Facebook is the new Web planet, nowadays it’s a part of human life. Facebook offering user data access via Graph API. This is very helpful for start-up web projects to quickly collecting people data. This post explains you how to request Facebook login, permissions,reading user data and updating Wall using Facebook SDK. Tutorial contains multiple demos try all these Thanks!.
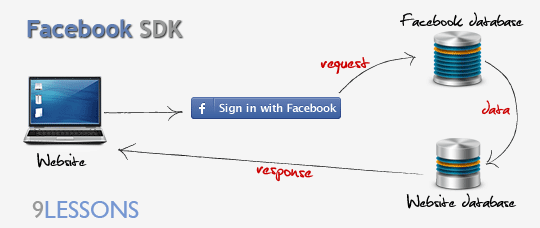
The tutorial contains lib directory contains facebook sdk and database config files with PHP files.
lib
-- facebook.php //Facebook SDK
-- base_facebook.php
-- fbconfig.php //Facebook Application configuration
-- db.php //Database connection
fblogin.php
home.php
status_update.php
-- facebook.php //Facebook SDK
-- base_facebook.php
-- fbconfig.php //Facebook Application configuration
-- db.php //Database connection
fblogin.php
home.php
status_update.php
Database
Sample database users table columns id,facebook_id, name, email etc..
CREATE TABLE users
(
id INT PRIMARY KEY AUTO_INCREMENT,
facebook_id INT(20),
name VARCHAR(200),
email VARCHAR(200),
gender VARCHAR(10),
birthday DATE,
location VARCHAR(200),
hometown VARCHAR(200),
bio TEXT,
relationship VARCHAR(30),
timezone VARCHAR(10),
access_token TEXT
);
(
id INT PRIMARY KEY AUTO_INCREMENT,
facebook_id INT(20),
name VARCHAR(200),
email VARCHAR(200),
gender VARCHAR(10),
birthday DATE,
location VARCHAR(200),
hometown VARCHAR(200),
bio TEXT,
relationship VARCHAR(30),
timezone VARCHAR(10),
access_token TEXT
);
Facebook Application
You have to create a application. Facebook will provide you app id and app secret id, just replace in the following code. fbconfig.php
<?php
$facebook_appid='App ID';
$facebook_app_secret='App Secret';
$facebook = new Facebook(array(
'appId' => $facebook_appid,
'secret' => $facebook_app_secret,
));
?>
$facebook_appid='App ID';
$facebook_app_secret='App Secret';
$facebook = new Facebook(array(
'appId' => $facebook_appid,
'secret' => $facebook_app_secret,
));
?>
fblogin.php
Facebook log in request for user details. Just a take a look at the scope, here requesting email and birthday. Storing $userdata value into session $_SESSION['userdata']
<?php
require 'lib/db.php';
require 'lib/facebook.php';
require 'lib/fbconfig.php';
$user = $facebook->getUser();
if ($user)
{
$logoutUrl = $facebook->getLogoutUrl();
try
{
$userdata = $facebook->api('/me');
}
catch (FacebookApiException $e) {
error_log($e);
$user = null;
}
$_SESSION['facebook']=$_SESSION;
$_SESSION['userdata'] = $userdata;
$_SESSION['logout'] = $logoutUrl;
//Redirecting to home.php
header("Location: home.php");
}
else
{
$loginUrl = $facebook->getLoginUrl(array(
'scope' => 'email,user_birthday'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
}
?>
require 'lib/db.php';
require 'lib/facebook.php';
require 'lib/fbconfig.php';
$user = $facebook->getUser();
if ($user)
{
$logoutUrl = $facebook->getLogoutUrl();
try
{
$userdata = $facebook->api('/me');
}
catch (FacebookApiException $e) {
error_log($e);
$user = null;
}
$_SESSION['facebook']=$_SESSION;
$_SESSION['userdata'] = $userdata;
$_SESSION['logout'] = $logoutUrl;
//Redirecting to home.php
header("Location: home.php");
}
else
{
$loginUrl = $facebook->getLoginUrl(array(
'scope' => 'email,user_birthday'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
}
?>
Basic User Information Demo
home.php
Parsing Facebook user details $userdata array values and inserting into users table.
<?php
require 'lib/db.php';
require 'lib/facebook.php';
require 'lib/fbconfig.php';
session_start();
$facebook=$_SESSION['facebook'];
$userdata=$_SESSION['userdata'];
$logoutUrl=$_SESSION['logout'];
//Facebook Access Token
$access_token_title='fb_'.$facebook_appid.'_access_token';
$access_token=$facebook[$access_token_title];
if(!empty($userdata))
{
$facebook_id=$userdata['id'];
$name=$userdata['name'];
$first_name=$userdata['first_name'];
$last_name=$userdata['last_name'];
$email=$userdata['email'];
$gender=$userdata['gender'];
$birthday=$userdata['birthday'];
$location=mysql_real_escape_string($userdata['location']['name']);
$hometown=mysql_real_escape_string($userdata['hometown']['name']);
$bio=mysql_real_escape_string($userdata['bio']);
$relationship=$userdata['relationship_status'];
$timezone=$userdata['timezone'];
mysql_query("INSERT INTO `users` (`facebook_id`, `name`, `email`, `gender`, `birthday`, `location`, `hometown`, `bio`, `relationship`, `timezone`, `access_token`)
VALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')")";
// Update or Post Facebook wall.
include('status_update.php');
}
else
{
header("Location: fblogin.php");
}
?>
require 'lib/db.php';
require 'lib/facebook.php';
require 'lib/fbconfig.php';
session_start();
$facebook=$_SESSION['facebook'];
$userdata=$_SESSION['userdata'];
$logoutUrl=$_SESSION['logout'];
//Facebook Access Token
$access_token_title='fb_'.$facebook_appid.'_access_token';
$access_token=$facebook[$access_token_title];
if(!empty($userdata))
{
$facebook_id=$userdata['id'];
$name=$userdata['name'];
$first_name=$userdata['first_name'];
$last_name=$userdata['last_name'];
$email=$userdata['email'];
$gender=$userdata['gender'];
$birthday=$userdata['birthday'];
$location=mysql_real_escape_string($userdata['location']['name']);
$hometown=mysql_real_escape_string($userdata['hometown']['name']);
$bio=mysql_real_escape_string($userdata['bio']);
$relationship=$userdata['relationship_status'];
$timezone=$userdata['timezone'];
mysql_query("INSERT INTO `users` (`facebook_id`, `name`, `email`, `gender`, `birthday`, `location`, `hometown`, `bio`, `relationship`, `timezone`, `access_token`)
VALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')")";
// Update or Post Facebook wall.
include('status_update.php');
}
else
{
header("Location: fblogin.php");
}
?>
status_update.php
Update Facebook Wall using access_token via Graph API. Log in scope should be status_update take a look at the Post to my Wall code.
<?php
if($_SERVER["REQUEST_METHOD"] == "POST")
{
$status=$_POST['status'];
$facebook_id=$userdata['id'];
$params = array('access_token'=>$access_token, 'message'=>$status);
$url = "https://graph.facebook.com/$facebook_id/feed";
$ch = curl_init();
curl_setopt_array($ch, array(
CURLOPT_URL => $url,
CURLOPT_POSTFIELDS => $params,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_SSL_VERIFYPEER => false,
CURLOPT_VERBOSE => true
));
$result = curl_exec($ch);
echo "Message Updated";
}
?>
//HTML Code
<form method="post" action="">
<textarea name="status"></textarea> <br/>
<input type="submit" value=" Update "/>
</form>
if($_SERVER["REQUEST_METHOD"] == "POST")
{
$status=$_POST['status'];
$facebook_id=$userdata['id'];
$params = array('access_token'=>$access_token, 'message'=>$status);
$url = "https://graph.facebook.com/$facebook_id/feed";
$ch = curl_init();
curl_setopt_array($ch, array(
CURLOPT_URL => $url,
CURLOPT_POSTFIELDS => $params,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_SSL_VERIFYPEER => false,
CURLOPT_VERBOSE => true
));
$result = curl_exec($ch);
echo "Message Updated";
}
?>
//HTML Code
<form method="post" action="">
<textarea name="status"></textarea> <br/>
<input type="submit" value=" Update "/>
</form>
Post to my Wall
Facebook log in scope for updating Facebook wall.
$loginUrl = $facebook->getLoginUrl(
array(
'scope' => 'email,user_birthday,status_update'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
array(
'scope' => 'email,user_birthday,status_update'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
Status Update Demo
Note: Above cases access_token value not static, it's depends on Facebook login session.
Facebook Offline Data Access
Here access_token is static, you can store this into users table. Any time you can do access or update Facebook using this access_token key. But facebook log out would't support so that you have to build native log out system for clearing sessions values as $userdata. Offline Post to my Wall
Here any time you can update the Facebook wall. Just notice Access my data any time in following screen shot.
$loginUrl = $facebook->getLoginUrl(
array(
'scope' => 'email,user_birthday,status_update,offline_access'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
array(
'scope' => 'email,user_birthday,status_update,offline_access'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
Offline Access Demo
9lessons labs is offline access.
Facebook Full Permission
Here I have requested full available scope to Facebook, based on your web project requirement you can select the user permission values. For more information about Facebook permissons read this link.
$loginUrl = $facebook->getLoginUrl(
array(
'scope' => 'user_about_me,user_activities,user_birthday,user_checkins,user_education_history,user_events,user_groups,user_hometown,user_interests,user_likes,user_location,user_notes,user_online_presence,user_photo_video_tags,user_photos,user_relationships,user_relationship_details,user_religion_politics,user_status,user_videos,user_website,user_work_history,email,read_friendlists,read_insights,read_mailbox,read_requests,read_stream,xmpp_login,ads_management,create_event,manage_friendlists,manage_notifications,offline_access,publish_checkins,publish_stream,rsvp_event,sms,publish_actions,manage_pages'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
array(
'scope' => 'user_about_me,user_activities,user_birthday,user_checkins,user_education_history,user_events,user_groups,user_hometown,user_interests,user_likes,user_location,user_notes,user_online_presence,user_photo_video_tags,user_photos,user_relationships,user_relationship_details,user_religion_politics,user_status,user_videos,user_website,user_work_history,email,read_friendlists,read_insights,read_mailbox,read_requests,read_stream,xmpp_login,ads_management,create_event,manage_friendlists,manage_notifications,offline_access,publish_checkins,publish_stream,rsvp_event,sms,publish_actions,manage_pages'
));
echo '<a href="'.$loginUrl.'">Login with Facebook</a>';
Full Access Demo
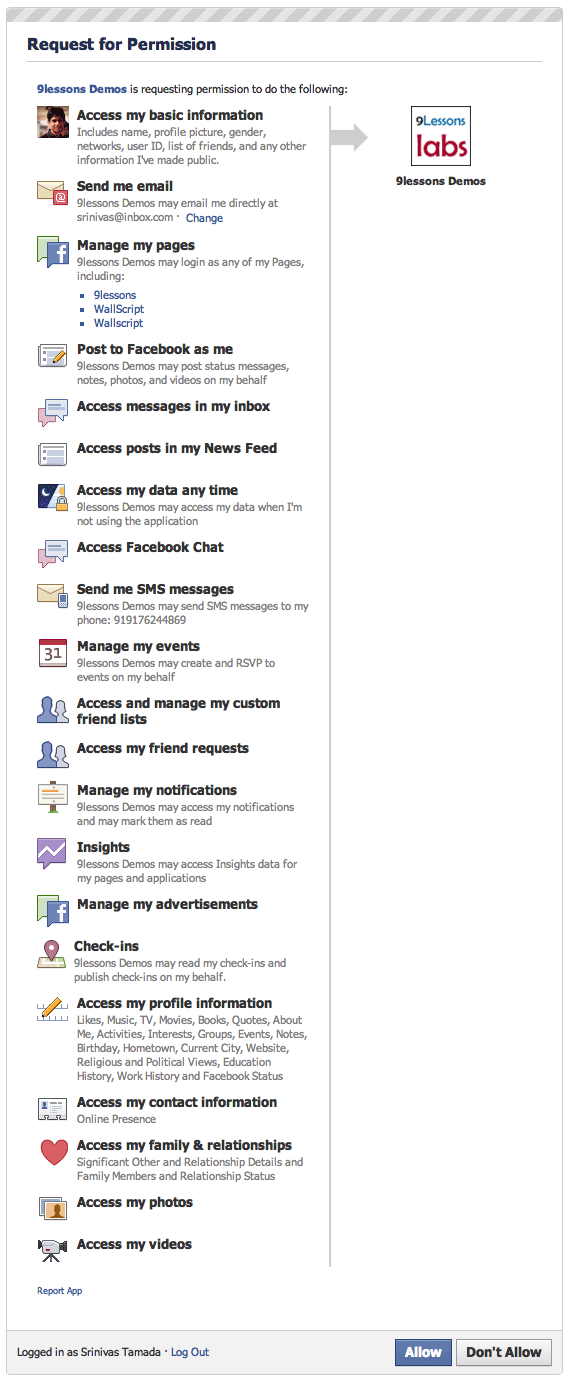
logout.php
Native log out sessions destroy.
<?php
session_start();
$user='';
$userdata='';
session_destroy();
header("Location: fblogin.php");
?>?
session_start();
$user='';
$userdata='';
session_destroy();
header("Location: fblogin.php");
?>?
db.php
PHP database configuration file
<?php
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
$mysql_hostname = "Host name";
$mysql_user = "UserName";
$mysql_password = "Password";
$mysql_database = "Database Name";
$bd = mysql_connect($mysql_hostname, $mysql_user, $mysql_password) or die("Could not connect database");
mysql_select_db($mysql_database, $bd) or die("Could not select database");
?>
Awesome Script!!! Thank you so much.
ReplyDeleteGreat Stuff! Great Stuff! Great Stuff! Thanks
ReplyDeleteLIKE!! super cool script!! thankz :)
ReplyDeleteThank You! Really helpful tutorial. You have shown how to get data like name, birthday etc. but how can I also retrieve information about school (name, year etc.) and other items that are in array ?
ReplyDeleteThanks !
Great tutorial, but can we have a tutorial on facebook like tagging friends in an image...it would be very helpful
ReplyDeleteGetting school information was simple :)
ReplyDelete$education = $userdata['education'];
foreach($education as $edu) {
$school = $edu['school']['name'];
$year = $edu['year']['name'];
$type = $edu['type']['name'];
$conc = $edu['concentration'];
if($conc) {
foreach($conc as $concentration) {
$concentration = $concentration['name'];
}
}
/*echo $school.$year.$type.$concentration;*/
}
Exactly what I was looking for. Can you do the same for the Google+ social network?
ReplyDeleteGreat bindas work it ;)
ReplyDeleteReally Good Work ..
ReplyDeletevery good i like :D
ReplyDeleteThanks for your Script (-: One Question. How can i update my Status when i logout or will post 2 days later? I have all Data in my Database.
ReplyDeleteHey guys, line 11 of home.php -
ReplyDelete$access_token=$facebook[$access_token_title];
- seems to give me an error, any ideas?
It says "Fatal error: Cannot use object of type Facebook as array"
Great job! Thaks to autors. Exelent!
ReplyDeleteFatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\wamp\www\Facebook_Twitter_Login\fbsdk_basic\basic\lib\base_facebook.php:19 Stack trace: #0 C:\wamp\www\Facebook_Twitter_Login\fbsdk_basic\basic\lib\facebook.php(18): require_once() #1 C:\wamp\www\Facebook_Twitter_Login\fbsdk_basic\basic\fblogin.php(5): require('C:\wamp\www\Fac...') #2 {main} thrown in C:\wamp\www\Facebook_Twitter_Login\fbsdk_basic\basic\lib\base_facebook.php on line 19
ReplyDeletepls help....
@Yogesh
ReplyDeleteEnable CURL extension for PHP
hello there,
ReplyDelete$loginUrl = $facebook->getLoginUrl(array(
'scope' => 'email,user_birthday'
));
what u mean by this email and birth day filed..
what value i will replace for this
Please help me
thanks in advance
Very helpful
ReplyDeleteYogesh
ReplyDeletehow to Enable CURL extension for PHP in Zend Server ...
Waoo!! gr8 tutorial
ReplyDeletehow can i do this with canvas?
ReplyDeleteWorking with Facebook SDK Permissions. I can't logout account. What can i do???????????????
ReplyDeletegetting error while creating facebook application...cant verify your account...what does it mean..??..how do i solve????
ReplyDeleteI tried to run it, but I get...
ReplyDeleteWarning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /home/content/50/8522650/html/9lessons/fblogin.php:3) in /home/content/50/8522650/html/9lessons/lib/facebook.php on line 37
Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /home/content/50/8522650/html/9lessons/fblogin.php:3) in /home/content/50/8522650/html/9lessons/lib/facebook.php on line 37
Any hints?
This worked really well for tracking individual logins! I'd like to use this to set up accounts for users and include other values, like game points. Should I simply get rid of the auto-incrementing ID, or will I still run into trouble with the SQL tables? Thanks!!
ReplyDeletei have a solution for your headers already send problem... you have whitespace before the in your PHP code. I struggled with this for several hours. Fix fblogin.php first.
ReplyDeletehttp://forum.mamboserver.com/showthread.php?t=42814
Hi Srinivash,
ReplyDeleteI want to access all the contacts detail of my friends from my gmail account just by giving an email id and password using PHP and JQuery..
[email protected]
hi , i am subscribed member of u r emails. i want to ask how to post user activity of our site to their facebook profile.
ReplyDeletefor example , now a days everybody is using Washington post social reader on facebook . when the user sign in for washigton post , washigton post updates that user profile that the given user is reading their articles which also appears in their friends news feed. So how to do that . Email me at [email protected]
Hi Srinivash,
ReplyDeleteBelow error coming after the login :
"An error occurred with Pawan. Please try again later."
Thanks
Pawan Sharma
hello srinivash. I like this article. but will it be totally secure to login through facebook? because now a days there are many hacking attacks happening in facebook.
ReplyDeleteSo cool dude
ReplyDeleteHey dude.. Its superlike script for facebook permission... But I am little bit confuse about how to get friends email id's.... Is it possible??
ReplyDeleteIf yes then please tell me some thing good way to find it out...
hey some error when login redirect the same page not in home page why? anybody know please help me
ReplyDeleteWarning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /home/..../status_update/fblogin.php:3) in /home/.../status_update/lib/facebook.php on line 37
ReplyDeleteshows this error and redirect to the same page...
Hey where this lib/facebook.php
ReplyDeleteWarning: require(lib/facebook.php) [function.require]: failed to open stream: No such file or directory in C:\wamp\www\FB\fblogin.php on line 3
Fatal error: require() [function.require]: Failed opening required 'lib/facebook.php' (include_path='.;C:\php5\pear') in C:\wamp\www\FB\fblogin.php on line 3
can you help me, how to create NOTEs using Facebook SDK ?
ReplyDeleteI can read note written by my friend, and with public setting.
but I can't read from non-friend or private setting, right?
This is My code to read note
---------------------------------------
$fql = "SELECT uid, note_id, title, content_html, content, created_time, updated_time FROM note WHERE note_id=$note_id";
$params = array(
'method' => 'fql.query',
'query' => $fql,
'callback' => '',
'access_token' => $facebook->getAccessToken()
);
$result = $facebook->api($params);
--------------------------------------------
Now, how to create a note ? confused :(
Example pleasee...
How to solve this error?
ReplyDeleteI just found this error when using Google Chrome, but if I use Firefox, my facebook app running normally and no error appear.
Fatal error: Uncaught Exception: 102:
Requires user session thrown in /home/XXXX/public_html/my_app/fb/base_facebook.php on line 1040
How to delete a status ?
ReplyDeletewhy, after complete login into facebook, fblogin.php not redirect into home.php?
ReplyDeleteIt works for me but it returns nothing and it does't redirect to home.php.
ReplyDeleteHi Srinivas,
ReplyDeleteQuick question, in "access my data anytime" mode... how do I then display the users info (specifically FB pic) throughout my website?
Thanks
George
hey (-:
ReplyDeletehow can i get tzhe user likes?
for example -> all music likes.
i tried it with $userdata['likes'] & $userdata['music'], but it dont work )-:
Hi Srinivas,
ReplyDeleteI created app on facebook, had provided app ID and app secret, but when I clicked on sign in to facebook link , I got an error sayin:
An error occurred with esprit. Please try again later.
API Error Code: 191
API Error Description: The specified URL is not owned by the application
Error Message: Invalid redirect_uri: Given URL is not allowed by the Application configuration.
@Reena
ReplyDeleteTry with registered live URL. Localhost it will not work.
Fatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\xampp\htdocs\basic\lib\base_facebook.php:19 Stack trace: #0 C:\xampp\htdocs\basic\lib\facebook.php(18): require_once() #1 C:\xampp\htdocs\basic\fblogin.php(5): require('C:\xampp\htdocs...') #2 {main} thrown in C:\xampp\htdocs\basic\lib\base_facebook.php on line 19
ReplyDeleteWarning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at C:\xampp\htdocs\basic\fblogin.php:3) in C:\xampp\htdocs\basic\lib\facebook.php on line 37
ReplyDeleteCan you help me, how we get friend list using Facebook SDK ?
ReplyDeleteMy Code is as:
$url="https://graph.facebook.com/".$userdata['id']."/friends?access_token=".$access_token;
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HEADER, 0);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$output = curl_exec($ch);
curl_close($ch);
echo $output;
I am getting error:
{"error":{"message":"(#200) Permissions error","type":"OAuthException"}}
Hi ,
ReplyDeletei want that facebook login page should be open in popwindow.
I some one can help me . .. . ?
Hello, can someone pls provide a code with a popup windows for login instead opening in the same window?
ReplyDeleteHello, the logout you provided doesnt work as it should, it doesnt kill the sesion and doesnt logout from facebook
ReplyDelete?
can u pls modifiy this?
when I try to login with facebook after filling my username and password it shows the following error ----
ReplyDeletehe webpage at https://www.facebook.com/dialog/oauth?client_id=177639729007660&redirect_uri=http%3A%2F%2Fvls1.iitkgp.ernet.in%2Fvls_web%2FFacebook_Twitter_Login%2Flogin-facebook.php&state=2e1fe22226096b629f7ab37f93796e6f&scope=email#_=_ has resulted in too many redirects. Clearing your cookies for this site or allowing third-party cookies may fix the problem. If not, it is possibly a server configuration issue and not a problem with your computer.
Warning: include(../db.php) [function.include]: failed to open stream: No such file or directory in /home/onlyimg/public_html/facebook/test/offline_access/fblogin.php on line 4 why????????????
ReplyDeleteFacebook is never safe.However i liked this info.
ReplyDeleteand it does't redirect to home.php. it doesn't work in localhost? thanks
ReplyDeletei cant retrieve user's friend list ... Is it SDK version problem ??
ReplyDeleteHi
ReplyDeleteGood Work
How can i get email address for facebook friends list using his ID
How can i give this type of permission
Actually this code really needs a lot of errors fixed. At least It finally worked for what I wanted it for and that is writing to the database but you're missing a ")" right before the ";" at the end of this line in home.php
ReplyDeleteVALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')";
It should read:
VALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')");
Needs a ")" before the ";" at the end of this line in home.php
ReplyDeleteVALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')";
it should read:
VALUES('$facebook_id','$name','$email','$gender','$birthday','$location','$hometown','$bio','$relationship','$timezone','$access_token')");
Now it will write to the database...but still needs a lot of work..like the redirects and why it keeps writing to the database..and doesn't show the profile_pic which is what most people would want over any of this.. but nevertheless it is a good idea.
Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /home/outlaw/public_html/facebook/basic/fblogin.php:3) in /home/outlaw/public_html/facebook/basic/lib/facebook.php on line 37
ReplyDeleteRe-directing not working...
ReplyDelete@ Srinivas Tamada
ReplyDeleteYou are using session variables in home.php to remember the data. This can be done by adding -include("fblogin.php") in home.php. Then u do nit hav eto store variables in $_SESSION. Am I correct or will there be any problem ?
Fatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\wamp\www\basic\lib\base_facebook.php:19 Stack trace: #0 C:\wamp\www\basic\lib\facebook.php(18): require_once() #1 C:\wamp\www\basic\fblogin.php(5): require('C:\wamp\www\bas...') #2 {main} thrown in C:\wamp\www\basic\lib\base_facebook.php on line 19
ReplyDeleteFatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\wamp\www\basic\lib\base_facebook.php:19 Stack trace: #0 C:\wamp\www\basic\lib\facebook.php(18): require_once() #1 C:\wamp\www\basic\fblogin.php(5): require('C:\wamp\www\bas...') #2 {main} thrown in C:\wamp\www\basic\lib\base_facebook.php on line 19
ReplyDeleteHi ! Is there a way to auto like a page after auth (opengraph, api, etc...) ? Thanx in advance and great job ;-)
ReplyDeleteThe redirect to home.php doesn't work anymore...
ReplyDeleteCan you please post an updated version of this code for download? The current code does not work properly according to the tutorial.
ReplyDeleteWarning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at C:\xampp\htdocs\fb_connect\offline_access\fblogin.php:3) in C:\xampp\htdocs\fb_connect\offline_access\lib\facebook.php on line 37
ReplyDeletewhats the wrong over here???
hi !
ReplyDeleteI was just implementing this script, but getting an error message as follows..
"Error
An error occurred. Please try again later."
Thanks
Advance
before you sign in is this possible to add sign up option in this demo? please help .
ReplyDeleteHey bro....it's very best script to get the data of FB users...but when m trying to upload this into Fb app m getting page isn't redirecting...gimme a solution.....thanx..
ReplyDeleteHi Srinivas,
ReplyDeleteIm developing an FB app which functionality is to Post message on the Wall of that person who granted access my application even the user is offline. Yet, when I added the offline_access permission on the SCOPE during login and made some test to post something on the wall of that user, no message is being displayed...
I have read that offline_message will be deprecated as that the PUBLISH_STREAM will be used instead. But when I tried again to use this scope, the same happens that no message is posted on the wall if the user is offline.
Any idea to settle this issue? thanks and regards
Hey guys, line 11 of home.php -
ReplyDelete$access_token=$facebook[$access_token_title];
- seems to give me an error, any ideas?
It says "Fatal error: Cannot use object of type Facebook as array"
I created an app which uses users basic information..Now i want to get access to users advanced information..Is it possible and how?
ReplyDeleteHi Srinivas,
ReplyDeleteIts a good blog, every piece of code is working very well... But I got one doubt. I've placed a logout link that I got using "$facebook->getLogoutUrl();" in "home.php". Once I click it, I am logged out of fb. But my application is once again redirecting to "home.php", but I want it to be redirected to "logout.php" How am I supposed to do this?
My name is Lee,
[email protected] is my mail-id.
Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in F:\xampp\htdocs\basic\lib\base_facebook.php:19 Stack trace: #0 F:\xampp\htdocs\basic\lib\facebook.php(18): require_once() #1 F:\xampp\htdocs\basic\fblogin.php(5): require('F:\xampp\htdocs...') #2 {main} thrown in F:\xampp\htdocs\basic\lib\base_facebook.php on line 19
ReplyDeleteFatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\xampp\htdocs\basic\lib\base_facebook.php:19 Stack trace: #0 C:\xampp\htdocs\basic\lib\facebook.php(18): require_once() #1 C:\xampp\htdocs\basic\fblogin.php(5): require('C:\xampp\htdocs...') #2 {main} thrown in C:\xampp\htdocs\basic\lib\base_facebook.php on line 19
ReplyDeleteHello, could you help me? I'm having a configuration error connecting to facebook SDK, presents the following error:
ReplyDeleteFatal error: Cannot use object of type Facebook as array in /home/onbookmarks/www/basic/home.php on line 10
for blogspot or website :(
ReplyDeleteI'm newbie
thanks bro....u saved my day :)
ReplyDeleteFacebook application update with new policy rules, now demos working fine
ReplyDeleteTrying to search for friends by using search box in app ,instead of strolling through them, but keep getting error message 102 requires users session thrown in. Familiar with this error message?
ReplyDeleteI am new to facebook development. I have a question. Can I save the facebook friend list that I get for a particular user in session.
ReplyDeleteConsidering there could be hundreds of friends.
if no what is the right place to save friend list.
please create table use script ..
ReplyDeleteHey, am getting this error Given URL is not allowed by the Application configuration.: One or more of the given URLs is not allowed by the App's settings. It must match the Website URL or Canvas URL, or the domain must be a subdomain of one of the App's domains....... Whats going wrong?
ReplyDeletethis warning is view Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at D:\xampplite\htdocs\jdshaadi\vipin\shaadi\admin\facebookapi\fblogin.php:3) in D:\xampplite\htdocs\jdshaadi\vipin\shaadi\admin\facebookapi\lib\facebook.php on line 39
ReplyDeleteHow can resolve this errors:
ReplyDelete( ! ) Fatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\wamp\www\facebook\lib\base_facebook.php on line 19
( ! ) Exception: Facebook needs the CURL PHP extension. in C:\wamp\www\facebook\lib\base_facebook.php on line 19
your great basu....
ReplyDeletehow to send app message daily on friends wall like daily horoscope in php pls help !!!!!
ReplyDeleteBhai yar mere me login ke bad kuch bhi record db me store nahi ho rahe yar...
ReplyDeletehello i cant print my data on my page after login success so what can i do?
ReplyDeletePlease help!!
ReplyDeleteI am getting this error
[10-Aug-2013 16:21:39 UTC] PHP Fatal error: Cannot use object of type Facebook as array in /.../public_html/test/home.php on line 10
Rachira Jayasuriya .... change this *** $access_token_title='fb_'.$facebook_appid.'_access_token';
ReplyDelete$access_token=$facebook[$access_token_title]; *** with this ***
$access_token = $_SESSION["fb_".$facebook_appid."_access_token"];
$facebook->setAccessToken($access_token);
$access_token = $facebook->getAccessToken();
Awesome Script!!! Thank you so much.
ReplyDeletethanks Srinivas Tamada
ReplyDeletewhat if after i click on login button then it update on my wall say ' Hi ' automatic.
ReplyDeleteHi Srinivas,
ReplyDeleteThanks for this script, now I want to access friends info using this script, i was trying through https://graph.facebook.com/me/friends but i am not able to implement it perfectly so please help me..
Server Error
ReplyDelete500 - Internal server error.
There is a problem with the resource you are looking for, and it cannot be displayed.
what the script to get Access Token ?
ReplyDeleteWould you help me Guy.
ReplyDeleteWhat the script to get Access Token ?
how can i set the scope to get user phone number
ReplyDeletelol this is nothing i make this see in pic no 1 can make this need to buy add me
ReplyDeletehttps://plus.google.com/photos?enfplm&hl=en&utm_source=lmnavbr&utm_medium=embd&utm_campaign=lrnmre&rtsl=1&pid=6000561672611870466&oid=105362770191746233150
thanks
ReplyDeleteplease if someone can help me i having this error each time......i am frustrated if someone please please please help me out
ReplyDeleteerror
Given URL is not allowed by the Application configuration.: One or more of the given URLs is not allowed by the App's settings. It must match the Website URL or Canvas URL, or the domain must be a subdomain of one of the App's domains
The following permissions have not been approved for use and are not being shown to people using your app: user_birthday, user_about_me and user_activities.
ReplyDeletePlease help how can set in developer app,
Plesae Help me
ReplyDeleteThe code Updated to Graph API V2.0 or older version,
if Not Updated, how to update V2.0
Vasanth,
ReplyDeleteI have the same issue, have you resolved this issue.
I am using this scope but getting default permissions
$loginURL = $facebook->getLoginUrl(array( 'scope' => "user_about_me,user_activities,user_birthday,user_checkins,user_education_history,user_events,user_groups,user_hometown,user_interests,user_likes,user_location,user_notes,user_online_presence,user_photo_video_tags,user_photos,user_relationships,user_relationship_details,user_religion_politics,user_status,user_videos,user_website,user_work_history,email,read_friendlists,read_insights,read_mailbox,read_requests,read_stream,xmpp_login,ads_management,create_event,manage_friendlists,manage_notifications,offline_access,publish_checkins, publish_stream,rsvp_event,sms,publish_actions,manage_pages", 'redirect_uri' => REDIRECT_URL));
Please tell it's working fine but i don't understand facebook owner have yet not given permission for me.Please let me know how to do this ?Thanks in advance.
ReplyDeletehelp me Guys
ReplyDeletewhat I do For Permission Access for Desktop Application
I want to use this logic in android, please tell me how it is possible
ReplyDeletei want the code for Full access demo
ReplyDeletecan any one help me???
My comments do not show? Maybe approval is required?...
ReplyDeleteBasically your demo's echo no data? Has Facebook removed the nice looking request? How to show User_About_Me?
Same problem as Timothy here. No data when returns to fblogin.php. No db insert and and no php error. Maybe it's outdated and no longer works. Any help will be appreciated
ReplyDeletescript with full permissions don't work
ReplyDeleteError_log showing : CSRF state token does not match one provided.
ReplyDeleteAfter login it redirects back to fblogin.php . No data comes to database and no eroor in php. PLz help ASAP
How can resolve this errors:
ReplyDelete( ! ) Fatal error: Uncaught exception 'Exception' with message 'Facebook needs the CURL PHP extension.' in C:\wamp\www\facebook\lib\base_facebook.php on line 19
( ! ) Exception: Facebook needs the CURL PHP extension. in C:\wamp\www\facebook\lib\base_facebook.php on line 19
Thanks for the info Srinivas
ReplyDelete