My last post explained about New Twitter design basic layout with CSS and Jquery. In this post I want to explain how to expand URLs like new Twitter user interface using jquery, ajax and PHP. It is very easy just implementing with oembed jquery media plugin. Take a look at this demo link.
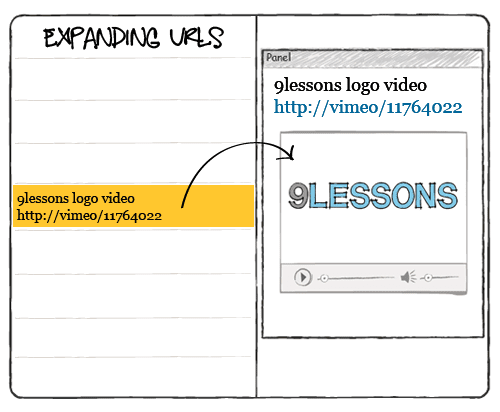


Database
MySQL messages table contains two columns msg_id and message
CREATE TABLE messages
(
msg_id INT PRIMARY KEY AUTO_INCREMENT,
message VARCHAR(150)
);
(
msg_id INT PRIMARY KEY AUTO_INCREMENT,
message VARCHAR(150)
);
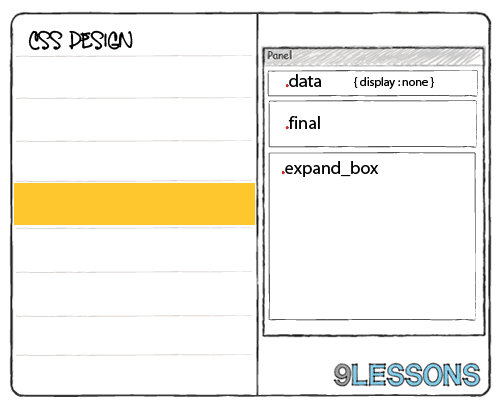
Index.php
Contains simple HTML and PHP code. In left class contains messages table records.
<div id='container'>
// Right part
<div class='right'>
</div>
<div id="panel-frame">
<div class="panel">
<div class="head"> <a href="#" class="close">Close</a></div>
<div class="data">// Message id</div>
<div class="final">// Message </div>
</div>
//Left Part
<div class="left">
// Message display here
<?php
include('db.php');
include('tolink.php');
$sql=mysql_query("select msg_id,message from messages order by msg_id desc");
while($row=mysql_fetch_array($sql))
{
$id=$row['msg_id'];
$message=$row['message'];
$message=tolink($message); // tutorial link click here
?>
<div class="block" id="<?php echo $id;?>">
<?php echo $message;?>
</div>
<?php
}
?>
</div>
// Right part
<div class='right'>
</div>
<div id="panel-frame">
<div class="panel">
<div class="head"> <a href="#" class="close">Close</a></div>
<div class="data">// Message id</div>
<div class="final">// Message </div>
<div class="expand_box">// Expanding URL area</div>
</div></div>
//Left Part
<div class="left">
// Message display here
<?php
include('db.php');
include('tolink.php');
$sql=mysql_query("select msg_id,message from messages order by msg_id desc");
while($row=mysql_fetch_array($sql))
{
$id=$row['msg_id'];
$message=$row['message'];
$message=tolink($message); // tutorial link click here
?>
<div class="block" id="<?php echo $id;?>">
<?php echo $message;?>
</div>
<?php
}
?>
</div>
Javascript
$(".block").click(function(){})- block is the class name of DIV tag. Using $(this).attr('id') - calling DIV tag ID value and $(this).html() - calling DIV tag data. Here you have to include jquery.oembed.js plugin download link
<script type="text/javascript" src="http://ajax.googleapis.com/
ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="jquery.oembed.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$('.block').click(function()
{
var id= $(this).attr('id'); // .block ID
var msg= $(this).html(); // .block DIV data
var data_id= $(".data").html(); // .data DIV value
var panel= $('.panel');
var panel_width=$('.panel').css('left'); // rolling panel width
//-Expanding URLs--------------------------------
$('.data').html(id);
$('.final').html(msg);
function expanding_url()
{
var dataString = 'id='+ id;
$.ajax({
type: "POST",
url: "newtwitterajax.php", // Expanding URL
data: dataString,
cache: false,
success: function(data)
{
$('#expand_box').show();
$('#expand_box').html(data);
}
});
}
//---------------------------------
if(data_id==id)
{
// Rolling Animation
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
//--------------------------------
if(panel_width=='341px')
{
$('#expand_box').hide();
}
else
{
expanding_url();
}
//--------------------------------
}
else
{
expanding_url();
// panel width CSS width:340px + border:1px = 341px
if(panel_width=='341px')
{
// No rolling animation
}
else
{
// Rolling Animation
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
}
}
// passing id value to <div class='data'$gt; </div>
$('.data').html(id);
return false;
});
// panel close link
$('.close').click(function()
{
var panel= $('.panel');
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
$('#expand_box').hide();
return false;
});
});
</script>
ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="jquery.oembed.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$('.block').click(function()
{
var id= $(this).attr('id'); // .block ID
var msg= $(this).html(); // .block DIV data
var data_id= $(".data").html(); // .data DIV value
var panel= $('.panel');
var panel_width=$('.panel').css('left'); // rolling panel width
//-Expanding URLs--------------------------------
$('.data').html(id);
$('.final').html(msg);
function expanding_url()
{
var dataString = 'id='+ id;
$.ajax({
type: "POST",
url: "newtwitterajax.php", // Expanding URL
data: dataString,
cache: false,
success: function(data)
{
$('#expand_box').show();
$('#expand_box').html(data);
}
});
}
//---------------------------------
if(data_id==id)
{
// Rolling Animation
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
//--------------------------------
if(panel_width=='341px')
{
$('#expand_box').hide();
}
else
{
expanding_url();
}
//--------------------------------
}
else
{
expanding_url();
// panel width CSS width:340px + border:1px = 341px
if(panel_width=='341px')
{
// No rolling animation
}
else
{
// Rolling Animation
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
}
}
// passing id value to <div class='data'$gt; </div>
$('.data').html(id);
return false;
});
// panel close link
$('.close').click(function()
{
var panel= $('.panel');
panel.animate({left: parseInt(panel.css('left'),0) == 0 ? +panel.outerWidth() : 0});
$('#expand_box').hide();
return false;
});
});
</script>
newtwitterajax.php
Contains PHP and javascript code. Here calling message record from messages table where msg_id = .block id and calling oembed JavaScript function. If you want to display more details about message(Retweet information) write your code.
<?php
include('db.php');
if($_POST)
{
$id=$_POST['id'];
$sql=mysql_query("select message from messages where msg_id='$id'");
$row=mysql_fetch_array($sql);
$message=$row['message'];
?>
<script type="text/javascript">
$(document).ready(function()
{
$("#expand_url<?PHP echo $id; ?>").oembed("<?php echo $message; ?>", {maxWidth: 300, maxHeight: 200});
});
</script>
<div id='expand_url<?php echo $id; ?>' ></div>
<?php
}
?>
include('db.php');
if($_POST)
{
$id=$_POST['id'];
$sql=mysql_query("select message from messages where msg_id='$id'");
$row=mysql_fetch_array($sql);
$message=$row['message'];
?>
<script type="text/javascript">
$(document).ready(function()
{
$("#expand_url<?PHP echo $id; ?>").oembed("<?php echo $message; ?>", {maxWidth: 300, maxHeight: 200});
});
</script>
<div id='expand_url<?php echo $id; ?>' ></div>
<?php
}
?>
CSS
.data
{
font-size:16px;
display:none;
}
.final
{
overflow:hidden;
padding:20px;
font-size:24px;
}
#expand_box
{
position:relative;
position:absolute;
padding-left:30px;
}
#expand_box img { width:250px; }
{
font-size:16px;
display:none;
}
.final
{
overflow:hidden;
padding:20px;
font-size:24px;
}
#expand_box
{
position:relative;
position:absolute;
padding-left:30px;
}
#expand_box img { width:250px; }
db.php
PHP database configuration file
<?php
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
Cool .. convert link on the fly :D
ReplyDeletekindly look at d design in IE 7.. it sucks
ReplyDelete@Karthikeyan
ReplyDeleteIE Dead
Hi Srinivas
ReplyDeleteI have tested this script in IE7 and IE6. The floating window is not appearing as expected.
Thanks
IE ?
ReplyDeleteI have a Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource here:
ReplyDeletewhile($row=mysql_fetch_array($sql))
How can i fix it :(?
I have found the error:
ReplyDelete$sql=mysql_query("select msg_id,message from messages where order by msg_id");
Bye "where"
$sql=mysql_query("select msg_id,message from messages order by msg_id");
Greetings ^^
IE is the only thing under 10 that michael jackson didn't get his hands on.
ReplyDeleteDemo Modification @Danilo Thanks!
ReplyDelete$sql=mysql_query("select msg_id,message from messages where order by msg_id");
Bye "where"
$sql=mysql_query("select msg_id,message from messages order by msg_id");
IE 8 supporting ..
ReplyDeleteits a cool...
ReplyDeletethumbsup...
we...ee...eee...^^
Will the picture is how to design? Use what software?
ReplyDeleteAdobe illustrator
ReplyDeletegreat one ! Thanks for sharing. I have added this code to fix the IE 7 issue.... agree that IE is dead but few clients still using and can not go back and tell them "IE is the only thing under 10 that michael jackson didn't get his hands on." like PuaHate suggested :-)
ReplyDeleteHello, what is the code tolink.php file?
ReplyDeleteThis is awesome. Just wondering how to make the block active when the slider is open and inactive with it closes. Maybe an addClass().
ReplyDeleteHow can I expand the panel´s width? Doing in the CSS makes a problem with the open/close effect.
ReplyDeleteHelp!
This is awesome. Just wondering how to make the block active when the slider is open and inactive with it closes? Maybe an addClass().
ReplyDeleteThis is awesome. Just wondering how to make the block active when the slider is open and inactive with it closes? Maybe an addClass().
ReplyDeleteCould someone solve this problem?
Pleaseeeeee :P
yep... you can do that with an addClass and removeClass used acordingly
ReplyDeleteHow can we add a loader.gif while opening the data on the window?
ReplyDeletethanks
It's cool but there is no infinite scroll implemented...for example
ReplyDeleteoembed jquery for jquery 1.4.4
ReplyDeleteOn line 200:
var oembed = $.extend(data);
You could just write
var oembed = data;
as the $.getJSON already returns a js object.
Leeas =)
Srinivas,
ReplyDeleteTo learn more about using jquery, whats the reason for not working in IE7, for example? Is it because a part of the code? Which? I would like to understand why,meven knowing that IE is dead.
Thanks from Brasil and your blog is the best!
how do i add data to the sql bases
ReplyDeletei've found error like this :
ReplyDeleteFatal error: Call to undefined function tolink() in C:\xampp\htdocs\test\9lessons\NewTwitter_Expand\index.php on line 241
i think you missed "tolink" function
@Budi
ReplyDeleteTo_link function available here
http://www.9lessons.info/2009/09/urls-as-links-with-regular-expressions.html
Hi, i am trying to implement the twitter load more link with this script but once you load more it does not allow me to expand the new div's loaded.
ReplyDeleteregards
ps. great work
As I see only Vimeo video display, youtube and others DO not is it any error or it was not made wor youtube and etc ... ?
ReplyDeleteI GET THIS ERROR CAN ANYONE HELP ME.
ReplyDeleteWarning: mysql_fetch_array() expects parameter 1 to be resource, boolean given in /home/content/42/8839342/html/twitter/index.php on line 236
Thanks for sharing!!!
ReplyDelete