This tutorial explains how to upgrade to Ionic 3 and Angular 4 and how to use signature pad for your application. If you are working with some agreement related project or something which needs some written proof from the customers, we might in need of a signature pad. The combination of Ionic 3 and Angular 4 provides some better features to achieve signature pad. This will allow you to sign/draw something on the application and save that image as data on the screen. Using this tutorial, you can also make a signature pad with Ionic 2 and Angular 3. Why late? Let’s start this small, but most commonly used task in your application.
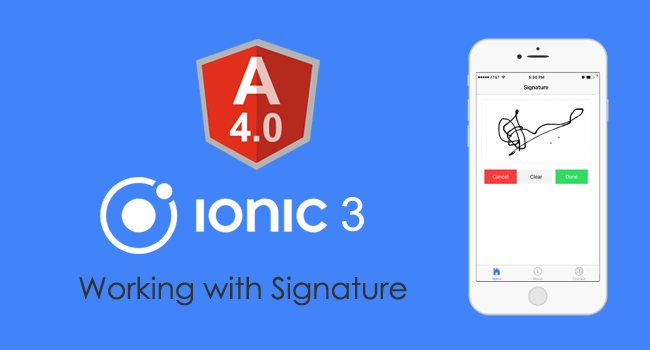
Note: This project works great with Ionic 2 and Angular 2
Home ![]() | Launch ![]() | Capture Signature ![]() |
Angular 4
Recently, Google introduced the latest version of AngularJS which is nothing but Angular 4.0.0. This is a major release and supports backwards compatibility with Angular 2 for most applications. It includes some major improvements and functionality. Angular 4 applications are more faster and smaller. This also have some new features like improved *ngFor and *ngIf which can be used in if/else style syntax. Ionic 3 which is also a new release and comes with Angular 4 support and many other features. Ionic 3 is compatible with Angular 4 and has lot of great features like lazy loading of modules, IonicPage decorator, etc.
Video Tutorial - Ionic 3 and Angular 4: Working with Signature Pad.
Install NodeJS
You need node.js to create a development server, download and install the latest version.
Installing Ionic and Cordova
You will find these instructions in Ionic Framework installation document..
$ npm install -g cordova ionic
$ ionic start --v2 YourAppName tabs
$ cd YourAppName
$ npm install
$ ionic serve
$ ionic start --v2 YourAppName tabs
$ cd YourAppName
$ npm install
$ ionic serve
Open your web browser and launch your application at http://localhost:8100.
Install Angular Signature Pad
This package will help you to capture signature.
$ npm install angular2-signaturepad --save
Generate Ionic Page
You have to create a page for signature model. The following ionic command help you to created automatically.
$ ionic g page signature
Note: Go to src/pages/signature and delete <i>signature.module.ts</i> and rename the class name to SignaturePage
app.module.ts
Now got src/app/app.module.ts and import SignaturePage and Signature Pad component.
import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { AboutPage } from '../pages/about/about';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { SignaturePage } from '../pages/signature/signature';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { SignaturePadModule } from 'angular2-signaturepad';
@NgModule({
declarations: [
MyApp,
AboutPage,
ContactPage,
SignaturePage,
HomePage,
TabsPage
],
imports: [
BrowserModule, SignaturePadModule,
IonicModule.forRoot(MyApp)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
AboutPage,
ContactPage,
HomePage,
SignaturePage,
TabsPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
import { BrowserModule } from '@angular/platform-browser';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { AboutPage } from '../pages/about/about';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { SignaturePage } from '../pages/signature/signature';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { SignaturePadModule } from 'angular2-signaturepad';
@NgModule({
declarations: [
MyApp,
AboutPage,
ContactPage,
SignaturePage,
HomePage,
TabsPage
],
imports: [
BrowserModule, SignaturePadModule,
IonicModule.forRoot(MyApp)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
AboutPage,
ContactPage,
HomePage,
SignaturePage,
TabsPage
],
providers: [
StatusBar,
SplashScreen,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
home.ts
Import signaturePage and ModalController. Here openSingatureModel function will trigger to create ionic modal with Signaturepage
import { Component } from '@angular/core';
import { NavController, NavParams, ModalController } from 'ionic-angular';
import {SignaturePage} from '../signature/signature'
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
public signatureImage : any;
constructor(public navCtrl: NavController, public navParams:NavParams, public modalController:ModalController) {
this.signatureImage = navParams.get('signatureImage');;
}
openSignatureModel(){
setTimeout(() => {
let modal = this.modalController.create(SignaturePage);
modal.present();
}, 300);
}
}
import { NavController, NavParams, ModalController } from 'ionic-angular';
import {SignaturePage} from '../signature/signature'
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
public signatureImage : any;
constructor(public navCtrl: NavController, public navParams:NavParams, public modalController:ModalController) {
this.signatureImage = navParams.get('signatureImage');;
}
openSignatureModel(){
setTimeout(() => {
let modal = this.modalController.create(SignaturePage);
modal.present();
}, 300);
}
}
home.html
Implement open signature button and bind signatureImage value with image source.
<ion-header>
<ion-navbar hideBackButton="true">
<ion-title>Home</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<h2>Welcome to Signature!</h2>
<button ion-button full (click)="openSignatureModel()">
Open Signature
</button>
<div><img [src]="signatureImage" *ngIf="signatureImage" /></div>
</ion-content>
<ion-navbar hideBackButton="true">
<ion-title>Home</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<h2>Welcome to Signature!</h2>
<button ion-button full (click)="openSignatureModel()">
Open Signature
</button>
<div><img [src]="signatureImage" *ngIf="signatureImage" /></div>
</ion-content>
signature.ts
Using NavController redirecting to home page, here using signaturePad builtin functions to perform actions like clearing the signature and capturing the signature data in base64
import { Component, ViewChild } from '@angular/core';
import { NavController} from 'ionic-angular';
import {SignaturePad} from 'angular2-signaturepad/signature-pad';
import {HomePage} from '../home/home';
@Component({
selector: 'page-signature',
templateUrl: 'signature.html',
})
export class SignaturePage {
@ViewChild(SignaturePad) public signaturePad : SignaturePad;
public signaturePadOptions : Object = {
'minWidth': 2,
'canvasWidth': 340,
'canvasHeight': 200
};
public signatureImage : string;
constructor(public navCtrl: NavController) {
}
//Other Functions
drawCancel() {
this.navCtrl.push(HomePage);
}
drawComplete() {
this.signatureImage = this.signaturePad.toDataURL();
this.navCtrl.push(HomePage, {signatureImage: this.signatureImage});
}
drawClear() {
this.signaturePad.clear();
}
}
import { NavController} from 'ionic-angular';
import {SignaturePad} from 'angular2-signaturepad/signature-pad';
import {HomePage} from '../home/home';
@Component({
selector: 'page-signature',
templateUrl: 'signature.html',
})
export class SignaturePage {
@ViewChild(SignaturePad) public signaturePad : SignaturePad;
public signaturePadOptions : Object = {
'minWidth': 2,
'canvasWidth': 340,
'canvasHeight': 200
};
public signatureImage : string;
constructor(public navCtrl: NavController) {
}
//Other Functions
drawCancel() {
this.navCtrl.push(HomePage);
}
drawComplete() {
this.signatureImage = this.signaturePad.toDataURL();
this.navCtrl.push(HomePage, {signatureImage: this.signatureImage});
}
drawClear() {
this.signaturePad.clear();
}
}
signature.html
Modify signature.html witth signature-pad component tag, this will provide you the signaturePad in canvas block.
<ion-header>
<ion-navbar >
<ion-title>Signature</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<signature-pad [options]="signaturePadOptions" id="signatureCanvas"></signature-pad>
<ion-grid>
<ion-row>
<ion-col col-4>
<button ion-button full color="danger" (click)="drawCancel()">Cancel</button>
</ion-col>
<ion-col col-4>
<button ion-button full color="light" (click)="drawClear()">Clear</button>
</ion-col>
<ion-col col-4>
<button ion-button full color="secondary" (click)="drawComplete()">Done</button>
</ion-col>
</ion-row>
</ion-grid>
</ion-content>
<ion-navbar >
<ion-title>Signature</ion-title>
</ion-navbar>
</ion-header>
<ion-content padding>
<signature-pad [options]="signaturePadOptions" id="signatureCanvas"></signature-pad>
<ion-grid>
<ion-row>
<ion-col col-4>
<button ion-button full color="danger" (click)="drawCancel()">Cancel</button>
</ion-col>
<ion-col col-4>
<button ion-button full color="light" (click)="drawClear()">Clear</button>
</ion-col>
<ion-col col-4>
<button ion-button full color="secondary" (click)="drawComplete()">Done</button>
</ion-col>
</ion-row>
</ion-grid>
</ion-content>
signature.scss
asdfasf
page-signature {
signature-pad {
canvas {
border: dashed 1px #cccccc;
width: 100%; height: 200px;
}
}
}
signature-pad {
canvas {
border: dashed 1px #cccccc;
width: 100%; height: 200px;
}
}
}
signature.ts
Include these function in for resizing signature pad canvas.
canvasResize() {
let canvas = document.querySelector('canvas');
this.signaturePad.set('minWidth', 1);
this.signaturePad.set('canvasWidth', canvas.offsetWidth);
this.signaturePad.set('canvasHeight', canvas.offsetHeight);
}
ngAfterViewInit() {
this.signaturePad.clear();
this.canvasResize();
}
let canvas = document.querySelector('canvas');
this.signaturePad.set('minWidth', 1);
this.signaturePad.set('canvasWidth', canvas.offsetWidth);
this.signaturePad.set('canvasHeight', canvas.offsetHeight);
}
ngAfterViewInit() {
this.signaturePad.clear();
this.canvasResize();
}
Video Tutorial - Ionic 3 and Angular 4: Working with Signature Pad.
Nice tut as always!
ReplyDeleteAny Solution???
ReplyDeletenpm ERR! fetch failed https://registry.npmjs.org/npm/-/npm-3.3.9.tgz
npm WARN retry will retry, error on last attempt: Error: connect ETIMEDOUT 151.101.16.162:443
Always cool
ReplyDeleteHow to use resize on orientation change ?
ReplyDeleteyour back button have bug.
ReplyDeletecan i pop page while passing data.
how to add image taking with camera on signature pad ??
ReplyDeleteWhen I try
ReplyDeletethis.signaturePad.set('canvasWidth', canvas.offsetWidth);
then the error is Cannot set property "width" of undefined, telling me that it cannot find this.signaturePad._canvas which is set in the "set" function. Do you know what might cause it?
Not worked for me i got error :
ReplyDeletecore.js:1449 ERROR Error: Uncaught (in promise): Error: Template parse errors:
Can't bind to 'options' since it isn't a known property of 'signature-pad'.
1. If 'signature-pad' is an Angular component and it has 'options' input, then verify that it is part of this module.
2. If 'signature-pad' is a Web Component then add 'CUSTOM_ELEMENTS_SCHEMA' to the '@NgModule.schemas' of this component to suppress this message.
3. To allow any property add 'NO_ERRORS_SCHEMA' to the '@NgModule.schemas' of this component. (