Now time to work with MongoDB NoSQL database management systems, it stores data into structured JSON like documents with dynamic schemas and improves application performance. This tutorial helps you how to create a simple login page using PHP and MongoDB. Try this download script and implement scalable application.
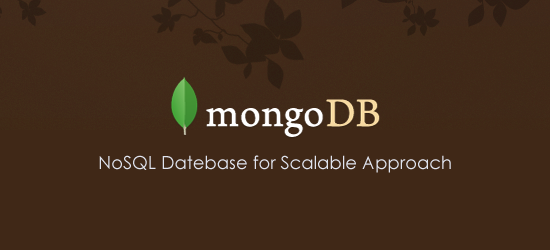
Download Script
Developer
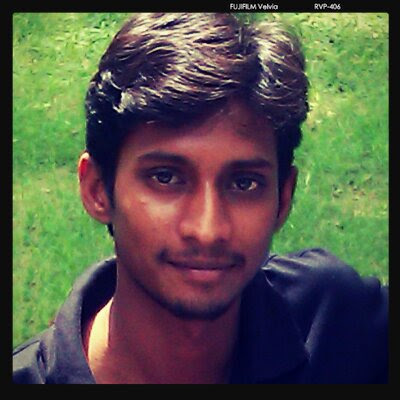
Arun Kumar Sekar
Engineer, Plugin Expert
Chennai, INDIA
Engineer, Plugin Expert
Chennai, INDIA
Installation
Windows
http://docs.mongodb.org/manual/tutorial/install-mongodb-on-windows/
You can download PHP mongo drive/extension dll file from following URL https://github.com/mongodb/mongo-php-driver/downloads. Copy php_mongo.dll file into your PHP extension directory
Linux & Mac
http://docs.mongodb.org/manual/tutorial/install-mongodb-on-ubuntu/
http://docs.mongodb.org/manual/tutorial/install-mongodb-on-ubuntu/
Connecting into MongoDB
PHP code connecting with out Authentication.
<?php
$mongo = new Mongo();
$db = $mongo->selectDB(“test”);
?>
$mongo = new Mongo();
$db = $mongo->selectDB(“test”);
?>
With Authentication
<?php
$mongo = new Mongo(“mongodb://{$username}:{$password}@{$host}”);
$db = $mongo->selectDB(“test”);
?>
$mongo = new Mongo(“mongodb://{$username}:{$password}@{$host}”);
$db = $mongo->selectDB(“test”);
?>
By default MongoDB contains sample database called “test”.
Creating New Database
$db = $mongo->Database_Name;
Note: Technically, you don't need to manually create databases in MongoDB due to its schemaless "lazy" way of creating databases.
Queries
PHP code to get MongoDB databases.
//Get list of databases
$mongo->admin->command(array(“listDatabases” => 1));
//Get list of Collections in test db
$db->listCollections();
$mongo->admin->command(array(“listDatabases” => 1));
//Get list of Collections in test db
$db->listCollections();
Mongo Shell/Terminal/Command Prompt
db.listDatabases
db.test.showCollections
db.test.showCollections
Create Collection(table)
You can created a collection/table but executing following code.
$db->createCollection(“people”,false);
Note: here false referred to unlimitted size, if you give true you have to mention the size of maximum.
Mongo Shell
db.createCollection(“people”,false);
Insert Record
Insert records int MongoDB.
<?php
$people = $db->people;
$insert = array(“user” => “[email protected]”, “password” => md5(“demo_password”));
$db->insert($insert);
?>
$people = $db->people;
$insert = array(“user” => “[email protected]”, “password” => md5(“demo_password”));
$db->insert($insert);
?>
Mongo Shell:
db.people.insert({user:”user_name”,password:”password”});
Update Records
Update records into MongoDB
<?php
$update = array(“$set” => array(“user” => “[email protected]”));
$where = array(“password” => “password”);
$people->update($where,$update);
?>
$update = array(“$set” => array(“user” => “[email protected]”));
$where = array(“password” => “password”);
$people->update($where,$update);
?>
Mongo Shell:
db.people.update({password:”password”},{$set : {user:”[email protected]”}});
HTML Code
<form action="index.php" method="POST">
Email:
<input type="text" id="usr_email" name="usr_email" />
Password:
<input type="password" id="usr_password" name="usr_password" />
<input name="submitForm" id="submitForm" type="submit" value="Login" />
</form>
Email:
<input type="text" id="usr_email" name="usr_email" />
Password:
<input type="password" id="usr_password" name="usr_password" />
<input name="submitForm" id="submitForm" type="submit" value="Login" />
</form>
PHP Code
<?php
$succss = "";
if(isset($_POST) and $_POST['submitForm'] == "Login" )
{
$usr_email = mysql_escape_string($_POST['usr_email']);
$usr_password = mysql_escape_string($_POST['usr_password']);
$error = array();
// Email Validation
if(empty($usr_email) or !filter_var($usr_email,FILTER_SANITIZE_EMAIL))
{
$error[] = "Empty or invalid email address";
}
if(empty($usr_password)){
$error[] = "Enter your password";
}
if(count($error) == 0){
$con = new Mongo();
if($con){
// Select Database
$db = $con->test;
// Select Collection
$people = $db->people;
$qry = array("user" => $usr_email,"password" => md5($usr_password));
$result = $people->findOne($qry);
if($result){
$success = "You are successully loggedIn";
// Rest of code up to you....
}
} else {
die("Mongo DB not installed");
}
}
}
?>
$succss = "";
if(isset($_POST) and $_POST['submitForm'] == "Login" )
{
$usr_email = mysql_escape_string($_POST['usr_email']);
$usr_password = mysql_escape_string($_POST['usr_password']);
$error = array();
// Email Validation
if(empty($usr_email) or !filter_var($usr_email,FILTER_SANITIZE_EMAIL))
{
$error[] = "Empty or invalid email address";
}
if(empty($usr_password)){
$error[] = "Enter your password";
}
if(count($error) == 0){
$con = new Mongo();
if($con){
// Select Database
$db = $con->test;
// Select Collection
$people = $db->people;
$qry = array("user" => $usr_email,"password" => md5($usr_password));
$result = $people->findOne($qry);
if($result){
$success = "You are successully loggedIn";
// Rest of code up to you....
}
} else {
die("Mongo DB not installed");
}
}
}
?>
-like it !
ReplyDeletevery clear thank you arun. One question mqsql vs mongodb which one is best.
ReplyDeletethats depened on project
Deletecool :)
ReplyDeleteHi, like the script but instead of mysql_escape_string() why not use mysql_real_escape_string() because mysql_escape_string() seems to be deprecated
ReplyDeleteIt is mongo+php form than why should we use mysql_real_escape_string()
DeleteThanks, very nice.
ReplyDeleteI aksed it and you make it! You are my php lover :)
ReplyDeleteThis is the first no sql database that i learnt :)
ReplyDeleteHi, liked the script but mysql_escape_string() is deprecated so i think you would rather use mysql_real_escape_string hey otherwise great article
ReplyDeleteGreat, its good starting point to learn mongodb :)
ReplyDeleteVery nice and helpful tutorial. Ty and keep up!
ReplyDeleteThis me helpful, http://anandafit.info/2011/10/03/introduction-to-mongodb/
ReplyDeleteThanks for this good tutorial on Mongo DB. Its very useful. I hope PHP with Mongo DB is more scalable than with mysql.
ReplyDeleteNice Post
ReplyDeletegood one
ReplyDeletevery informative...good job..
ReplyDeletethanks 4 sharing this awesome tutorial
ReplyDeletenice
ReplyDeleteDoes mysql_real_escape_string work without specifying mysql credentials?
ReplyDeleteexcellent
ReplyDeleteNice Post ...
ReplyDeleteVery nice post!!
ReplyDeleteI think
ReplyDelete$db->insert($insert);
Should be
$people->insert($insert);
In "Insert Record" part?
Thanks for that.
ReplyDeleteWhy you used mysql escape function mysql_escape_string() for escaping. I do not understand. Has mongo extension any escaping method?
Mongo() has been DEPRECATED as of version 1.3.0. Relying on this feature is highly discouraged. Please use MongoClient instead.
ReplyDeletehttp://in3.php.net/manual/en/class.mongo.php
~ Bhupendra Yadav
http://www.youtube.com/watch?v=URJeuxI7kHo
ReplyDeletegreat tutorial, thanks :)
ReplyDeletesuper ;)
ReplyDeletePhp-Mysql, free course, tutorials code and lessons, learn how to use Php programming language and Mysql database.
ReplyDeleteIt would be gr8 if u can take a normal mysql schema say a blog db and then convert that schema to nosql document format. That will help a lot of people to get to know the power of nosql at the same time its negative role
ReplyDeleteEcellant aticle
ReplyDeleteThis comment has been removed by a blog administrator.
ReplyDeleteis there a need for a mysql escape on a NoSQL script?
ReplyDeleteNice article
ReplyDeleteThanks for the tutorial, now get rid of those special quotes in your sample code. Never c/p from microsoft products!
ReplyDeleteWhat about moving an existing appllication into MongoDB by just editing the config.php class itself to point to the MongoDB servers?
ReplyDelete$people = $db->people;
ReplyDelete$insert = array(“user” => “[email protected]”, “password” => md5(“demo_password”));
$db->insert($insert);
instead of this
$people = $db->people;
$insert = array(“user” => “[email protected]”, “password” => md5(“demo_password”));
$people->insert($insert);
Correct this :)
Does mangodb supports for shared hosts?
ReplyDeleteI will try installing MongoDB tonight and study this. Thank you
ReplyDeletesuper ;) super ;) super ;)
ReplyDeleteHi,.
ReplyDeletePHP version -5.5.12
apache - 2.4.9
windows-32bit
i have tried with many dll files but it is not working, can you please help me out how to get currect dll file for above versions
Thanks
Vinayak
Thanks ...very informative and to the point.
ReplyDeletethank's .. it's work for me ..
ReplyDeleteFirst of all Thanks for writing simple n wonderful code.
ReplyDeleteOne Mistake in Insert Record code
Change $db->insert($insert);
to $people->insert($insert);
SUBRAHMANYAM
DeleteCan i have your email please.
How to Get Id From url with Using a Php code Can u help me
ReplyDelete