We covered almost all the Open Authentication (OAuth) login systems for Facebook, Twitter, Google plus and Instagram, but unfortunately I missed most important Google Open Authentication login. Today I want explain how to implement this for your web project, this script is very quick and sure it helps you to increase your web project registrations.



Previous Tutorial Login with Google Account OpenID and Login with Google Plus OAuth
Database
Sample database design
CREATE TABLE users
(
id INT PRIMARY KEY AUTO_INCREMENT,
email VARCHAR(50) UNIQUE,
fullname VARCHAR(100),
firstname VARCHAR(50),
lastname VARCHAR(50),
google_id VARCHAR(50),
gender VARCHAR(10),
dob VARCHAR(15),
profile_image TEXT,
gpluslink TEXT
)
(
id INT PRIMARY KEY AUTO_INCREMENT,
email VARCHAR(50) UNIQUE,
fullname VARCHAR(100),
firstname VARCHAR(50),
lastname VARCHAR(50),
google_id VARCHAR(50),
gender VARCHAR(10),
dob VARCHAR(15),
profile_image TEXT,
gpluslink TEXT
)
Step 1: Domain Registration
Add or register your domain at click here.
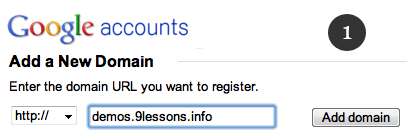
Step 2: Ownership verification
Verify your domain ownership with HTML file upload or including META tag.
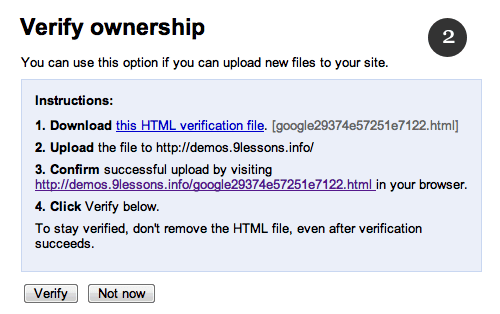
Step 3: OAuth Keys
Google will provide you OAuth consumer key and OAuth consumer secret key.
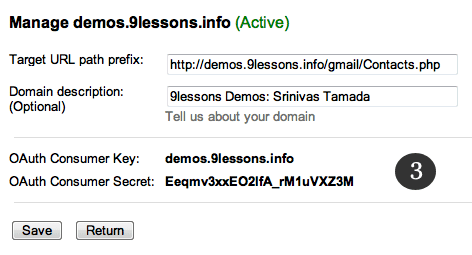
Step 4: Google APIConsole
Create client ID OAuth Console here.
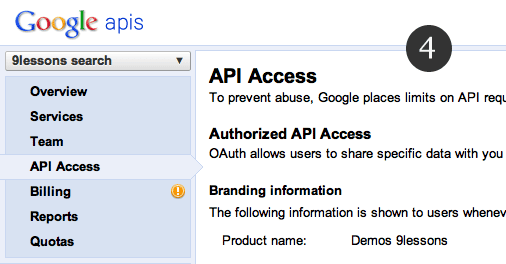
Step 5:
Create client ID.

Step 6
Here the application OAuth client ID and client secret.
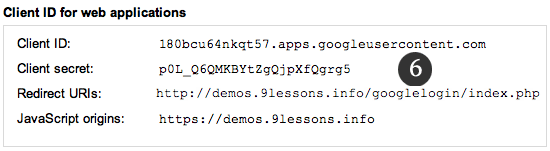
config.php
You can find this in src folder, here you have to configure application OAuth keys, Consumer keys and redirection callback URL.
// OAuth2 Settings, you can get these keys at https://code.google.com/apis/console Step 6 keys
'oauth2_client_id' => 'App Client ID',
'oauth2_client_secret' => 'App Client Secret',
'oauth2_redirect_uri' => 'http://yoursite.com/gplus/index.php',
// OAuth1 Settings Step 3 keys.
'oauth_consumer_key' => 'OAuth Consumer Key',
'oauth_consumer_secret' => 'OAuth Consumer Secret',
'oauth2_client_id' => 'App Client ID',
'oauth2_client_secret' => 'App Client Secret',
'oauth2_redirect_uri' => 'http://yoursite.com/gplus/index.php',
// OAuth1 Settings Step 3 keys.
'oauth_consumer_key' => 'OAuth Consumer Key',
'oauth_consumer_secret' => 'OAuth Consumer Secret',
google_login.php
Google plus login system. Just include the file in index.php
<?php
require_once 'src/apiClient.php';
require_once 'src/contrib/apiOauth2Service.php';
session_start();
$client = new apiClient();
setApplicationName("Google Account Login");
$oauth2 = new apiOauth2Service($client);
if (isset($_GET['code']))
{
$client->authenticate();
$_SESSION['token'] = $client->getAccessToken();
$redirect = 'http://' . $_SERVER['HTTP_HOST'] . $_SERVER['PHP_SELF'];
header('Location: ' . filter_var($redirect, FILTER_SANITIZE_URL));
}
if (isset($_SESSION['token'])) {
$client->setAccessToken($_SESSION['token']);
}
if (isset($_REQUEST['logout'])) {
unset($_SESSION['token']);
unset($_SESSION['google_data']); //Google session data unset
$client->revokeToken();
}
if ($client->getAccessToken())
{
$user = $oauth2->userinfo->get();
$_SESSION['google_data']=$user; // Storing Google User Data in Session
header("location: home.php");
$_SESSION['token'] = $client->getAccessToken();
} else {
$authUrl = $client->createAuthUrl();
}
if(isset($personMarkup)):
print $personMarkup;
endif
if(isset($authUrl))
{
echo "<a class="login" href="$authUrl">Google Account Login</a>";
} else {
echo "<a class="logout" href="?logout">Logout</a>";
}
?>
require_once 'src/apiClient.php';
require_once 'src/contrib/apiOauth2Service.php';
session_start();
$client = new apiClient();
setApplicationName("Google Account Login");
$oauth2 = new apiOauth2Service($client);
if (isset($_GET['code']))
{
$client->authenticate();
$_SESSION['token'] = $client->getAccessToken();
$redirect = 'http://' . $_SERVER['HTTP_HOST'] . $_SERVER['PHP_SELF'];
header('Location: ' . filter_var($redirect, FILTER_SANITIZE_URL));
}
if (isset($_SESSION['token'])) {
$client->setAccessToken($_SESSION['token']);
}
if (isset($_REQUEST['logout'])) {
unset($_SESSION['token']);
unset($_SESSION['google_data']); //Google session data unset
$client->revokeToken();
}
if ($client->getAccessToken())
{
$user = $oauth2->userinfo->get();
$_SESSION['google_data']=$user; // Storing Google User Data in Session
header("location: home.php");
$_SESSION['token'] = $client->getAccessToken();
} else {
$authUrl = $client->createAuthUrl();
}
if(isset($personMarkup)):
print $personMarkup;
endif
if(isset($authUrl))
{
echo "<a class="login" href="$authUrl">Google Account Login</a>";
} else {
echo "<a class="logout" href="?logout">Logout</a>";
}
?>
home.php
Contains PHP code inserting Google plus session details into users table.
<?php
session_start();
include('db.php'); //Database Connection.
if (!isset($_SESSION['google_data'])) {
// Redirection to application home page.
header("location: index.php");
}
else
{
//echo print_r($userdata);
$userdata=$_SESSION['google_data'];
$email =$userdata['email'];
$googleid =$userdata['id'];
$fullName =$userdata['name'];
$firstName=$userdata['given_name'];
$lastName=$userdata['family_name'];
$gplusURL=$userdata['link'];
$avatar=$userdata['picture'];
$gender=$userdata['gender'];
$dob=$userdata['birthday'];
//Execture query
$sql=mysqli_query("insert into users(email,fullname,firstname,lastname,google_id,gender,dob,profile_image,gpluslink) values('$email','$fullName','$firstName','$lastName','$googleid','$gender','$dob','$avatar','$gplusURL')");
}
?>
session_start();
include('db.php'); //Database Connection.
if (!isset($_SESSION['google_data'])) {
// Redirection to application home page.
header("location: index.php");
}
else
{
//echo print_r($userdata);
$userdata=$_SESSION['google_data'];
$email =$userdata['email'];
$googleid =$userdata['id'];
$fullName =$userdata['name'];
$firstName=$userdata['given_name'];
$lastName=$userdata['family_name'];
$gplusURL=$userdata['link'];
$avatar=$userdata['picture'];
$gender=$userdata['gender'];
$dob=$userdata['birthday'];
//Execture query
$sql=mysqli_query("insert into users(email,fullname,firstname,lastname,google_id,gender,dob,profile_image,gpluslink) values('$email','$fullName','$firstName','$lastName','$googleid','$gender','$dob','$avatar','$gplusURL')");
}
?>
db.php
Database configuration file, modify username, password, database and base url values.
<?php
define('DB_SERVER', 'localhost');
define('DB_USERNAME', 'username');
define('DB_PASSWORD', 'password');
define('DB_DATABASE', 'database');
$connection = @mysqli_connect(DB_SERVER,DB_USERNAME,DB_PASSWORD,DB_DATABASE);
$base_url='http://www.youwebsite.com/email_activation/';
?>
define('DB_SERVER', 'localhost');
define('DB_USERNAME', 'username');
define('DB_PASSWORD', 'password');
define('DB_DATABASE', 'database');
$connection = @mysqli_connect(DB_SERVER,DB_USERNAME,DB_PASSWORD,DB_DATABASE);
$base_url='http://www.youwebsite.com/email_activation/';
?>
Great. Thanks.
ReplyDeleteGood job, only i recommend you, and learning readers dont use never mysql_* functions of php, its better other drivers like PDO. If you can in future posts Srinivas use PDO.
ReplyDeleteRegardings from Valencia - Spain, in WidPlay Project ;)
what about returning clients after registering?
ReplyDeletemysql_ functions are used throughout all tutorials and examples over the net.
ReplyDeletethe developer is responsible for writing the proper code for his project.
Never use an example code for live projects.
The tutorial is only used to grasp the logic behind some action - not to be used "as is".
Other than that .. nice tutorial Mr. Tamada
how to use response_type=token?
ReplyDeleteGreat tutorial. Thanks :)
ReplyDeleteAs always a great tutorial. Well written and easy to follow.
ReplyDeleteJust a personal point, I already have a MySQL database and would like to integrate this function into it... That would be useful to know
Keep the good tutorials coming
I like your tutorial, Is that the API used in this tutorial is the latest version?
ReplyDeleteGreat tutorial srini...good job...
ReplyDeleteGreat tutorial and demo for Google ID authentication! Rock on!
ReplyDelete~Duru
keep up the good work
ReplyDeletewell explained tutorial!!!
ReplyDeleteGrate Thanks!!!!!!
Really good post.
ReplyDeleteJust FYI if anyone have some problem with headers, run session at index.php on first line.
Hello to all!
ReplyDeleteHow can I get someone's bio?
Can you also give me the link to the API documentation for this Google library because I can not find.
Thank you!
Great post Srinivas, this code was really needed after your login by twitter, Facebook script :)
ReplyDeleteyou are always rocking i will try same using java thanks for explanation.....!!!
ReplyDeleteGoogle Feedburner is down new subscribers please be patient
ReplyDeleteGreat..!!!!!
ReplyDeleteGreat tutorial. thank you
ReplyDeleteNice tutorial machi, thanks :)
ReplyDelete@Srinivas Can you do the same thing for Twitter with API 1.1?
ReplyDeleteThank you!
great tutorials
ReplyDeleteGood tutorial.. but 1 bug??
ReplyDeletehttp://demos.9lessons.info/googlelogin/home.php loads even after i log out!!!
I am having the following error:
ReplyDeleteFatal error: Uncaught exception 'apiIOException' with message 'HTTP Error: (0) Couldn't resolve host 'accounts.google.com'' in /home/sellasit/public_html/google-api-php-client/src/io/apiCurlIO.php:127 Stack trace: #0 /home/sellasit/public_html/google-api-php-client/src/auth/apiOAuth2.php(93): apiCurlIO->makeRequest(Object(apiHttpRequest)) #1 /home/sellasit/public_html/google-api-php-client/src/apiClient.php(138): apiOAuth2->authenticate(Array) #2 /home/sellasit/public_html/google-site-verify.php(18): apiClient->authenticate() #3 {main} thrown in /home/sellasit/public_html/google-api-php-client/src/io/apiCurlIO.php on line 127
Any Idea??
thanks but this only use for ssl certificate sites
ReplyDelete
ReplyDeleteImportant: OAuth 1.0 has been officially deprecated as of April 20, 2012. It will continue to work as per our deprecation policy, but we encourage you to migrate to OAuth 2.0 as soon as possible.
Please guide me
Please tell how to change the permissions???
ReplyDeleteVery nice tips, thanks for sharing!
ReplyDeleteThis is simply awsome good work and it helps me alot with my web work
ReplyDeletethanks bro a lot me too from chennai :D
I really appreciate sharing this great post. I like this blog and have bookmarked it. Thumbs up
ReplyDeleteThankyou for this.
ReplyDeleteIts realy cool to use this auth method.
Nice post, thanks
ReplyDeletebagikode.com/jquery
Very nice
ReplyDeletethis there a way to do this with youtube login and get channel info and Name
Hi , i have an error, can you help me?
ReplyDeleteError : invalid_scope
Some requested scopes were invalid. {invalid=[https://www.googleapis.com/auth/oauth2]}
Hi..,
ReplyDeleteGood tutorial. But how can i logout from google using this code? That is, even i logout from ur demo, I can access my other google applications (like gmail, blog, etc) diectly.
Thanks...
good tut
ReplyDeletehow to download i can't able to download this code !!!!
ReplyDeleteThanks Dude !
ReplyDeleteI Want to recovered placelived ( country ) plz help me
ReplyDeletewhen login time google Product is requesting permission to: it's should ask one time, how to do that, any pls help me
ReplyDeleteWarning: include(google_login.php) [function.include]: failed to open stream: Operation not permitted in C:\Inetpub\vhosts\7sinfotech.in\subsite\googlelogin\index.php on line 21
ReplyDeleteWarning: include(google_login.php) [function.include]: failed to open stream: Operation not permitted in C:\Inetpub\vhosts\7sinfotech.in\subsite\googlelogin\index.php on line 21
Warning: include() [function.include]: Failed opening 'google_login.php' for inclusion (include_path='.;./includes;./pear') in C:\Inetpub\vhosts\7sinfotech.in\subsite\googlelogin\index.php on line 21
Error: invalid_scope
ReplyDeleteSome requested scopes were invalid. {invalid=[https://www.googleapis.com/auth/oauth2]}
It doesn't really logout! If I go back to the http://demos.9lessons.info/googlelogin/home.php after logging out it still has me logged in and displays my google account basic info.. any solution?
ReplyDeleteHi,
ReplyDeleteIt's great tutorial! But i have problem with download source :( I have tried with 3 different accounts and nothing :( Could you help me with it?
Best,
Rafal
nice
ReplyDeleteNice script but its toooooo big with many folders and files !! for why ??. 300kb ?? wanna a script with just 2 or 3 files . i found one script but i couldnt get gender and guid from it. this script is easy but BIG.
ReplyDeleteThanks for sharing. i need help, its always asking permission every login. how to skip it?
ReplyDeletehi its a good tutorial it helped me. i want to display all the posts from my google plus page to website. How can i do this? i was able to get only those posts which are posted by the owner. I am trying to fetch the posts posted by others on my timeline in google plus.
ReplyDeleteAny help is greatly appreciated. Thank you.
how to change or reset the consumer key/secret?
ReplyDeleteNice tutorial...
ReplyDeleteI am also using Google api but In my api it asks for two time to login (Only first time or when I delete all the history of my browser including cookies and all).
Can you suggest me any solution?
http://stackoverflow.com/questions/24757810/php-google-api-is-asking-to-log-in-twice
how to get country,city and state in google login
ReplyDeleteho do I add state=THE_STATE_PARAMETERS in the above example?
ReplyDeleteawesome! thanks.
ReplyDeletei am using GOOGLE API with PHP ; My question if user is already logged in from other application or user leave the page;
ReplyDeleteHow can i Re Authenticate it so that my programme asked password
Nice Work, I have use both codigniter or php site
ReplyDeleteGood Work ,Thanks. I have implemented both codigniter or php site , no issues. Thank you very much..............
ReplyDeleteplss update thiss
ReplyDeletecant get age
ReplyDeletei need java script
ReplyDelete