It is very simple to trigger any camera device and show the resulting output. This has been discussed in my article to take multiple photos with delete action using Ionic and AngularJS. Here is a problem. You can only see the image temporarily, since it has not been stored in any storage. Today’s article will explain to you how to store the captured image in a database, so that you can use it later. I’ll be using RESTful API to achieve this. Look into the demo below and also the code to see how to connect with RESTful API to store any captured image into a database.
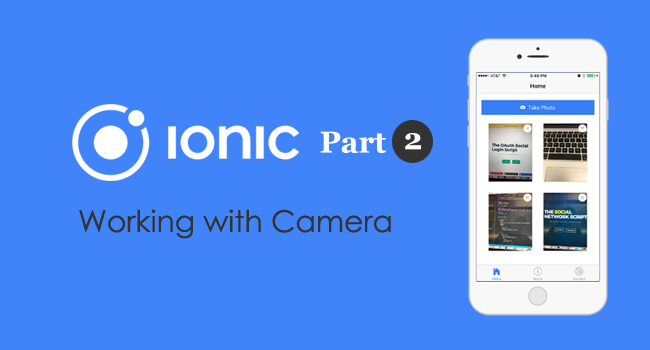
Part One: Ionic 3 and Angular 2: Using the Native Camera, Take Multiple Photos with Delete Action.
Video Tutorial - Ionic Angular Native Camera with Restful Upload.
Database
Here we are storing the image in Base64 format.
CREATE TABLE imagesData(
img_id INT PRIMERY KEY AUTO_INCREMENT,
b64 TEXT,
user_id_fk INT
);
img_id INT PRIMERY KEY AUTO_INCREMENT,
b64 TEXT,
user_id_fk INT
);
Install Latest Ionic & Cordova
Ionic has been updated with new libraries and it supports hotloading with IonicDevApp.
$npm install ionic cordova -g
Create AuthService Provider
You have to create an injectable component for API autentication. The following ionic command help you to create files automatically.
$ionic g provider authService
authService.ts
Include post data function for HTTP post access. You will understand more in the youtube video.
import { Injectable } from '@angular/core';
}
import { Http, Headers } from '@angular/http';
let apiUrl = "http://localhost/PHP-Slim-Restful/api/";
@Injectable()
export class AuthService {
constructor(public http: Http) {
console.log('Hello AuthService Provider');
}
postData(credentials, type){
return new Promise((resolve, reject) =>{
let headers = new Headers();
this.http.post(apiUrl+type, JSON.stringify(credentials), {headers: headers}).
subscribe(res =>{
resolve(res.json());
}, (err) =>{
reject(err);
});
});
}
app.module.ts
Now go to src/app/app.module.ts and import authService provider and HttpModule. For more information Ionic Angular PHP Restful API User Authentication for Login and Signup.
Home.ts
You have to import authServiceProvider for comunicating with APIs.
import { Component } from "@angular/core";
import { AuthService } from "../../providers/auth-service/auth-service";
import { NavController, AlertController } from "ionic-angular";
import { Camera, CameraOptions } from "@ionic-native/camera";
takePhoto
Included some more options for Camera. In video I haven't specified the targetWidth and tagetHeight options, this will helps you to reduce the image b64 code.
takePhoto() {
}
const options: CameraOptions = {
quality: 50,
destinationType: this.camera.DestinationType.DATA_URL,
encodingType: this.camera.EncodingType.JPEG,
mediaType: this.camera.MediaType.PICTURE,
targetWidth: 600,
targetHeight: 600,
saveToPhotoAlbum: false
};
this.camera.getPicture(options).then(
imageData => {
this.base64Image = "data:image/jpeg;base64," + imageData;
this.photos.push(this.base64Image);
this.photos.reverse();
this.sendData(imageData);
},
err => {
console.log(err);
}
);
sendImageData Function
Sending b64 code to RESTful API.
sendData(imageData) {
}
this.userData.imageB64 = imageData;
this.userData.user_id = "1";
this.userData.token = "222";
console.log(this.userData);
this.authService.postData(this.userData, "userImage").then(
result => {
this.responseData = result;
},
err => {
// Error log
}
);
Download PHP Restul Project
$git clone https://github.com/srinivastamada/PHP-Slim-Restful.git
userImage
Getting images based on the user_id foreign key. You will find the in about Git repository.
$app->post('/userImage','userImage'); /* User Details */
}
function userImage(){
$request = \Slim\Slim::getInstance()->request();
$data = json_decode($request->getBody());
$user_id=$data->user_id;
$token=$data->token;
$imageB64=$data->imageB64;
$systemToken=apiToken($user_id);
try {
// if($systemToken == $token){
if(1){
if(1){
$db = getDB();
$sql = "INSERT INTO imagesData(b64,user_id_fk) VALUES(:b64,:user_id)";
$stmt = $db->prepare($sql);
$stmt->bindParam("user_id", $user_id, PDO::PARAM_INT);
$stmt->bindParam("b64", $imageB64, PDO::PARAM_STR);
$stmt->execute();
$db = null;
echo '{"success":{"status":"uploaded"}}';
} else{
echo '{"error":{"text":"No access"}}';
}
} catch(PDOException $e) {
echo '{"error":{"text":'. $e->getMessage() .'}}';
}
getImages
Getting all the images.
$app->post('/getImages', 'getImages');
}
function getImages(){
$request = \Slim\Slim::getInstance()->request();
$data = json_decode($request->getBody());
$user_id=$data->user_id;
$token=$data->token;
$systemToken=apiToken($user_id);
try {
// if($systemToken == $token){
// if($systemToken == $token){
if(1){
$db = getDB();
$sql = "SELECT b64 FROM imagesData";
$stmt = $db->prepare($sql);
$stmt->execute();
$imageData = $stmt->fetchAll(PDO::FETCH_OBJ);
$db = null;
echo '{"imageData": ' . json_encode($imageData) . '}';
} else{
echo '{"error":{"text":"No access"}}';
}
} catch(PDOException $e) {
echo '{"error":{"text":'. $e->getMessage() .'}}';
}
Display Images
Just modifed the about page for getting all the user uploaded images.
about.ts
Here getImage functions is communicating with getImages Restful and assigened to this.images
import { Component } from '@angular/core';
import { NavController } from 'ionic-angular';
import { AuthService } from "../../providers/auth-service/auth-service";
@Component({
selector: 'page-about',
templateUrl: 'about.html'
})
export class AboutPage {
public response: any;
public images: any;
userData = { user_id: "", token: "" };
constructor(public navCtrl: NavController, public authService: AuthService) {
this.getImages();
}
getImages(){
this.userData.user_id = "1";
this.userData.token = "222";
this.authService.postData(this.userData, "getImages").then(
result => {
this.response = result;
this.images = this.response.imageData
console.log(this.images.imageData);
},
err => {
console.log("error");
}
);
}
}
about.html
User ngFor and displaying all the images.
<ion-header><ion-navbar><ion-title>
About
</ion-title></ion-navbar></ion-header>
<ion-content padding>
<ion-grid>
<ion-row>
<ion-col col-6 *ngFor="let image of images;">
<ion-card class="block">
<ion-icon name="trash" class="deleteIcon" ></ion-icon>
<img src="data:image/png;base64,{{image.b64}}" *ngIf="image.b64" />
</ion-card>
</ion-col>
</ion-row>
</ion-grid>
</ion-content>
Build iOS App
Following commands for executing Xcode build, watch the video tutorial you will understand more.
$ cordova add platform ios
$ ionic cordova build ios
$ ionic cordova build ios
Build Android App
Open Android build using Android SDK>
$ cordova add platform android
$ ionic cordova build android
$ ionic cordova build android
Video Tutorial - Ionic 2 Using the Native Camera, Take Multiple Photos with Delete Action
Hello Srinivas, there must be a folder to where the images go except from the data inserted on the database? Because i upload the image, the values go to the database but when i hit refresh the images do not appear
ReplyDeleteThank you sir, your tutorial is really helpful for my project...
ReplyDeleteThanks for sharing it.
ReplyDeleteThank you very moch for the tutorial ! Can it work with firebase ?
ReplyDeleteNo, this is a native feature. Try with xcode
DeleteThanks alot for this valuable tutorial.
ReplyDeleteKindly Help me How I can upload the image to the serve in the directories.
Not in the database. Because When I uploaded the image to database. The database becomes to much heavy.
Kindly guide me.
Thanks
ReplyDeleteThanks for this artical
ReplyDeletethank you for such a useful article
ReplyDelete