class New_Class extends Old_Class { ....... }
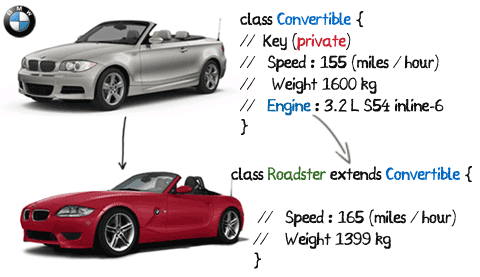
The inherited methods and data are those that are declared public or protected inside the super class. Using(Extending) same Engine.
Note: private members are not inherited. Here key is private
Java Access Modifiers Lesson
Eg How Inheritance works.
class Add
{
int x;
int y;
public int Add_xy()
{
int sum=0;
sum=x+y;
return sum;
}
}
class Sub extends Add
{
public int Sub_xy()
{
int sub;
sub=x-y;
return sub;
}
}
class Inheritance
{
pubic static void main(String args[])
{
Sub sub_obj=new Sub();
sub_obj.x=10;
sub_obj.y=4;
int a=sub_obj.Add_xy();
int s=sub_obj.Sub_xy();
System.out.println("x value is "+sub_obj.x);
System.out.println("y value is "+sub_obj.y);
System.out.println("sum is "+a);
System.out.println("subtraction is "+s);
}
}
>javac Inheritance.java
>java Inheritance
output:
x value is 10;
y value is 4;
sum is 14;
subtraction is 6;
Note : The object of the sub-class was created inside the main. The class Sub extends the class Add. This ist derives all the protected and public members of the class Add. The class Sub contains a method of it own, Sub_xy()/ The Object of class Sub in the main() is used to assign values to x and y and then the two methods are called.
Java does not support multiple inheritance directly. This means that the class in Java cannot have more than one super class.
Eg:
class Derived extends Super_one, Super_two
{
-----------------
-----------------
}
is illegal/not permitted in Java.
However, to be able to let the java programmers use the immense functionality provided by multiple inheritance, the java language developers incorporated this concept with the use of interfaces. (I will post an article about Interfaces with in few days)
A wrong example for inheritance
ReplyDeleteAn important aspect which is missing, is the relationship between the super-class and the derived sub-class.
Inheritance should be used when we see an "is a" relationship.
The example of Sub extends Add would be incorrect since
we can't say that Sub is a Add.
Always when using inheritance check whether you have the "is a" relationship.
Do not inherit for the sake of inheriting methods from another class.
The two classes should have a natural "is a" relation.
Very interesting.. this is Great Coding Technique.. Very interesting.. Post Awesome post.. Thanks
ReplyDelete