As I promised to continue the Angular/Ionic project series, as a developer perspective mock server is the most important to progress the development. We should not depend on the production or development API for front-end development. This post is about creating a simple Node Express server with mock JSON object files. You can import the project to any of the front-end applications like Angular, React, Ionic and VueJS projects.
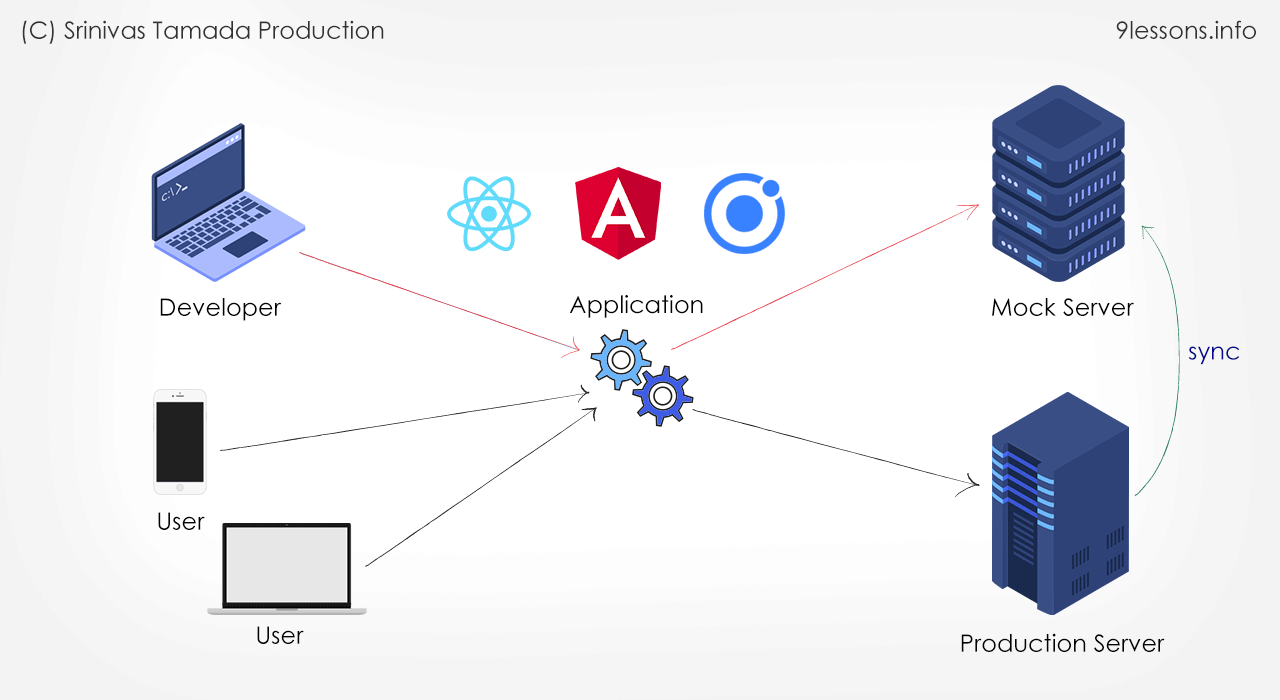
Video Tutorial
Getting Started with Mock Server
Create a folder called server and initiate node package.
$npm init
This will create a package.json file. You have to provide the following information.
See `npm help json` for definitive documentation on these fields
and exactly what they do.
Use `npm install` afterwards to install a package and
save it as a dependency in the package.json file.
Press ^C at any time to quit.
package name: (mock) mock_server
version: (1.0.0)
description: Mock Server
entry point: (index.js) server.js
test command:
git repository:
keywords:
author: Your Name
license: (ISC)
About to write to /mock/package.json:
{
"name": "mock_server",
"version": "1.0.0",
"description": "Mock Server",
"main": "server.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node server.js"
},
"author": "Srinivas Tamada",
"license": "ISC"
}
Is this OK? (yes) yes
and exactly what they do.
Use `npm install
save it as a dependency in the package.json file.
Press ^C at any time to quit.
package name: (mock) mock_server
version: (1.0.0)
description: Mock Server
entry point: (index.js) server.js
test command:
git repository:
keywords:
author: Your Name
license: (ISC)
About to write to /mock/package.json:
{
"name": "mock_server",
"version": "1.0.0",
"description": "Mock Server",
"main": "server.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node server.js"
},
"author": "Srinivas Tamada",
"license": "ISC"
}
Is this OK? (yes) yes
Install Express JS
Express is a flexible Node.js web application framework that provides an API HTTP utility methods.
$ npm install express
server.js
Create a server.js file and import express plugin for HTTP untility methods.
let express = require('express');
});
let app = express();
let bodyParser = require('body-parser');
let backendPort = 8084;
app.use(function(req, res, next) {
res.header('Access-Control-Allow-Origin', '*');
res.header('Access-Control-Allow-Credentials', true);
res.header('Access-Control-Allow-Methods', 'GET,HEAD,OPTIONS,POST,PUT');
res.header(
'Access-Control-Allow-Headers',
'Access-Control-Allow-Headers, Origin,Accept, X-Requested-With, Content-Type, Access-Control-Request-Method, Access-Control-Request-Headers'
);
next();
});
app.use(bodyParser.json());
app.use(bodyParser.text());
app.use(
bodyParser.urlencoded({
extended: true
})
);
app.listen(backendPort, function() {
console.log('Express server listening on port ' + backendPort);
File Structure
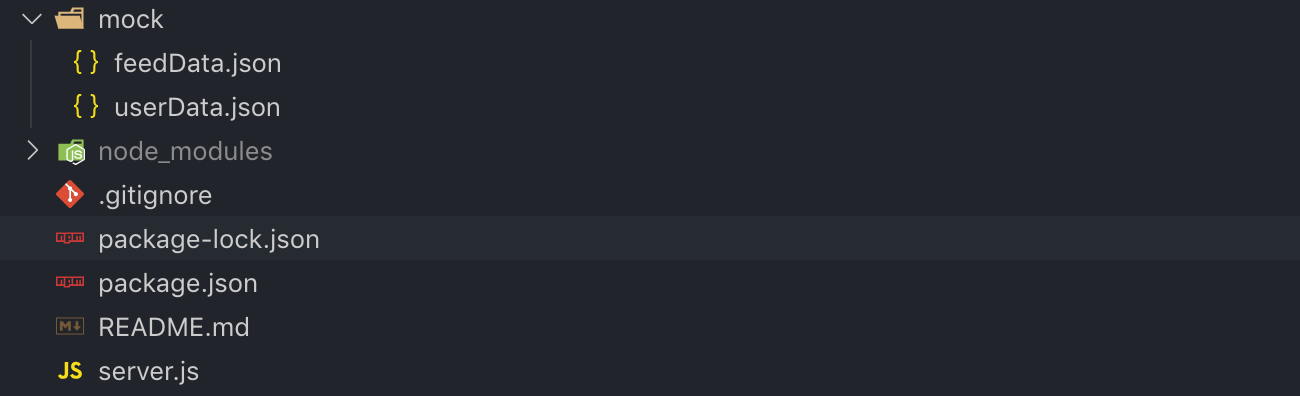
userData.json
Copy the user data response from the production API for mock testing. Here is the live API URL for login https://api.thewallscript.com/restful/login you will find this when you access Banana Ionic project.
{
}
"userData": {
"user_id": "1",
"name": "Srinivas Tamada",
"email": "[email protected]",
"username": "srinivas",
"token": "084627b29261fc50f9fd3f3ebc029da"
}
feedData.json
Follow the same and copy the feed data response.
{
}
"feedData": [
{
"feed_id": "853",
"feed": "Make people fall in love with your ideas",
"user_id_fk": "1",
"created": "1566456759"
},
{
"feed_id": "850",
"feed": "Technolgy blog www.9lessons.info",
"user_id_fk": "1",
"created": "1566283656"
},
{
"feed_id": "840",
"feed": "Node mock server",
"user_id_fk": "1",
"created": "1566137529"
}
]
Import JSON files - server.js
Import JSON file paths.
let mock = {
};
userData: require('./mock/userData'),
feedData: require('./mock/feedData'),
API login - server.js
Create a POST login API with test credentials and map the JSON response with 200 status code. If you want change the error status.
app.post('/login', function(req, res, next) {
});
let data = JSON.parse(req.body);
let username = data.username;
let password = data.password;
if (username === 'testuser' && password === 'testpass') {
return res.status(200).json(mock.userData);
} else {
return res
.status(200)
.send('{"error":{"text":"Bad request wrong username and password"}}');
}
Run the Mock Server
$node server.js
Postman Application
Download the latest Postman and test the login API.
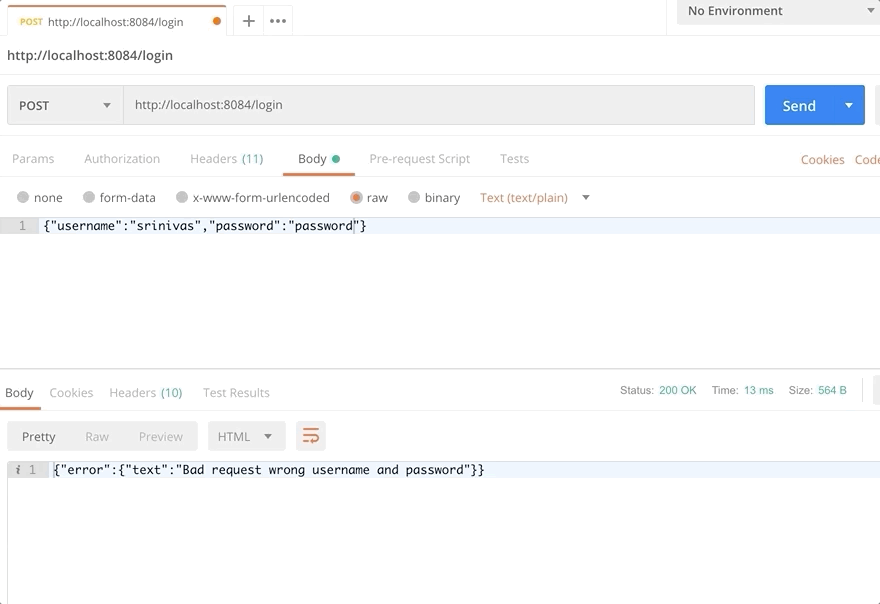
API feed - server.js
Create a POST feed API validate with user token and map the JSON response with 200 status code.
app.post('/feed', function(req, res, next) {
});
let data = JSON.parse(req.body);
if (data.token && data.user_id === '1') {
return res.status(200).json(mock.feedData);
} else {
return res.status(401).send('No Access');
}
Test the feed API.
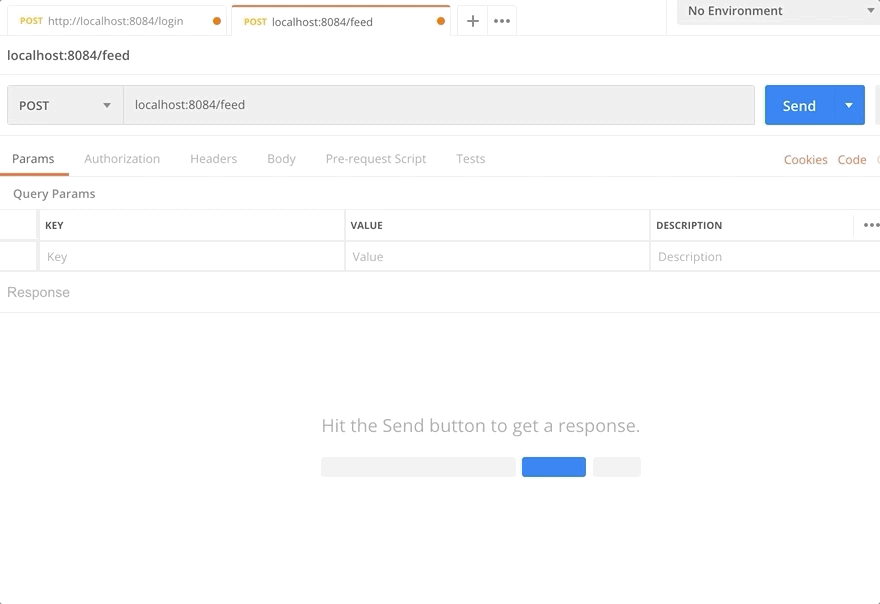
Angular or React Environment
You will find an environment folder in your Angular or React project.
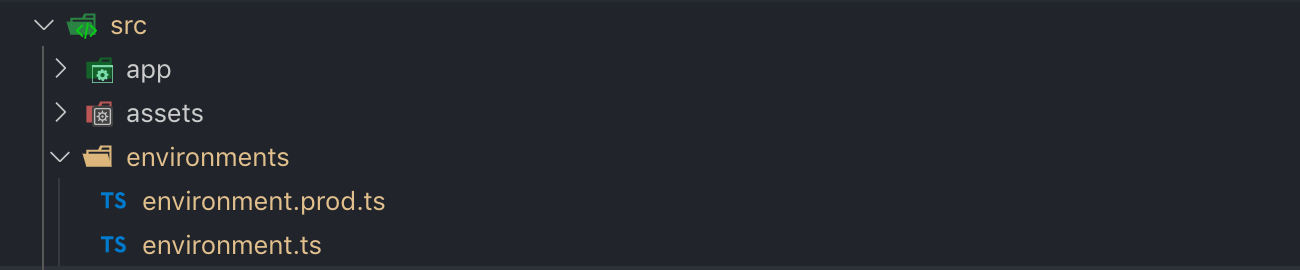
environment.ts
This is configuration for development environment. Here set your mock server API path.
export const environment = {
};
production: false,
apiUrl: 'http://localhost:8084/'
environment.prod.ts
Production configuration file. Here set you production API end point. Production build ng build --prod command automatically maps with live API.
export const environment = {
};
production: true,
apiUrl: 'https://api.thewallscript.com/restful/'
0 comments: