg":"Invalid Email address or Password"}
hi,
ReplyDeletei am use odbc php but i have a problem. can you help me? please
code -- code -- code --
$sorgu="SELECT mno,durum FROM dba.masa";
$sorgu=@odbc_exec($conn,$sorgu);
if (!$sorgu) { echo"Sorgu Hatası"; }
$result = array();
while ($sorgu_yaz = odbc_fetch_array($sorgu)) {
$result[] = $sorgu_yaz;
}
..
$this->response($this->json($result), 200);
..
private function json($data){
if(is_array($data)){
return json_encode($data);
}
}
this is not working pleasee help me
i am not able to change headers to json content type
ReplyDeleteHost: localhost
User-Agent: Mozilla/5.0 (Windows NT 10.0; WOW64; rv:43.0) Gecko/20100101 Firefox/43.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
Hi,
ReplyDeleteIt is very useful for me...Thank you for this article...
I have a issue to close the connection of MySQL. I am using destruct function for close the connection but it is not working...Please help me.
here is the code,
function __destruct() {
mysql_close($this->db);
}
you want use with html?that done using ajax.
ReplyDeleteAuthors, you should answer to those questions asked in your blog, else this REST API a waste of time. I see there is a lot of unanswered questions up there. Please answer those. Thanks.
ReplyDeletePlease try to use latest one http://www.9lessons.info/2014/12/create-restful-services-using-slim-php.html
Deletegiving me 404 error
ReplyDeletethanks for help me
ReplyDeletehttp://localhost/rest/login/, to post the inputs, i face 400 Bad Request error when inputing the following json information:
ReplyDelete{
"email":"[email protected]",
"password":"test123"
}
Hi,
ReplyDeleteI am getting
Notice: Undefined index: email in D:\XMAPP\xampp\htdocs\rest\api.php on line 92
Notice: Undefined index: pwd in D:\XMAPP\xampp\htdocs\rest\api.php on line 93
{"status":"Failed","msg":"Invalid Email address or Password"}
When i using http://localhost/rest/login API with post method
Very helpful
ReplyDeleteAjax php demo username check script.
Very helpful article.Appreciating
ReplyDelete