I received a mail from my reader that asked to me how to implement live username availability checking. So I had prepared a simple and understanding tutorial with changing background color using jQuery and Ajax. Take a look at live demo.
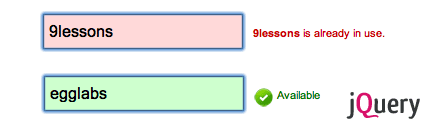


jQuery 1.3 with PHP
user_check.php
$('#username').change(function(){} - username is the ID of the input. Using $("#username").val("id") calling input field value. First checking the value string length max 3 (username.length > 3))
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/
libs/jquery/1.3.0/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$("#username").change(function()
{
var username = $("#username").val();
var msgbox = $("#status");
if(username.length > 3)
{
$("#status").html('<img src="loader.gif"> Checking availability.');
$.ajax({
type: "POST",
url: "check_ajax.php",
data: "username="+ username,
success: function(msg){
$("#status").ajaxComplete(function(event, request){
if(msg == 'OK')
{
// if you don't want background color remove these following two lines
$("#username").removeClass("red"); // remove red color
$("#username").addClass("green"); // add green color
msgbox.html('<img src="yes.png"> <font color="Green"> Available </font>');
}
else
{
// if you don't want background color remove these following two lines
$("#username").removeClass("green"); // remove green color
$("#username").addClass("red"); // add red color
msgbox.html(msg);
}
});
}
});
}
else
{
// if you don't want background color remove this following line
$("#username").addClass("red"); // add red color
$("#status").html('<font color="#cc0000">Enter valid User Name</font>');
}
return false;
});
});
</script>
<input type="text" name="username" id="username" />
<span id="status"></span>
libs/jquery/1.3.0/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function()
{
$("#username").change(function()
{
var username = $("#username").val();
var msgbox = $("#status");
if(username.length > 3)
{
$("#status").html('<img src="loader.gif"> Checking availability.');
$.ajax({
type: "POST",
url: "check_ajax.php",
data: "username="+ username,
success: function(msg){
$("#status").ajaxComplete(function(event, request){
if(msg == 'OK')
{
// if you don't want background color remove these following two lines
$("#username").removeClass("red"); // remove red color
$("#username").addClass("green"); // add green color
msgbox.html('<img src="yes.png"> <font color="Green"> Available </font>');
}
else
{
// if you don't want background color remove these following two lines
$("#username").removeClass("green"); // remove green color
$("#username").addClass("red"); // add red color
msgbox.html(msg);
}
});
}
});
}
else
{
// if you don't want background color remove this following line
$("#username").addClass("red"); // add red color
$("#status").html('<font color="#cc0000">Enter valid User Name</font>');
}
return false;
});
});
</script>
<input type="text" name="username" id="username" />
<span id="status"></span>
check_ajax.php
Contains PHP code.
<?php
include('db.php');
if(isSet($_POST['username']))
{
$username = $_POST['username'];
$username = mysql_real_escape_string($username);
$sql_check = mysql_query("SELECT user_id FROM users WHERE username='$username'");
if(mysql_num_rows($sql_check))
{
echo '<font color="#cc0000"><b>'.$username.'</b> is already in use.</font>';
}
else
{
echo 'OK';
}
}
?>
include('db.php');
if(isSet($_POST['username']))
{
$username = $_POST['username'];
$username = mysql_real_escape_string($username);
$sql_check = mysql_query("SELECT user_id FROM users WHERE username='$username'");
if(mysql_num_rows($sql_check))
{
echo '<font color="#cc0000"><b>'.$username.'</b> is already in use.</font>';
}
else
{
echo 'OK';
}
}
?>
CSS Code
body
{
font-family:Arial, Helvetica, sans-serif
}
#status
{
font-size:11px;
margin-left:10px;
}
.green
{
background-color:#CEFFCE;
}
.red
{
background-color:#FFD9D9;
}
{
font-family:Arial, Helvetica, sans-serif
}
#status
{
font-size:11px;
margin-left:10px;
}
.green
{
background-color:#CEFFCE;
}
.red
{
background-color:#FFD9D9;
}
Just love`it!
ReplyDeleteThanQ !
ReplyDeleteLI love the every effort you put on to expalin, really awesome and useful post,.. thankk u... :)
ReplyDeletethanks so much for this tutorial, I have a different question; How can I get a comment box like this? if you could answer that would be great thanks again
ReplyDeleteJust great, can put timer on image loader by example 3 seconds 2 seconds qherever i want ??
ReplyDeletecan u use timer in the above code... me struggling .. Plz help
ReplyDeleteNice Post,
ReplyDeleteThank you.
great post
ReplyDeleteUseful
ReplyDeleteNice
ReplyDelete:D THX
ReplyDeleteUSEFUL AND NICE.
ReplyDeleteThanks.
A very nice script, though if you try one and its not available and you try another after the loading animation is finished it will show the previous result for a short second. Just thought I would mention it :).
ReplyDeleteA script I will for sure use!
This is awesome! Exactly what I've been looking for for my forms. Well done!
ReplyDeletethank you ,perfect work
ReplyDeletevery nice! thanks for sharing!
ReplyDeleteawesome work man
ReplyDeletehey nice code..use comments so that code will be more understandable..
ReplyDeleteThanks Srinivas!
ReplyDeleteFollowing you on twitter now, this is probably the best page on the net that shows how to use jQuery and other great techniques!
Best Regards
Charlie
Just a tip!
ReplyDeleteYou´ve made a variable stating that status is $("#status) div.
Use that in all places instead of the:
$("#status").html( mg src="loader.gif"> Checking availability.');
like-> status.html('img....'>" Checking availability.');
Thank you for the best learningsite on the web!
nice post
ReplyDeleteliked it thanx
ReplyDeleteAwesome bro, it saved me a life time..
ReplyDeletethank you for sharing..
Not too friendly for someone without too much experience :-(
ReplyDeleteCould not get it to work.
Too complicated....not enough explanation of where things go.
But I'm sure if you're already a web developer with LOT and LOTS of knowledge it's a breeze...so thanks !
thank you for every lesson in your website
ReplyDeleteGoooooooooooooooood!
ReplyDeletemysql_num_rows(): supplied argument is not a valid MySQL result resource in E:\xampp\htdocs\live_availability\check_ajax.php on line 12
ReplyDeletehello... i want to use ur code with jsp.... please tell me how it would be done... i tried it and its working fine in php... i changed the file name to jsp where it is calling the php file(check_ajax.php) but its not working.... please tell me as soon as possible....
ReplyDeletesir , i need your help .may i
ReplyDeleteThis is really nice..Thanks for sharing you knowledge..
ReplyDeletecool
ReplyDeletehow can i check email live availability
ReplyDeletewith validation
Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource in /username_checker_live/check_ajax.php on line 12
ReplyDeleteOK
mysql_num_rows() error means that your sql query has an error. Check for the syntax and also the spelling of your table and field names. also ensure if you have used '$variablename' in quotes.
ReplyDeletenot work for me "Checking availability."
ReplyDeleteit works fine..!!
ReplyDeletebut i have a issue when i use it with form submission.it does prompt error but not stops at that point rather it gives me duplicate entry !! i have used return false .
This is great thank you! How do I add a line of code that automatically prohibits spaces in usernames?
ReplyDeleteThanks its great
ReplyDeleteIt works fine if i check the availability in one table, suppose i want to check in more than one table or the whole database, how can i do it???
ReplyDeleteYour site was very awsome .... I like man
ReplyDeletegood word
ReplyDeleteHello bro,
ReplyDeletefirst time i used it, it worked.
but now, it stacked, indicator checker keep running and running
whats wrong?
Can we do this in spring mvc using only jsp's? Need help with this validation for spring mvc environment and jsp pages.
ReplyDeleteCould'nt be be better. Works perfectly. Thanks Srinivas
ReplyDeleteYou are providing very useful information to us.Thnk u Sir..!
ReplyDelete