Facebook wall script with expanding URLs one of the popular post on 9lessons. It is just collaboration of my previous jquery articles. Recent days I received lots of requests from my readers that asked to me how to display old updates. So that I have decided to release new version with user control support. This script very easy to install few lines of code changes, take a quick look at following steps.
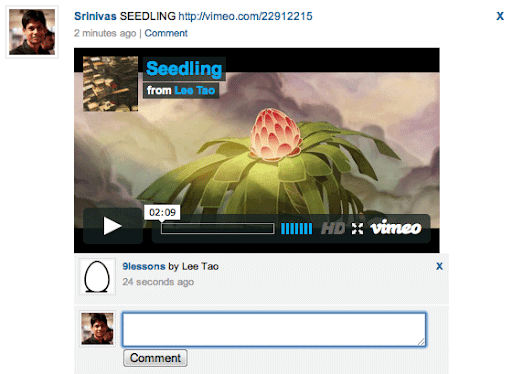


Social Network System with React JS Part One
Angular JS Facebook Wall System with Multiple Bindings Tutorial
The download script contains three folders called includes,css and js with PHP files.
includes
-- functions.php
-- db.php //Database connection
js
-- wall.js
-- jquery.oembed.js
-- jquery.min.js // 1.4.2 version
css
-- wall.css
//Files
index.php, load_messages.php, load_comments.php, message_ajax.php,comment_ajax.php, delete_message_ajax.php, delete_comment_ajax.php
-- functions.php
-- db.php //Database connection
js
-- wall.js
-- jquery.oembed.js
-- jquery.min.js // 1.4.2 version
css
-- wall.css
//Files
index.php, load_messages.php, load_comments.php, message_ajax.php,comment_ajax.php, delete_message_ajax.php, delete_comment_ajax.php

Sample database design for Facebook wall script. Contains there tables users, messages and comments.
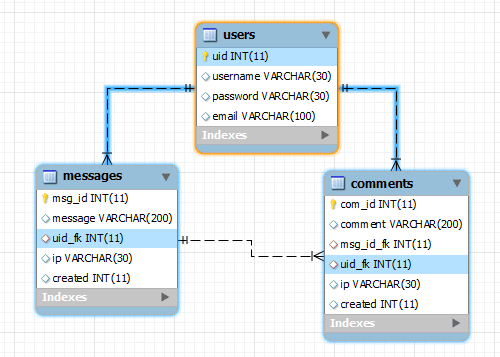
Step 1
You have to create following tables otherwise find out wall.sql and execute SQL queries. Users
Contains user management details username, password, email and etc.
CREATE TABLE `users` (
`uid` int(11) AUTO_INCREMENT PRIMARY KEY,
`username` varchar(255) UNIQUE KEY,
`password` varchar(100),
`email` varchar(255) UNIQUE KEY
)
`uid` int(11) AUTO_INCREMENT PRIMARY KEY,
`username` varchar(255) UNIQUE KEY,
`password` varchar(100),
`email` varchar(255) UNIQUE KEY
)
Messages
Contains messages/updates details messages, created, user and etc.
CREATE TABLE `messages` (
`msg_id` int(11) AUTO_INCREMENT PRIMARY KEY,
`message` varchar(255)
`uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(uid_fk) REFERENCES users(uid)
)
`msg_id` int(11) AUTO_INCREMENT PRIMARY KEY,
`message` varchar(255)
`uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(uid_fk) REFERENCES users(uid)
)
Comments
Contains user management details username, password, email and etc.
CREATE TABLE `comments` (
`com_id` int(11) AUTO_INCREMENT PRIMARY KEY,
`comment` varchar(255)
`msg_id_fk` int(11),
`uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(uid_fk) REFERENCES users(uid),
FOREIGN KEY(msg_id_fk) REFERENCES messages(msg_id)
)
`com_id` int(11) AUTO_INCREMENT PRIMARY KEY,
`comment` varchar(255)
`msg_id_fk` int(11),
`uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(uid_fk) REFERENCES users(uid),
FOREIGN KEY(msg_id_fk) REFERENCES messages(msg_id)
)
Step 2
MySQL database configuration settings.db.php
You have to modify Host, Username and Password.
<?php
define('DB_SERVER', 'Host');
define('DB_USERNAME', 'User Name');
define('DB_PASSWORD', 'Password');
define('DB_DATABASE', 'Database');
$connection = mysql_connect(DB_SERVER, DB_USERNAME, DB_PASSWORD) or die(mysql_error());
$database = mysql_select_db(DB_DATABASE) or die(mysql_error());
?>
define('DB_SERVER', 'Host');
define('DB_USERNAME', 'User Name');
define('DB_PASSWORD', 'Password');
define('DB_DATABASE', 'Database');
$connection = mysql_connect(DB_SERVER, DB_USERNAME, DB_PASSWORD) or die(mysql_error());
$database = mysql_select_db(DB_DATABASE) or die(mysql_error());
?>
Step 3
Root file it includes javascript and css files such as Jquery 1.4.2, jquery.oembed.js, wall.js and wall.css. Note: Expanding URL oembed plugin works with Jquery 1.4.2 problem with Jquery higher versions.index.php
Contains PHP and HTML code. Included db.php, functions.php, sessions.php and load_messages.php.
<?php
include_once 'includes/db.php';
include_once 'includes/functions.php';
include_once 'session.php';
$Wall = new Wall_Updates();
$updatesarray=$Wall->Updates($uid);
?>
<link href="css/wall.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.oembed.js"></script>
<script type="text/javascript" src="js/wall.js"></script>
What's up?
<form method="post" action="">
<textarea cols="30" rows="4" name="update" id="update" maxlength="200" ></textarea>
<br />
<input type="submit" value=" Update " id="update_button" class="update_button"/>
</form>
<div id="content">
<?php include('load_messages.php'); ?>
</div>
include_once 'includes/db.php';
include_once 'includes/functions.php';
include_once 'session.php';
$Wall = new Wall_Updates();
$updatesarray=$Wall->Updates($uid);
?>
<link href="css/wall.css" rel="stylesheet" type="text/css">
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.oembed.js"></script>
<script type="text/javascript" src="js/wall.js"></script>
What's up?
<form method="post" action="">
<textarea cols="30" rows="4" name="update" id="update" maxlength="200" ></textarea>
<br />
<input type="submit" value=" Update " id="update_button" class="update_button"/>
</form>
<div id="content">
<?php include('load_messages.php'); ?>
</div>
Step 4
Session configuration.session.php
User authentication success login.php should generate user id value as session.
<?php
session_start();
$uid=$_SESSION['user_id']; // In demo $uid=1
?>
session_start();
$uid=$_SESSION['user_id']; // In demo $uid=1
?>
Step 5
Final step if you want to add new functionalities just modify functions.phpCSS design: Status Message Design with CSS
functions.php
Contains PHP code.
<?php
class Wall_Updates
{
// Updates
public function Updates($uid)
{
$query = mysql_query("SELECT M.msg_id, M.uid_fk, M.message, M.created, U.username FROM messages M, users U WHERE M.uid_fk=U.uid and M.uid_fk='$uid' order by M.msg_id desc ") or die(mysql_error());
while($row=mysql_fetch_array($query))
$data[]=$row;
return $data;
}
//Comments
public function Comments($msg_id)
{
$query = mysql_query("SELECT C.com_id, C.uid_fk, C.comment, C.created, U.username FROM comments C, users U WHERE C.uid_fk=U.uid and C.msg_id_fk='$msg_id' order by C.com_id asc ") or die(mysql_error());
while($row=mysql_fetch_array($query))
$data[]=$row;
if(!empty($data))
{
return $data;
}
}
//Avatar Image
public function Gravatar($uid)
{
$query = mysql_query("SELECT email FROM `users` WHERE uid='$uid'") or die(mysql_error());
$row=mysql_fetch_array($query);
if(!empty($row))
{
$email=$row['email'];
$lowercase = strtolower($email);
$imagecode = md5( $lowercase );
$data="http://www.gravatar.com/avatar.php?gravatar_id=$imagecode";
return $data;
}
else
{
$data="default.jpg";
return $data;
}
}
//Insert Update
public function Insert_Update($uid, $update)
{
$update=htmlentities($update);
$time=time();
$ip=$_SERVER['REMOTE_ADDR'];
$query = mysql_query("SELECT msg_id,message FROM `messages` WHERE uid_fk='$uid' order by msg_id desc limit 1") or die(mysql_error());
$result = mysql_fetch_array($query);
if ($update!=$result['message'])
{
$query = mysql_query("INSERT INTO `messages` (message, uid_fk, ip,created) VALUES ('$update', '$uid', '$ip','$time')") or die(mysql_error());
$newquery = mysql_query("SELECT M.msg_id, M.uid_fk, M.message, M.created, U.username FROM messages M, users U where M.uid_fk=U.uid and M.uid_fk='$uid' order by M.msg_id desc limit 1 ");
$result = mysql_fetch_array($newquery);
return $result;
}
else
{
return false;
}
}
//Delete update
public function Delete_Update($uid, $msg_id)
{
$query = mysql_query("DELETE FROM `comments` WHERE msg_id_fk = '$msg_id' ") or die(mysql_error());
$query = mysql_query("DELETE FROM `messages` WHERE msg_id = '$msg_id' and uid_fk='$uid'") or die(mysql_error());
return true;
}
//Insert Comments
public function Insert_Comment($uid,$msg_id,$comment)
{
$comment=htmlentities($comment);
$time=time();
$ip=$_SERVER['REMOTE_ADDR'];
$query = mysql_query("SELECT com_id,comment FROM `comments` WHERE uid_fk='$uid' and msg_id_fk='$msg_id' order by com_id desc limit 1 ") or die(mysql_error());
$result = mysql_fetch_array($query);
if ($comment!=$result['comment'])
{
$query = mysql_query("INSERT INTO `comments` (comment, uid_fk,msg_id_fk,ip,created) VALUES ('$comment', '$uid','$msg_id', '$ip','$time')") or die(mysql_error());
$newquery = mysql_query("SELECT C.com_id, C.uid_fk, C.comment, C.msg_id_fk, C.created, U.username FROM comments C, users U where C.uid_fk=U.uid and C.uid_fk='$uid' and C.msg_id_fk='$msg_id' order by C.com_id desc limit 1 ");
$result = mysql_fetch_array($newquery);
return $result;
}
else
{
return false;
}
}
//Delete Comments
public function Delete_Comment($uid, $com_id)
{
$query = mysql_query("DELETE FROM `comments` WHERE uid_fk='$uid' and com_id='$com_id'") or die(mysql_error());
return true;
}
}
?>
class Wall_Updates
{
// Updates
public function Updates($uid)
{
$query = mysql_query("SELECT M.msg_id, M.uid_fk, M.message, M.created, U.username FROM messages M, users U WHERE M.uid_fk=U.uid and M.uid_fk='$uid' order by M.msg_id desc ") or die(mysql_error());
while($row=mysql_fetch_array($query))
$data[]=$row;
return $data;
}
//Comments
public function Comments($msg_id)
{
$query = mysql_query("SELECT C.com_id, C.uid_fk, C.comment, C.created, U.username FROM comments C, users U WHERE C.uid_fk=U.uid and C.msg_id_fk='$msg_id' order by C.com_id asc ") or die(mysql_error());
while($row=mysql_fetch_array($query))
$data[]=$row;
if(!empty($data))
{
return $data;
}
}
//Avatar Image
public function Gravatar($uid)
{
$query = mysql_query("SELECT email FROM `users` WHERE uid='$uid'") or die(mysql_error());
$row=mysql_fetch_array($query);
if(!empty($row))
{
$email=$row['email'];
$lowercase = strtolower($email);
$imagecode = md5( $lowercase );
$data="http://www.gravatar.com/avatar.php?gravatar_id=$imagecode";
return $data;
}
else
{
$data="default.jpg";
return $data;
}
}
//Insert Update
public function Insert_Update($uid, $update)
{
$update=htmlentities($update);
$time=time();
$ip=$_SERVER['REMOTE_ADDR'];
$query = mysql_query("SELECT msg_id,message FROM `messages` WHERE uid_fk='$uid' order by msg_id desc limit 1") or die(mysql_error());
$result = mysql_fetch_array($query);
if ($update!=$result['message'])
{
$query = mysql_query("INSERT INTO `messages` (message, uid_fk, ip,created) VALUES ('$update', '$uid', '$ip','$time')") or die(mysql_error());
$newquery = mysql_query("SELECT M.msg_id, M.uid_fk, M.message, M.created, U.username FROM messages M, users U where M.uid_fk=U.uid and M.uid_fk='$uid' order by M.msg_id desc limit 1 ");
$result = mysql_fetch_array($newquery);
return $result;
}
else
{
return false;
}
}
//Delete update
public function Delete_Update($uid, $msg_id)
{
$query = mysql_query("DELETE FROM `comments` WHERE msg_id_fk = '$msg_id' ") or die(mysql_error());
$query = mysql_query("DELETE FROM `messages` WHERE msg_id = '$msg_id' and uid_fk='$uid'") or die(mysql_error());
return true;
}
//Insert Comments
public function Insert_Comment($uid,$msg_id,$comment)
{
$comment=htmlentities($comment);
$time=time();
$ip=$_SERVER['REMOTE_ADDR'];
$query = mysql_query("SELECT com_id,comment FROM `comments` WHERE uid_fk='$uid' and msg_id_fk='$msg_id' order by com_id desc limit 1 ") or die(mysql_error());
$result = mysql_fetch_array($query);
if ($comment!=$result['comment'])
{
$query = mysql_query("INSERT INTO `comments` (comment, uid_fk,msg_id_fk,ip,created) VALUES ('$comment', '$uid','$msg_id', '$ip','$time')") or die(mysql_error());
$newquery = mysql_query("SELECT C.com_id, C.uid_fk, C.comment, C.msg_id_fk, C.created, U.username FROM comments C, users U where C.uid_fk=U.uid and C.uid_fk='$uid' and C.msg_id_fk='$msg_id' order by C.com_id desc limit 1 ");
$result = mysql_fetch_array($newquery);
return $result;
}
else
{
return false;
}
}
//Delete Comments
public function Delete_Comment($uid, $com_id)
{
$query = mysql_query("DELETE FROM `comments` WHERE uid_fk='$uid' and com_id='$com_id'") or die(mysql_error());
return true;
}
}
?>
Collaboration of Previous post.
Live Update and Delete Records with Animation Effect using Jquery and Ajax
Facebook like multi Toggle Comment Box with jQuery and PHP
Submit multiple forms with jQuery and Ajax
Delete Records with Random Animation Effect using jQuery and Ajax
How to add new function/method.
For example if you want to get image path value. Just include the following function inside class Wall_Updates{}. Alter table users add image_path text; public function Image_Path($uid)
{
$result = mysql_query("SELECT image_path FROM users WHERE uid = $uid");
$user_data = mysql_fetch_array($result);
echo $user_data['image_path'];
}
{
$result = mysql_query("SELECT image_path FROM users WHERE uid = $uid");
$user_data = mysql_fetch_array($result);
echo $user_data['image_path'];
}
Display image paht value.
<?php
$Wall = new Wall_Updates();
$image=$Wall->Image_Path($uid);
$user->Image_Path($uid);
echo "<img src='$image'/>"
?>
$Wall = new Wall_Updates();
$image=$Wall->Image_Path($uid);
$user->Image_Path($uid);
echo "<img src='$image'/>"
?>
Installation Video
Note: New subscribers email data update every 18 hours
This is amazing! Wow really liked it.
ReplyDeleteamazing, but i think than the comments schema on database must be comment text
ReplyDeleteReally great work.... But can you help me with a php version problem. I am using php 5.3 and I get the "Function eregi_replace() is deprecated
ReplyDelete"error. Please can you update the toLink function with the preg_replace.?
Thanks a lot mate for your really nice and ellegant work.. Keep up!
download is not working it is going to the tutorial. also why is there no facebook connect for the comments on this site?
ReplyDeleteAmazing : )
ReplyDeleteSrivas, ur the MAN!
ReplyDeleteSubscriber emails update every 18 hours.
ReplyDeleteWhy no facebook connect for comments?
9lessons.info powered by blogger
Good Tutor Srinivas. Its amazing. I like it.
ReplyDeleteIm very very thank to you
Great but what about add pictures with a lightbox ????
ReplyDeleteSalam,..
ReplyDeletehow to add notifications to the email,...
Excelente hermano, mil y mil gracias.
ReplyDeleteHay,
ReplyDeleteThats really usefull ;)
God Bless you.....
ReplyDeleteTable Messages: COMMA MISSED LINE 3
ReplyDeleteTable Comments: COMMA MISSED LINE 3
What do you mean by "... how to display old updates."? Because i didnt find anything related about in this script
Good tutorial! Greetings.
Hello, this post it's really great.
ReplyDeleteHow can I do to refresh the wall, I mean to make a new comment show automatically while the user has opened its wall? Take care.
Hi Everyone ,
ReplyDeleteI would like to implement Parent Child relationship between html tables using Datatables plugin and php.
Could u show me one example how to implement with php and jquery plugin.
Hi evryone,
ReplyDeleteHow to select an image(blob) from the oracle database and how to insert an image into oracle database using php and jquery.
Could u please give me a example.
Very Nice Tutorial and i am proud of u that u r indian
ReplyDeletegreat tutorial, can you make a version with voting system?
ReplyDeleteanybody else encountered 'Parse error: syntax error, unexpected T_STRING, expecting T_OLD_FUNCTION or T_FUNCTION or T_VAR or '}' in /home/chiggo12/public_html/pwnedbookv4/includes/functions.php on line 10'
ReplyDelete???
Great script thanks
ReplyDeleteFOREIGN KEY(uid_fk) REFERENCES users(uid)
ReplyDeletethis is not working
I can use only primary key , unique key & index key plz help me
@SmitDesai Create 'Users' tables first
ReplyDeleteNote for anybody who may be struggling with this like i was..This is written in PHP5. You must be using PHP5 for this to work.
ReplyDeleteHello, this post it's really great.
ReplyDeleteHow can I do to refresh the wall, I mean to make a new comment show automatically while the user has opened its wall? Take care.
i have uploaded script to my site and changed the db files as explained. The box to insert a message is displayed and messages are stored in my database but not on the page.
ReplyDeleteAnybody know why?
Thanx for your previous help
ReplyDeleteI create all table & given correct locations
but now I get error on index page as below
php_network_getaddresses: getaddrinfo failed: No such host is known.
hi,nice tutorial...
ReplyDeleteIs it possible to combine your Collapsed Comments funtion to this.
Mind to add it to this tutorial??...
Thankx in advance.
@Smit
ReplyDeleteMay be problem with $ip=$_SERVER['REMOTE_ADDR']; meanwhile just disable this line.
sorry but same error again
ReplyDeletephp_network_getaddresses: getaddrinfo failed: No such host is known.
I removed $ip=$_SERVER['REMOTE_ADDR'];
line which is twice in function.php
& yes i would like to tell i upload all this file in zymic.com
is there problem in server ?
Wow.Its really wonderful script on php.This blog really helps visitors understand face wall script.I must appreciate your efforts.
ReplyDelete@Sam
ReplyDeleteCollapsed Comments
http://www.9lessons.info/2009/12/display-collapsed-comments-like.html
Download CSS update replace following css class on css/wall.css
ReplyDelete.stcommenttext
{
margin-left: 45px;
min-height: 40px;
word-wrap: break-word;
overflow: hidden;
padding: 3px;
display: block;
font-size: 11px;
width: 340px;
}
@Anonymous
ReplyDeleteI think its because the page displays messages of either the demo user or logged-in users, so we would need to set a login form...
Srinivas, is it necessary to login as a user to post? If so, how to implement that?
Thanks for the tutorial!
Hi! I visit your blog very often, but I didn't comment your posts until now. The scripts posted here are really great. I'm working with ASP.NET not PHP, and every script I found here is just a good guide to me to do that working in ASP.NET with jQuery of course. Great work, keep going on.
ReplyDeleteHello, how can I implement a automatic refresh like facebook? can you please do a tutorial? it would be really great. please.
ReplyDeletehi, how can i search with (select) the post from all my fiends (only) not the whole database in mysql to post in the wall..? if you know i really appreciate your help...
ReplyDeleteyou do great tutorials, keep going on.
nice tutorial. planing to use in my social project
ReplyDeleteStill having problems with messages and comments not being displayed when the pages is refreshed.
ReplyDeleteAnybody else know a fix to this?
hey sir..!!!
ReplyDeleteI only want status update like that.. real time...
Great tutorial
ReplyDeleteWhy when i put a link of youtube video is cok and in my downloaded code only shows the link?
ReplyDeletehey i know it is very easy .....but i'm dont know about mysql creating table , i download your script ..... and go doing what you say.. but i m getting this error msg : Table 'surban6_world.messages' doesn't exist where suraban6_world is my Mysql Username .. have any idea please help me ....
ReplyDeleteI like this article. it is really nice.
ReplyDeleteRespect for Srinivas, I really like your articles. Maybe it's nice to add a function like:
ReplyDeleteadd a title to the video you add, or text you add. Something like:
I read here a book:
- here the video / picture etc. -
you says that create a table ~~ Actually i dont know about PHP ~ MySql ~ will you post new topic on creating table for user ~ Message ~ comment ?? i will be thankfull if you explain that briefly. please post that soon ....
ReplyDelete@Shuya
ReplyDeleteImport wall.sql file using mysql administrator or PhpMyAdmin
i try to import but it shows ErroR : MySQL said: Documentation
ReplyDelete#1046 - No database selected
@Shuya Please try to learn at least basics.
ReplyDeleteHi, I have a little trouble with this script.
ReplyDeleteAt the time I click on the "update" button it doesn't update the messages, I mean, the message I wrote appears in the database but not on the wall, how can I fix it?
Hello, how can I implement a automatic refresh like facebook? can you please do a tutorial? it would be really great. please.
ReplyDeleteSrinivas,
ReplyDeleteCan you provide a little more detail and how to set this up?
I have created the tables, changed the database settings and uploaded the script.
However, messages are not displayed when the page is refreshed.
Also, in the database, it always shows the 'uid_fk' as '1' regardless of who is logged in and posted the messages.
Your scripts are quality but a little more detail and support is required
@Hopohiggo
ReplyDeleteHave you modified $uid value at session.php
great work mate ..thanks
ReplyDeleteFriends tonight I will release wall script installation screen-cast video.
ReplyDeleteAs always first class. This is one of my fav blogs. Thanks again!
ReplyDeleteOne thing I have noticed, it does fail on HTML validation. I've managed to modify the code and eliminate most of the errors however the code is repeating id's ($msg_id). Have you thought of how to get around this?
ReplyDelete@Bryn
ReplyDeleteYou have to add prefix for IDs. I feel private pages not required W3 validations and SEO standards
@Srinivas
ReplyDeleteI can`t seem to locate where you`ve defined that it only should load the current $Session user messages, and not all messages in the "messages" table.
Hi Srinivas, great tutorial but could you add some details on modifying the javascript? I've had no issues with customising the php and database side but I want to let users put in a name and email before posting. But I can't figure out how to pass the extra variables through your javascript script.
ReplyDelete@Mark
ReplyDeletePlease check my previous post. This is just collaboration of my old posts.
@srinivas
ReplyDeleteThank you for posting the video and clarifying the installation process.
I would like to ammend the script to show all messages. How can this be done?
Hello, how can I implement an automatic refresh like facebook? can you please do a tutorial/add a javascript code for that? it would be really great. please.
ReplyDeleteThanks in advance.
Superb tutorial.
Excellent tutorial, thank you for sharing :)
ReplyDelete@srinivas
ReplyDeletecan u help me? can u tell me what should i code in session.php? please =[
I too am also having problems with the session.php
ReplyDeleteSrinivas, facebook and twitter updates feed time auto. Can you make a tutorial on this.
ReplyDeleteI am following your blog for a long time. You are doing a great work.
Working nice but I have doubt
ReplyDelete1. I attach the whole thing with my register , login , logout , member page .
2. It is working fine .
3. As you told I changed session.php
User authentication success login.php should generate user id value as session.php as
?php
session_start();
$uid=$_SESSION['user_id']; // In demo $uid=1
?
4. problem here is the member is not able to view or comment to the other members post ; will you help me solve this problem ?
Thank you
Hello,
ReplyDeletefirst of all thanks for sharing this awesome script.
It works great but i would like to ask you some things.
1) i want to make it work with TaskFreak users.
i made it and i change the queries.
But in session php i add
$uid = $pDefaultUserId;
where $pDefaultUserId is the user session id of logged user and after this i add a javascript alert of $pDefaultUserId to test it and on the alert i get the right id , but the comments and posts always getting user 0 .
Do you know why is that happening ?
if i add manually $uid 1 or 2 or anything else works great
2) i cannot add greek characters , i tried utf8 database , making all files utf8 , i tried with setnames, urf8 on mysqlquery, but nothing
3) when i add a video , i get the player like your demo , but after some visits on the page, or some refresh i'm not getting any more the player but only the link
4) and finally , is there any pagination for the comments ?
Srinivas are you for hire? i'd like to pay you to implement some of these scripts.
ReplyDelete@Smit
ReplyDeleteFollow this tutorial my labs.9lessons database design
http://www.9lessons.info/2010/04/database-design-create-tables-and.html
Hello,
ReplyDeletefirst of all thanks for sharing this awesome script.
It works great but i would like to ask you some things.
1) i cannot add greek characters , i tried utf8 database , making all files utf8 , i tried with setnames, urf8 on mysqlquery, but nothing
2) and finally , is there any pagination for the comments ?
hi, it's a great script
ReplyDeletebut is there a login script that i can use pleaz
hello,
ReplyDeleteit's a great tutorial is there a login script that i can use with facebook wall 3.0 pleaz
Twitter and Facebook style load more results
ReplyDeletehttp://www.9lessons.info/2009/12/twitter-style-load-more-results-with.html
how to : PHP Login Page Example.
ReplyDeletelock.php
and
pleaz
i tried to use the more result script you make but i can't get it to work. I see with Firebug that i get response from ajax_more.php with the right results , but nothing appears to the main page .
ReplyDeletei add the script to the main index.
i add the limit to the query on functions.php and the
$msg_id=$row['msg_id'];
$message=$row['message'];
Any ideas ? Any guide on how to merge these two scripts ??
I try to create the table with thats sentence:
ReplyDeleteCREATE TABLE `messages` (
`msg_id` int(11) AUTO_INCREMENT PRIMARY KEY,
`message` varchar(255)
`uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(uid_fk) REFERENCES users(uid)
)
but Mysql on Phpmyadmin dont let me create, mysql shows this error:
#1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'uid_fk` int(11),
`ip` varchar(30),
`created` int(11),
FOREIGN KEY(`uid_fk`) R' at line 4
@Olber
ReplyDeleteExecution tables order Users, Messages and finally Comments
Thansk, the error was a "," after mesagge. Srinivas i cant download the files in zip, check the Url of download Script
ReplyDelete@Olber
ReplyDeleteSubscriber mails updated.
@Srinivas
ReplyDeleteI have successfully installed this script, but the wall only shows posts of the logged in user.
How can i change this to show all posts?
@Hoponhiggo
ReplyDeleteYou have to chage the Updates function SQL statement. Read the following link
http://www.9lessons.info/2010/04/database-design-create-tables-and.html
BUG FIX:
ReplyDeleteFor the script to be a real facebook; from function.php on line 12 remove the words in double quotes below.
"and M.uid_fk='$uid'"
Leaving the words in the query results into seen your own posts (messages)
Thankyou, this is really helpful.
ReplyDeleteI have learn a lot form your script.
What if I want to embed an image instead a video?
ReplyDeleteI`ve implemented the script, and I`m experiencing a bug. When another user are adding a message, the youtube embed code disappear and the URL is only showing. Have someone located this bug in the code?
ReplyDeleteI did. Because of this I'm trying to add "Facebook like url extractor" instead.
ReplyDelete@srinivas
ReplyDeleteCould you please do a tutorial on how to implement a friends system to go with this script?
The solution to my problem is to donwload the latest oembed release:
ReplyDeletehttp://code.google.com/p/jquery-oembed/downloads/detail?name=jquery.oembed.1.1.0.zip&can=2&q=
and use this instead
Hello guys, and Srinivas Tamada, i have tried to implement this code and its working fine, the Updates are posting to the DB and are fetched back and displayed, My problem is here,
ReplyDelete$('.commentopen').live("click",function()
{
var ID=$(this).attr("id");
......
How can i make this id unique so that when i click on a "comment" link it opens commen_text_area for that update. Because now its opening for only one no matter the one you click. How do i make this "id" Unique for each update.
I have tried
a href='#' id='' title="comment"
But its not working.
Please help.
[email protected]
Srinivas Tamada I love this script, i have modified to my needs except one issue... i need it to let people make longer posts i have altered the database, but i can't find where in the script that it shortens user input.
ReplyDeletethanks!!!
Im sorry about my last post, it is actually only removing part of the links, I can post as much text if I want, if it is a link it takes out everything past & and the & sign... I have set up a simple form using the same database and the same tolink function, and it stores the information correctly. I also added a field image link, it works as long as there aren't any "special characters" in it, but if there is, it removes it and everything past it... thanks for any advice...judy
ReplyDeleteheh ;)
ReplyDeleteGreat work u did. I modified it my way, but it's not parsing anymore.
Could you explain this, how it's parsing links etc., i need to understand it, that would be more than nice :))
Thank you.
Ok there's bug with oembed.
ReplyDeleteIf I post some link it's parsing, and if I then post some text the link before will not be parsed :/
Any Fixxing Ideas??
i got problem with Arabic it look hebro
ReplyDeleteHi when i click the download button it opens demo page
ReplyDeleteplz update it
@Usman
ReplyDeleteDownload button available header right top.
Hello is giving the script error how to solve this special character to follow the tutorial but the error message continued when I add that has "dog" = "Ã § Ã £ o"? will be error UTF8
ReplyDeleteHi, Your wall script is amazing...
ReplyDeleteNow the problem is i have a table called friends list with fields, id, user_id, rel_id(relation id).
Now i want to integrate friends message to my wall.. How to do that?
Thanks in advance
Nice work you have a great mind.........nice work.
ReplyDeletegreat work!
ReplyDeletehow to do multy sessions
ReplyDeleteHow to creat pagination for the comments?
ReplyDeletecan I split this script between 'form input' with 'load_message.php' in the different file?
ReplyDelete(sorry my english too bad)
good tut
ReplyDeleteI have followed whatever u have said...there is no error in the database..bt wenevr i type and press the update button..nothing is displayed on my wall..please help me with the same...
ReplyDeleteHi, I dont download the script, no email found subscribe below but I subscribe here! what happend please....
ReplyDeletehow would i add an image upload to your script?
ReplyDeletegood excuse.
ReplyDeleteYour script is very good thank you ... but I want to make is that registered users to leave comments on any page you place the application for comment.
Understood to be done through sessions but not to start. I could help with that thanks to research or have an idea where to begin because I'm new to php
Hola saludos de peru.
ReplyDeletegracias por el post es muy bueno!!
ahora mi pregunta disculpa mi ingles no es muy bueno.
1. How I do for anyone to see the comments left byusers.
2. that users do identify to leave your comment
but that the comments look for all, not only for one users. like this comment box. thanks
Hi!
ReplyDeleteSomething is wrong with oembed?
If another post doesnt contain link to video all others post with oembed wont show. Just a link.
The download link is bringing me back to the demo. Is there any download link ?
ReplyDeletesir ihave small peoblem with facebook wall sript...i created login and register page sir...but i could not generate the session id..please send me login.php to generate session id to wall.php...
ReplyDeletethank you
hello,
ReplyDeletehow to create a page with all users?
Hi!
ReplyDeleteMy error the character coding.
Wrong character: áéűúó
This i get: áéűúó
GREAT WORK!!
ReplyDeleteHow can I add the code for the http://www.9lessons.info/2009/12/display-collapsed-comments-like.html to the facebook script. A updated version with that would be nice.
@Janathan Sure. Thank you :)
ReplyDeleteHi,
ReplyDeleteGreat script and job done!
Question:
How can you remake this script so that only users of a certain group can display/add post to th group facebook wall? So FB authentication and connection is needed.
Greeting from cold Belgium :-)
Hello buddy. great tutorial... one more thing though... I am using an array to hold all the users friends. for example
ReplyDelete1, 45, 27, 17, 29, 93
the array is the id of the members... now when I want to only show the status messages for the peoples friends... how can I make the sql match against the array will i have to do another for each ? please give me an example code thanks buddie :)
you are awesome !! thanks
ReplyDeleteThanks for this great tutorials.I looking for that tutorials for my site.Thanks again.
ReplyDeleteI need to support with thai mysql
ReplyDeleteHelp me pls..
thanks but where i get wall.js?
ReplyDeleteis there any way to limit the comment count?
ReplyDeletehey Srinivas.
ReplyDeleteplease help me! i need to made an include in the function file, but i can't when i do it over class it can't find it, and if i do it under public function it still dont work, where can i put a include php in the function file so it works?
Very nice! is it possible to add "view all" comments if there are more than 10 comments???
ReplyDeleteThis script is really super. Tried somebody to put it in a active site, not blog, which have already members? I tried but i have problems with connection to data base . You can advice me Srinivas.Indeesd you are very smart . Sorry for my bad english . You can help me pls ? I put on my site it,s work the script very well and it,s very nice but i have problem with the data base .It,s normal i must make other tables for conections . Ty in advance Dr Srinivas .
ReplyDeleteHi
ReplyDeleteI have download the script but it's not working because that is the FAQ your sql query when execute then raised a error and when any users come to download the files then you did not provide the full script
hi srinivash here is some problem in script, because when we post a new text comment over the youtube or any video link, then video link didnt work.
ReplyDeletehi Srinivas I have problem character " áéűúó "
ReplyDeleteComing week I'm going to release version 4.0
ReplyDeleteFinally :D
ReplyDeleteI hope it will support pagination
where is load_message.php?
ReplyDeletehello thanks for the grt work . i have been using your version 3.0 and now u gonna release version 4.0 please can u also release update from 3.0 to 4.0 so that is easy for people to upgrade the script. thanks frnd
ReplyDeletehey its working fine but how to post comment as a diff. user ???
ReplyDeletehey how to post comment as a diff. user
ReplyDeletehello Srinivas Tamada ,
ReplyDeleteI download the code from the tutorial , it works fine in chrome, but problematic in firefiox6. The comment text of the messages is below the writer's picture. But when i view your online demo, everything works well. Is there any difference between your demo code and the download code?
Thanks
I'm waiting for version 4 :D :D
ReplyDeleteWill support greek characters ?
Show more button on bottom of comments ?
Like ?
Tell us what new :D :D
sir i their is no email update there...whenever i try to download script....plz help...
ReplyDeletewhere is 4.0? :)
ReplyDeleteyeah :( where is it ? :'(
ReplyDeleteI have signed up and trying to download bit it tells me I am not signed up
ReplyDeletehttp://www.9lessons.info/2011/05/facebook-wall-script-with-php-and.html
Sorry but the videos of youtube, i cant insert, can you help me...please
ReplyDeleteerick.fix @ hotmail.com
Youtube api updated. We have to update our jquery.oembed.js plugin.
ReplyDeletethank you admin
ReplyDeletealso is version 4 coming out soon? thanks for this great script btw, i have learn't loads on your site!
ReplyDeleteFacebook Wall Script 4.0 commercial version.
ReplyDeleteMails updated
ReplyDeleteWhen is 4.0 lesson coming?
ReplyDeleteIt's ready demo video pending.
ReplyDeletewill 4.0 have "load more" and paging, how much will it be and how long before release? Thanks
ReplyDeleteWall Script 4.0 can deploy my site and database? or just for blog is Srinivas? I can use for all my users from my site? Tanks in advance
ReplyDeleteif i use facebook accound,how can I?
ReplyDeleteIf I had a comment with "+1", the wall displays "1". The + disappears.
ReplyDeleteCould someone help me to keep the + in my text ?
Thanks.
Exelente, me encanta :D
ReplyDeleteHi Srinivas. I am a regular visitor of 9lessons. Please suggest me how do i add this wall script to a drupal 7 site. I want to show the facebook wallposts of the site owner on his website. How do i do that. Please suggest me
ReplyDeletenice tutorial
ReplyDeletenice, very nice
ReplyDeletehello frnd . thank u for such a nice script . can u plz tell me when u gonna release the version 4 of this script, i am very eager to buy it. thanks again
ReplyDeletewhen is version 4 gonna release??? plz can u tell this
ReplyDelete4.0 Release on 3rd Oct
ReplyDeleteAny word on 4.0?
ReplyDeletebut today is 5th Oct and... :(
ReplyDeleteI'm really curious to see version 4.0
Cant wait until 3rd OCT! :)
ReplyDeleteToday is 5th of october. I am eagerly waiting for that.
ReplyDeletewhat is the syntac highlighter that u are using?
ReplyDeleteWall Script 4.0 is UP
ReplyDeletehttp://www.9lessons.info/2011/05/facebook-wall-script-with-php-and.html
why I have to buy wall script 4.0 if using wall script 3.0 and your posts I can create it easily by myself? :] ihihiiihi
ReplyDelete:D. i using wall script 3. it's greate
ReplyDeleteWhere i can download this files : load_messages.php, load_comments.php, message_ajax.php,comment_ajax.php, delete_message_ajax.php, delete_comment_ajax.php
ReplyDeletePlease help me
for Wall Script 3,
ReplyDeletethe videos of youtube.com, i cant insert, can you help me...please
(Youtube api updated. We have to update our jquery.oembed.js plugin.)<- not working/no change
[email protected]
Hi I really liked this tutorial, I'm testing it because it seems very interesting, if all goes well I'd buy the 4.0 version. I've implemented without any problems, I have only one question, not how to make a user accesses the 'wall' of another user and leave your message or comment here.
ReplyDeleteI thought of creating a 'wall_id' for each 'wall' has an identification number belonging to a particular user so you can access it and leave my message, do not know if this would be the best option. If it should not be variations in multiple files or simply change the 'index.php' would suffice. Can anyone guide me about it? Does version 4.0 details how to do this? Greetings
Hi I really liked this tutorial, I'm testing it because it seems very interesting, if all goes well I'd buy the 4.0 version. I've implemented without any problems, I have only one question, not how to make a user accesses the 'wall' of another user and leave your message or comment here.
ReplyDeleteI thought of creating a 'wall_id' for each 'wall' has an identification number belonging to a particular user so you can access it and leave my message, do not know if this would be the best option. If it should not be variations in multiple files or simply change the 'index.php' would suffice. Can anyone guide me about it? Does version 4.0 details how to do this? Greetings
for Wall Script 3,
ReplyDeletethe videos of youtube.com, i cant insert, can you help me...please
(Youtube api updated. We have to update our jquery.oembed.js plugin.)<- not working/no change
X2
please can you help me
[email protected]
@Youtube updated API HTTP to HTTPs
ReplyDeleteDeleting a post with images doesnt delete the images. How to?
ReplyDeletehey,
ReplyDeletethe scripts are wonderful..how do i make the user see updates from other users???this is because each user is restricted to his/her comments...please help
@shrivina I have integrated your script into mysite I think there is a bug with snapshot.
ReplyDeleteWhen you access wall sometime you have to refresh the page several times before the webcam snapshots work. Do you know why this is happening? this even happens when script is standalone install.
Hi srinivas?great work! How do i allow users to see other users comments and comment on them?
ReplyDeleteHi, this is a very good script, but how do i make it as dynamic users login for posting? as i have seem now it is only fixed to a user session and allow other users to actually comment them
ReplyDeleteI have a problem, I use your script for the Russian theme and I need utf-8, but with a change in the tables does not change, and the output is not that necessary.
ReplyDeleteIf you change the message by hand to the table in Russian, it appears, as if to add to the wall for example, "Привет"("hello") prints "Ð ¿Ñ? Ð ¸ Ð ² ÐμÑ?" How to fix?
Спасибо за сайт, Много всего полезного нашел для своей социальной сети. Жаль этот скрипт купить не могу. Вразработке
ReplyDeleteForeign Language fix.
ReplyDeletehttp://www.9lessons.info/2011/08/foreign-languages-using-mysql-and-php.html
Could you please help us define the owner value for when users posts on friend's wall. I only found a reference in your post and didn't find a defined value.
ReplyDeleteI suscribed to mail list of 9lessons.info and I can´t download the file, why?
ReplyDeletehow to do pagination in my posts?
ReplyDeleteI have been struggling with the sessions.php when i put a value like 1 it works fine but when i do like $uid=SESSION["user_id"] the uid_fk is zero and no comments or messages will be loaded could you please clarify on this?thanks
ReplyDeleteis this real time?
ReplyDeleteHey Srinivas,
ReplyDeleteThanks for another great PHP tutorial. Your code is real clean.
I had a question, when you reload the wall, why does it reload empty? I have set the user id to a session variable and it is storing in the database properly.
Is there a way to make the wall display all posts every time?
Thanks!!
hello Srinivas,
ReplyDeleteI have the same probleme with $uid
they dont work can help me to fix the problem
Hi Srinivas,
ReplyDeletegreat Script!!! Can you please update the subscribers email? Can't wait to get the whole Script :D.
Tanks!!!
Hi!
ReplyDeleteMy error the character coding.
Wrong character: õüäö
This i get: õüäö
I signed up for your infomercials a day ago but i still cant download the wall script. can you plz help me with that?....love ur work btw!!
ReplyDeleteHey, can someone please tell me why i cant download scripts even though i registered a day back? :( help.
ReplyDeletehey Srinivas Tamada! russian and romanian don`t work in your script, why? i change the db charset, but the russian and romanian don`t work, how to fix it?
ReplyDeletei need .zip to version 3.0.! its free ?
ReplyDeleteplease how do i create a sign in and signup script for this plugin
ReplyDeleteHiii Srinivas
ReplyDeleteThanks for another great PHP tutorial. Your code is real clean
I thought this script will post on Facebook wall...but it doesn't
ReplyDeleteI was looking for a Facebook sharer