Ionic is the most popular framework for Angular mobile application development. Now Ionic announced with React beta and Vue Js. This post is about getting started with Ionic React with Capacitor(Ionic Product). The Capacitor is a native bride for Cross-Platform for building universal applications. This blog post will explain how to set up a react project using Ionic mobile components and generating iOS, Android and Desktop(Electron framework build) applications.
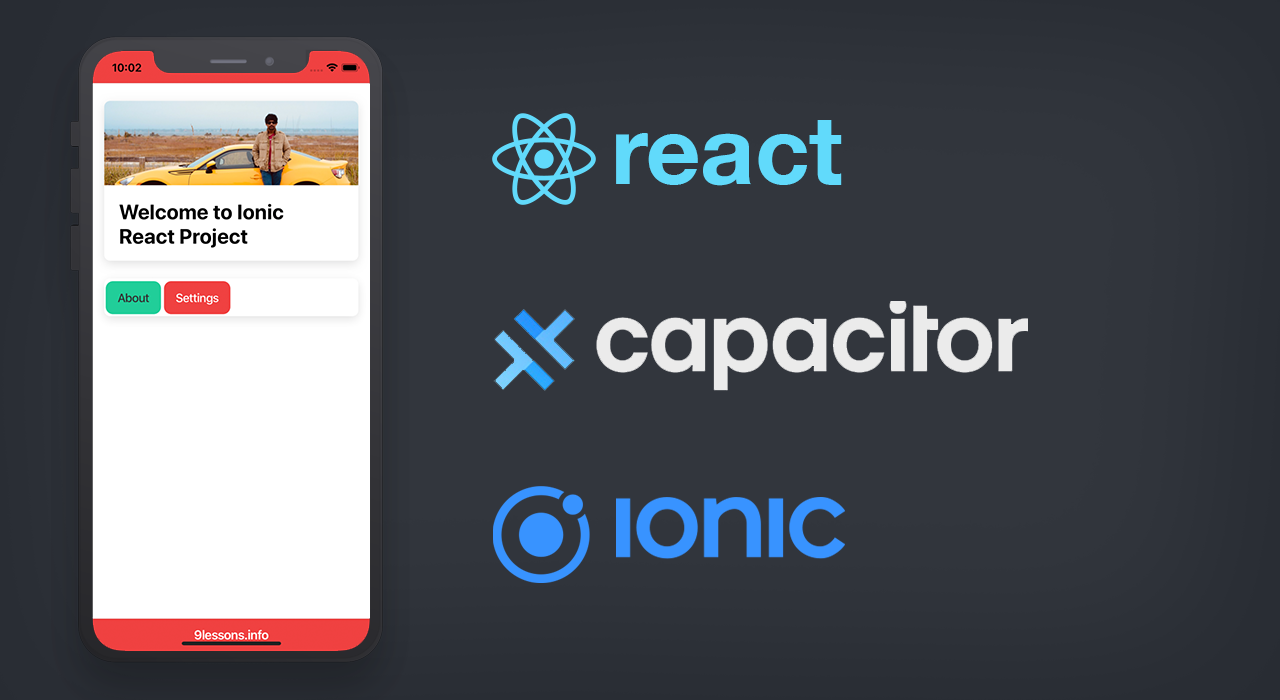
Live Demo
GitHub Checkout
Checkout the GIT branch and switch the branch to old for upcoming steps.
$git clone https://github.com/srinivastamada/react-ionic-app
$cd react-ionic-app
$git checkout chapter-1
$npm install
$npm run start
$cd react-ionic-app
$git checkout chapter-1
$npm install
$npm run start
Video Tutorial
Required Softwares
You need following softwares to start the project.
- Node.js latest version.
- NPM
- NPX(node package executer)
- Yarn
Create an Ionic React Project
Execute this command to create a react based ionic project.
$npx create-react-app react-ionic-app --typescript
$cd react-ionic-app
$cd react-ionic-app
Install Ionic and React route dependancies.
$npm install @ionic/react
$npm install react-router
$npm install react-router-dom
$npm install @types/react-router
$npm install @types/react-router-dom
$npm install react-router
$npm install react-router-dom
$npm install @types/react-router
$npm install @types/react-router-dom
App.js
Now import Ionic component packages with CSS. Clear of existing App.css code.
import '@ionic/core/css/core.css';
export default App;
import '@ionic/core/css/ionic.bundle.css';
import { IonApp } from '@ionic/react';
import React, { Component } from 'react';
class App extends Component {
render() {
return (
<IonApp></IonApp>
);
}
}
Running the Project
Use following command to execute the project. I recommend use yarn, this works great with React. Project runs at port 3000.
npm run start
or
yarn start
Compiled successfully!
You can now view react-ionic-app in the browser.
Local: http://localhost:3000/
On Your Network: http://192.168.0.17:3000/
or
yarn start
Compiled successfully!
You can now view react-ionic-app in the browser.
Local: http://localhost:3000/
On Your Network: http://192.168.0.17:3000/
Structure Your Project
Create pages, components and services folders under the project src directory.
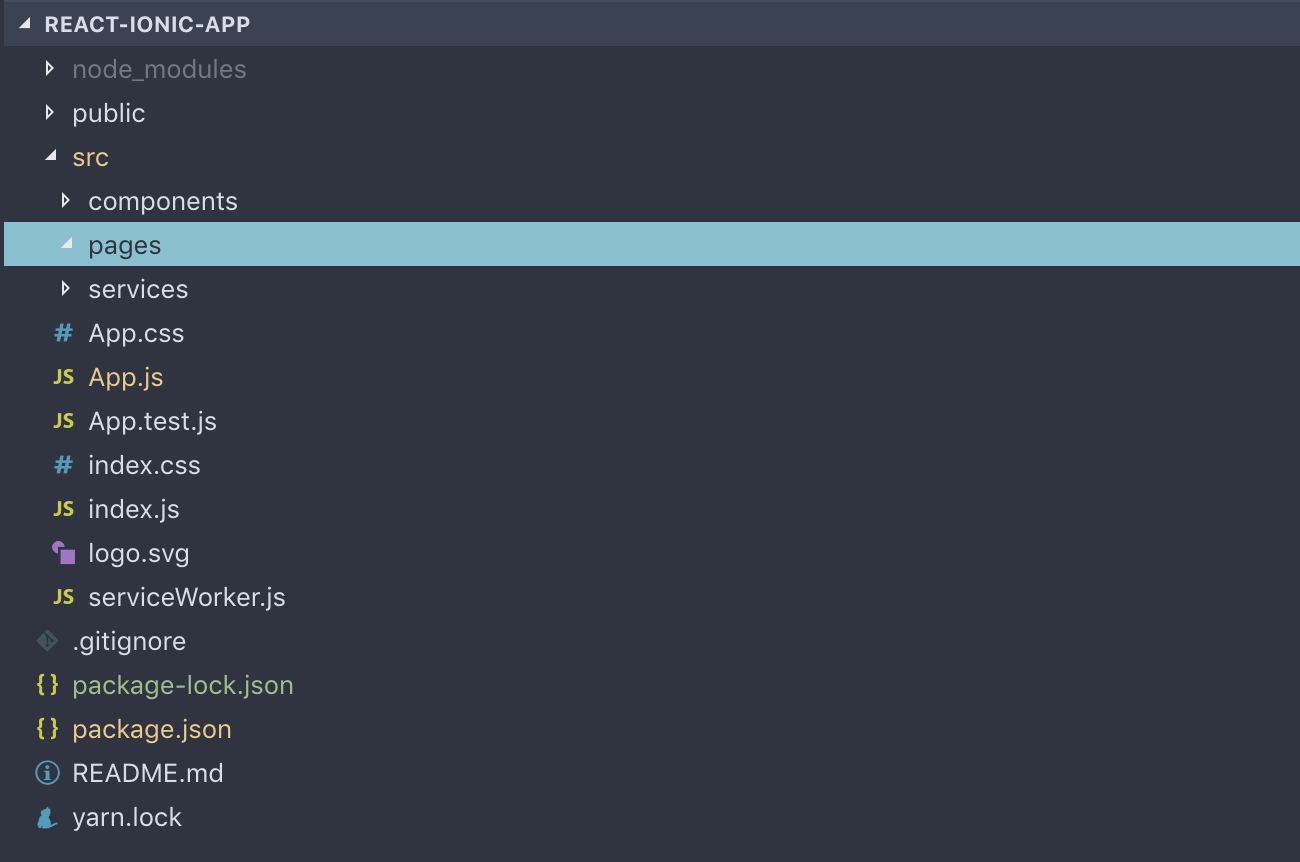
Generate React Components
React is not offering any command line to auto generate components. The generact is the third-party plugin, that will help you to generate files very quickly.
Install Generact
npm install -g generact
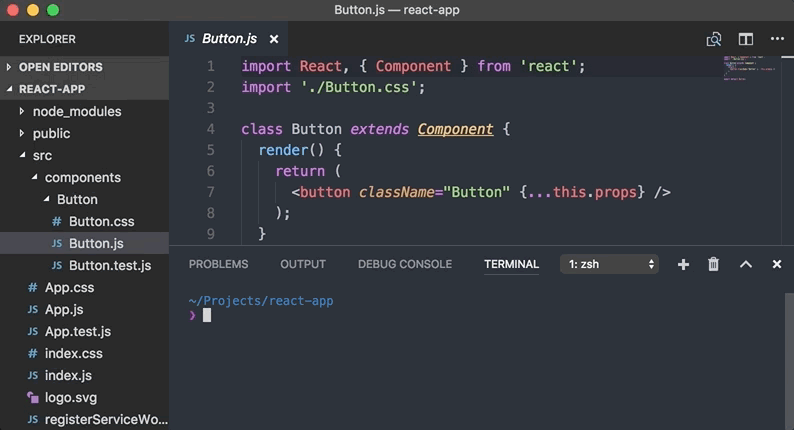
Generate React Components using generact
Go to project level and execute generact command. This will prompt you which components need to replicate.
react-ionic-app$ generact
? Which component do you want to replicate? App
? How do you want to name App component? Header
? In which folder do you want to put Header component? src/components/Header
? Which component do you want to replicate? App
? How do you want to name App component? Header
? In which folder do you want to put Header component? src/components/Header
This way all the component file generate automatically.
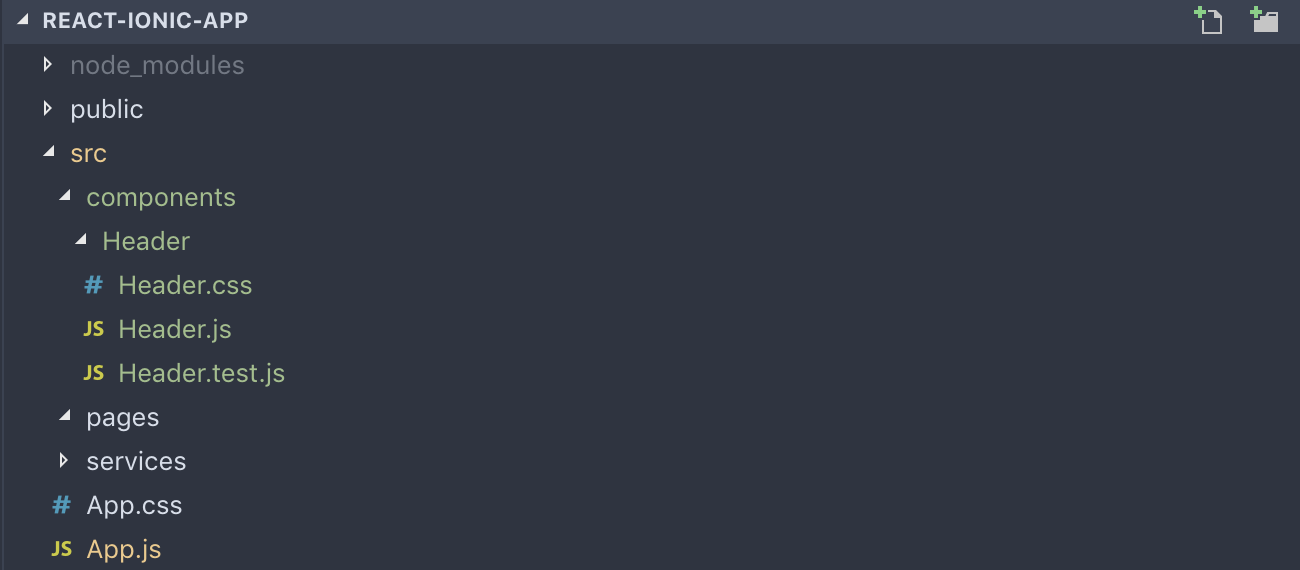
Header.js
Create Header component under components folder. Drop CSS imports, because we already imported on App.js
import {
IonHeader,
IonTitle,
IonToolbar
} from '@ionic/react';
import React, { Component } from 'react';
class Header extends Component {
render() {
return (
<IonHeader>
<IonToolbar color="danger">
<IonTitle>My Navigation Bar</IonTitle>
</IonToolbar>
</IonHeader>
);
}
}
Replicated Footer component based on Header
react-ionic-app$ generact
? Which component do you want to replicate? Header
? How do you want to name Header component? Footer
? In which folder do you want to put Footer component? src/components
? Which component do you want to replicate? Header
? How do you want to name Header component? Footer
? In which folder do you want to put Footer component? src/components
Footer.js
Import IonFooter component for the design.
import { IonFooter, IonTitle, IonToolbar } from '@ionic/react';
export default Footer;
import React, { Component } from 'react';
class Footer extends Component {
render() {
return (
<IonFooter>
<IonToolbar color="primary">
<IonTitle>Footer</IonTitle>
</IonToolbar>
</IonFooter>
);
}
}
Generate Home Page
Using generact replicate the Header components for Home page.
react-ionic-app$ generact
? Which component do you want to replicate? Header
? How do you want to name Header component? Home
? In which folder do you want to put Home component? src/pages
? Which component do you want to replicate? Header
? How do you want to name Header component? Home
? In which folder do you want to put Home component? src/pages
Home.js
This contains the page content.
import {
IonCard,
IonCardHeader,
IonCardTitle,
IonContent
} from '@ionic/react';
import React, { Component } from 'react';
class Home extends Component {
render() {
return (
<IonContent>
<IonCard>
<IonCardHeader>
<IonCardTitle>Welcome to Home Page</IonCardTitle>
</IonCardHeader>
</IonCard>
</IonContent>
);
}
}
export default Home;
Follow the same for other pages.
react-ionic-app$ generact
? Which component do you want to replicate? Home
? How do you want to name Home component? Settings
? In which folder do you want to put Settings component? src/pages
react-ionic-app$ generact
? Which component do you want to replicate? Home
? How do you want to name Home component? About
? In which folder do you want to put About component? src/pages
? Which component do you want to replicate? Home
? How do you want to name Home component? Settings
? In which folder do you want to put Settings component? src/pages
react-ionic-app$ generact
? Which component do you want to replicate? Home
? How do you want to name Home component? About
? In which folder do you want to put About component? src/pages
You will find the generated components in following structure.
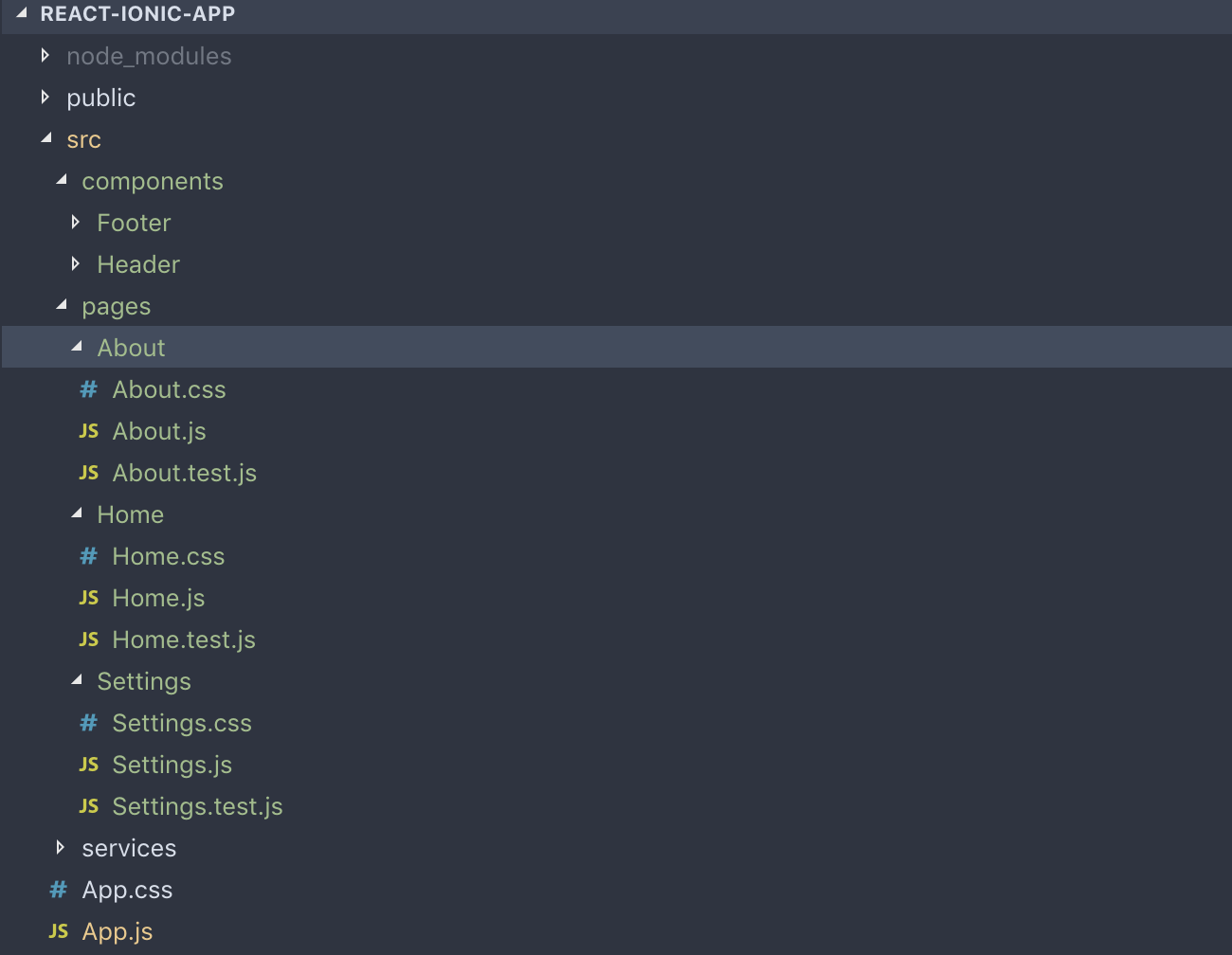
Construct with Ionic Components
App.js
Now import all of the components unser IonApp.
import '@ionic/core/css/core.css';
export default App;
import '@ionic/core/css/ionic.bundle.css';
import { IonApp } from '@ionic/react';
import React, { Component } from 'react';
import Footer from './components/Footer/Footer';
import Header from './components/Header/Header';
import Home from './pages/Home/Home';
class App extends Component {
render() {
return (
<IonApp>
<Header />
<Home />
<Footer />
</IonApp>
);
}
}
You will find the output with Home component.
Working with Routes
Now we need to connect all the pages using Routes.
Create a routes.js configuration file under the project src directory.
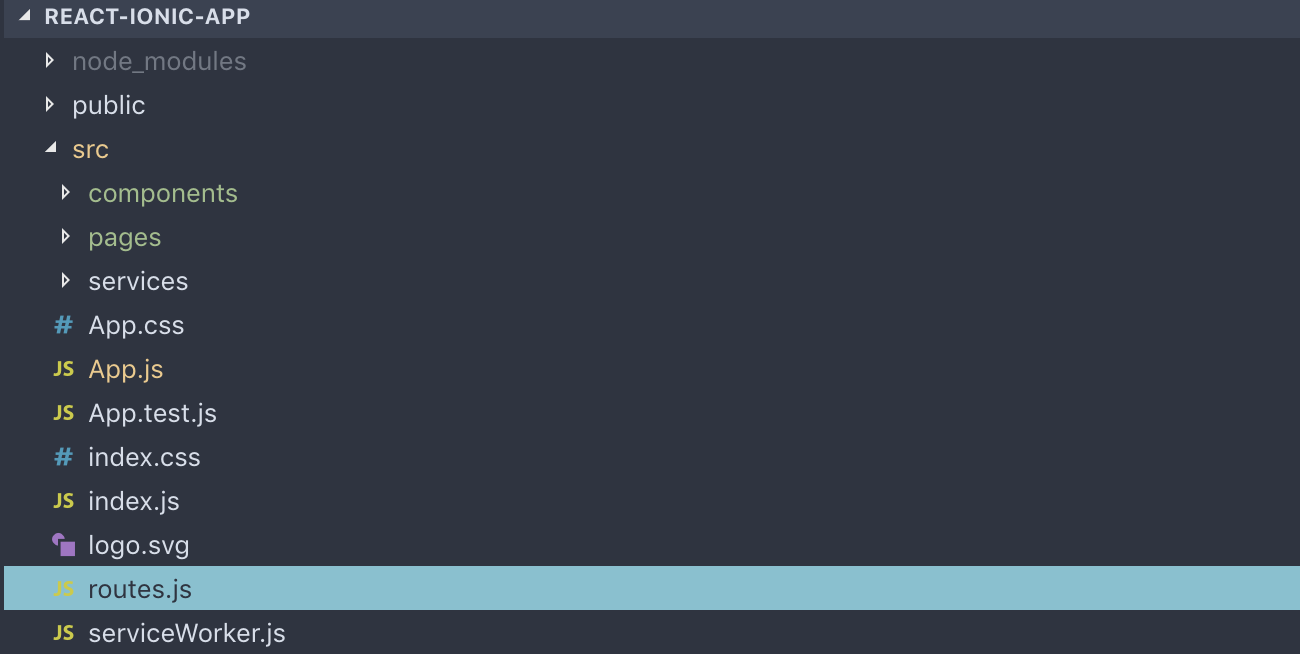
routes.js
Connect the components with React routes. Here path * refers to 404 page.
import React from 'react';
export default Routes;
import { BrowserRouter, Switch, Route } from 'react-router-dom';
import Home from './pages/Home/Home';
import About from './pages/About/About';
import Settings from './pages/Settings/Settings';
import NoPage from './pages/NoPage/NoPage';
import NoPage from './pages/NoPage/NoPage';
const Routes = () => (
<BrowserRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route exact path="/about" component={About} />
<Route exact path="/settings" component={Settings} />
<Route exact path="/settings" component={Settings} />
<Route exact path="*" component={NoPage} />
</Switch>
</BrowserRouter>
);
App.js
Now include Routes in main App page. The default route load the Home page.
import '@ionic/core/css/core.css';
import '@ionic/core/css/ionic.bundle.css';
import { IonApp } from '@ionic/react';
import React, { Component } from 'react';
import Footer from './components/Footer/Footer';
import Header from './components/Header/Header';
import Routes from './routes';
class App extends Component {
render() {
return (
<IonApp>
<Header />
<Routes />
<Footer />
</IonApp>
);
}
}
export default App;
Home.js
For navigation to other pages use Link component to redirect.
import {
IonCard,
IonCardHeader,
IonCardTitle,
IonContent
} from '@ionic/react';
import React, { Component } from 'react';
import { Link } from 'react-router-dom';
class Home extends Component {
render() {
return (
<IonContent>
<IonCard>
<IonCardHeader>
<IonCardTitle>Welcome to Home Page</IonCardTitle>
<Link to="/about">About</Link>
<Link to="/settings">Settings</Link>
</IonCardHeader>
</IonCard>
</IonContent>
);
}
}
export default Home;
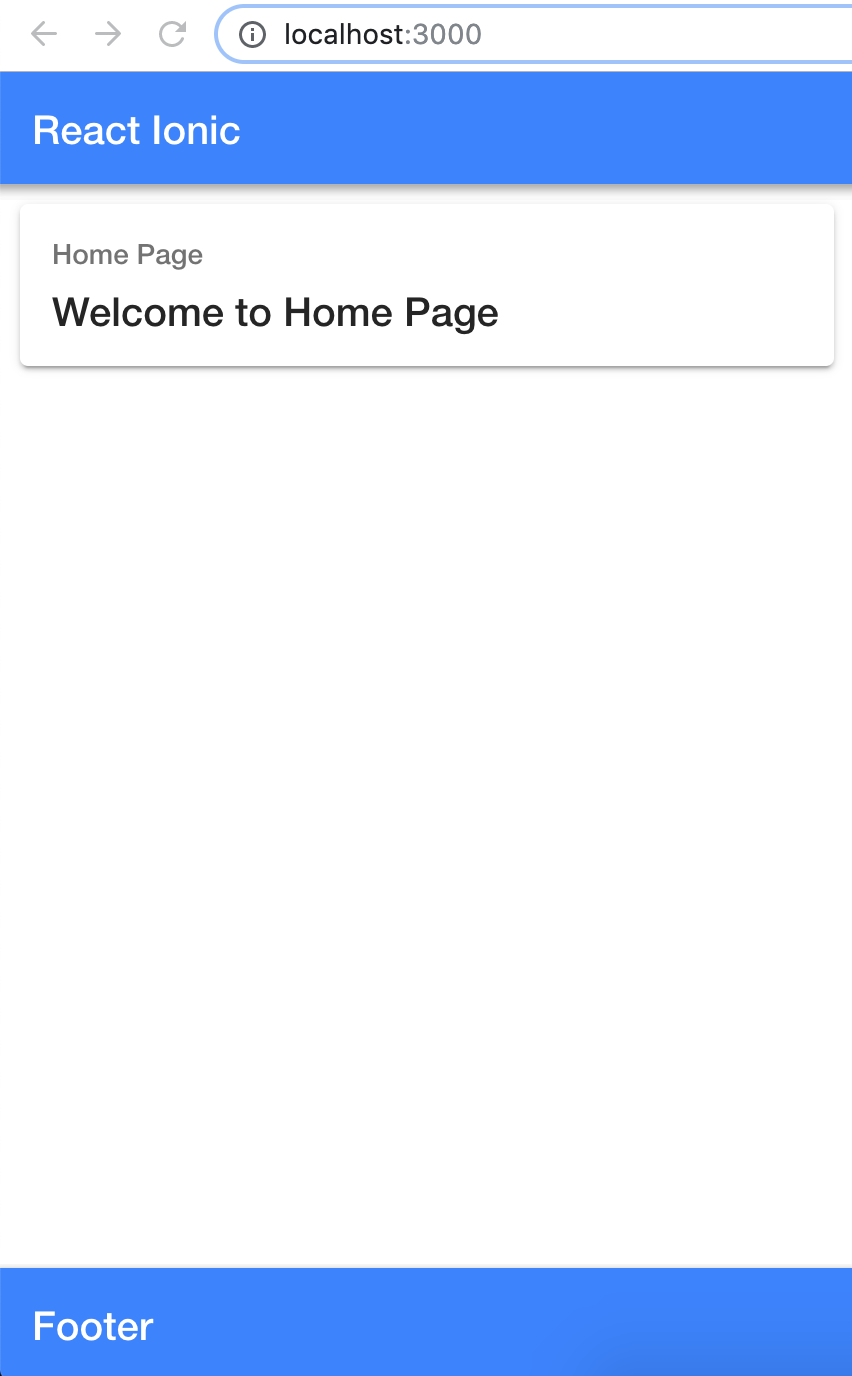
Working with Capacitor - Build Mobile Application
You need following plugins to covert the web project to a mobile application.
npm install --save @capacitor/core @capacitor/cli
Build the Web Project
You have to build the web project for creating the mobile applications.
npm run build
or
yarn build
or
yarn build
Initialize the Capacitor
The command npx cap init creates the configuration file. Modify webDir to build, instead of www
react-ionic-app$ npx cap init
? App name YourAppName
? App Package ID (in Java package format, no dashes) com.reactionic.com
? Which npm client would you like to use? yarn
✔ Initializing Capacitor project in /react-ionic-app in 2.57ms
🎉 Your Capacitor project is ready to go! 🎉
Add platforms using "npx cap add":
npx cap add android
npx cap add ios
npx cap add electron
Follow the Developer Workflow guide to get building:
https://capacitor.ionicframework.com/docs/basics/workflow
? App name YourAppName
? App Package ID (in Java package format, no dashes) com.reactionic.com
? Which npm client would you like to use? yarn
✔ Initializing Capacitor project in /react-ionic-app in 2.57ms
🎉 Your Capacitor project is ready to go! 🎉
Add platforms using "npx cap add":
npx cap add android
npx cap add ios
npx cap add electron
Follow the Developer Workflow guide to get building:
https://capacitor.ionicframework.com/docs/basics/workflow
iOS Project
The following command generates the iOS support file for Xcode.
react-ionic-app$ npx cap add ios
✔ Installing iOS dependencies in 20.32s
✔ Adding native xcode project in: /react-ionic-app/ios in 37.37ms
✔ add in 20.36s
✔ Copying web assets from build to ios/App/public in 712.87ms
✔ Copying native bridge in 4.76ms
✔ Copying capacitor.config.json in 2.28ms
✔ copy in 731.23ms
✔ Updating iOS plugins in 2.25ms
Found 0 Capacitor plugins for ios:
✖ Updating iOS native dependencies:
✖ update ios:
[error] Error running update: [!] Unknown installation options: disable_input_output_paths.
✔ Installing iOS dependencies in 20.32s
✔ Adding native xcode project in: /react-ionic-app/ios in 37.37ms
✔ add in 20.36s
✔ Copying web assets from build to ios/App/public in 712.87ms
✔ Copying native bridge in 4.76ms
✔ Copying capacitor.config.json in 2.28ms
✔ copy in 731.23ms
✔ Updating iOS plugins in 2.25ms
Found 0 Capacitor plugins for ios:
✖ Updating iOS native dependencies:
✖ update ios:
[error] Error running update: [!] Unknown installation options: disable_input_output_paths.
Update Cocoapods
If you are getting "Updating iOS native dependencies" issue.
$pod repo update
CocoaPods 1.7.0.rc.1 is available.
To update use: `sudo gem install cocoapods --pre`
[!] This is a test version we'd love you to try.
sudo gem install cocoapods --pre
react-ionic-app$ npx cap update ios
✔ Updating iOS plugins in 12.39ms
Found 0 Capacitor plugins for ios:
✔ Updating iOS native dependencies in 9.81s
✔ update ios in 9.84s
Update finished in 9.871s
CocoaPods 1.7.0.rc.1 is available.
To update use: `sudo gem install cocoapods --pre`
[!] This is a test version we'd love you to try.
sudo gem install cocoapods --pre
react-ionic-app$ npx cap update ios
✔ Updating iOS plugins in 12.39ms
Found 0 Capacitor plugins for ios:
✔ Updating iOS native dependencies in 9.81s
✔ update ios in 9.84s
Update finished in 9.871s
Android Project
The following command generates the Android SDK files.
react-ionic-app$ npx cap add android
✔ Installing android dependencies in 28.66s
✔ Adding native android project in: /react-ionic-app/android in 75.12ms
✔ Syncing Gradle in 51.01s
✔ add in 79.75s
✔ Copying web assets from build to android/app/src/main/assets/public in 677.46ms
✔ Copying native bridge in 2.39ms
✔ Copying capacitor.config.json in 1.53ms
✔ copy in 701.45ms
✔ Updating Android plugins in 2.77ms
Found 0 Capacitor plugins for android:
✔ update android in 27.06ms
✔ Installing android dependencies in 28.66s
✔ Adding native android project in: /react-ionic-app/android in 75.12ms
✔ Syncing Gradle in 51.01s
✔ add in 79.75s
✔ Copying web assets from build to android/app/src/main/assets/public in 677.46ms
✔ Copying native bridge in 2.39ms
✔ Copying capacitor.config.json in 1.53ms
✔ copy in 701.45ms
✔ Updating Android plugins in 2.77ms
Found 0 Capacitor plugins for android:
✔ update android in 27.06ms
Elector Desktop Project
This generates the desktop SDK files. For more information check Ionic Electron Desktop App
react-ionic-app$ npx cap add electron
✔ Adding Electron project in: /react-ionic-app/electron in 17.66ms
⠋ Installing NPM DependenciesInstalling NPM Dependencies...
⠴ addyarn install v1.16.0
info No lockfile found.
[1/4] Resolving packages...
[2/4] Fetching packages...
[3/4] Linking dependencies...
[4/4] Building fresh packages...
success Saved lockfile.
Done in 36.18s.
warning electron > electron-download > nugget > progress-stream > through2 > xtend > [email protected]:
NPM Dependencies installed!
✔ Installing NPM Dependencies in 36.58s
✔ add in 36.60s
⠋ Copying web assets from build to electron/appCleaning /Users/SrinivasTamada/Desktop/projects/react-ionic-app/electron/app...
Copying web assets...
✔ Copying web assets from build to electron/app in 1.07s
✔ Copying capacitor.config.json in 2.23ms
✔ copy in 1.08s
✔ update electron in 682.89μp
✔ Adding Electron project in: /react-ionic-app/electron in 17.66ms
⠋ Installing NPM DependenciesInstalling NPM Dependencies...
⠴ addyarn install v1.16.0
info No lockfile found.
[1/4] Resolving packages...
[2/4] Fetching packages...
[3/4] Linking dependencies...
[4/4] Building fresh packages...
success Saved lockfile.
Done in 36.18s.
warning electron > electron-download > nugget > progress-stream > through2 > xtend > [email protected]:
NPM Dependencies installed!
✔ Installing NPM Dependencies in 36.58s
✔ add in 36.60s
⠋ Copying web assets from build to electron/appCleaning /Users/SrinivasTamada/Desktop/projects/react-ionic-app/electron/app...
Copying web assets...
✔ Copying web assets from build to electron/app in 1.07s
✔ Copying capacitor.config.json in 2.23ms
✔ copy in 1.08s
✔ update electron in 682.89μp
Update Mobile Packages
Use the following sync command for updating the mobile packages with latest web build update.
react-ionic-app$ npx cap sync
✔ Copying web assets from build to android/app/src/main/assets/public in 609.50ms
✔ Copying native bridge in 2.68ms
✔ Copying capacitor.config.json in 1.54ms
✔ copy in 634.23ms
✔ Updating Android plugins in 2.04ms
Found 0 Capacitor plugins for android:
✔ update android in 19.84ms
✔ Copying web assets from build to ios/App/public in 491.27ms
✔ Copying native bridge in 1.29ms
✔ Copying capacitor.config.json in 2.72ms
✔ copy in 502.19ms
✔ Updating iOS plugins in 1.94ms
Found 0 Capacitor plugins for ios:
✔ Updating iOS native dependencies in 15.03s
✔ update ios in 15.04s
⠋ Copying web assets from build to electron/appCleaning /react-ionic-app/electron/app...
⠙ Copying web assets from build to electron/appCopying web assets...
✔ Copying web assets from build to electron/app in 610.96ms
✔ Copying capacitor.config.json in 5.15ms
✔ copy in 619.52ms
✔ update electron in 19.43μp
✔ copy in 336.19μp
✔ update web in 17.34μp
Sync finished in 16.839s
✔ Copying web assets from build to android/app/src/main/assets/public in 609.50ms
✔ Copying native bridge in 2.68ms
✔ Copying capacitor.config.json in 1.54ms
✔ copy in 634.23ms
✔ Updating Android plugins in 2.04ms
Found 0 Capacitor plugins for android:
✔ update android in 19.84ms
✔ Copying web assets from build to ios/App/public in 491.27ms
✔ Copying native bridge in 1.29ms
✔ Copying capacitor.config.json in 2.72ms
✔ copy in 502.19ms
✔ Updating iOS plugins in 1.94ms
Found 0 Capacitor plugins for ios:
✔ Updating iOS native dependencies in 15.03s
✔ update ios in 15.04s
⠋ Copying web assets from build to electron/appCleaning /react-ionic-app/electron/app...
⠙ Copying web assets from build to electron/appCopying web assets...
✔ Copying web assets from build to electron/app in 610.96ms
✔ Copying capacitor.config.json in 5.15ms
✔ copy in 619.52ms
✔ update electron in 19.43μp
✔ copy in 336.19μp
✔ update web in 17.34μp
Sync finished in 16.839s
Build iOS App
Following commands for executing Xcode build, watch the video tutorial you will understand more.
$ npx cap open ios
Build Android App
Open Android build using Android SDK>
$npx cap open android
Build Electron Desktop Application
Open Electron build.
$npx cap open android
Sir thank you
ReplyDelete