Are you working with social applications and user profiles? This post is about implementing dynamic routes for Angular application with basic route validations. This article is a part of Angular routing series for better understanding, please check my previous articles. Here you find importing the dynamic route value and validating with a regular expression for protecting the application routes.
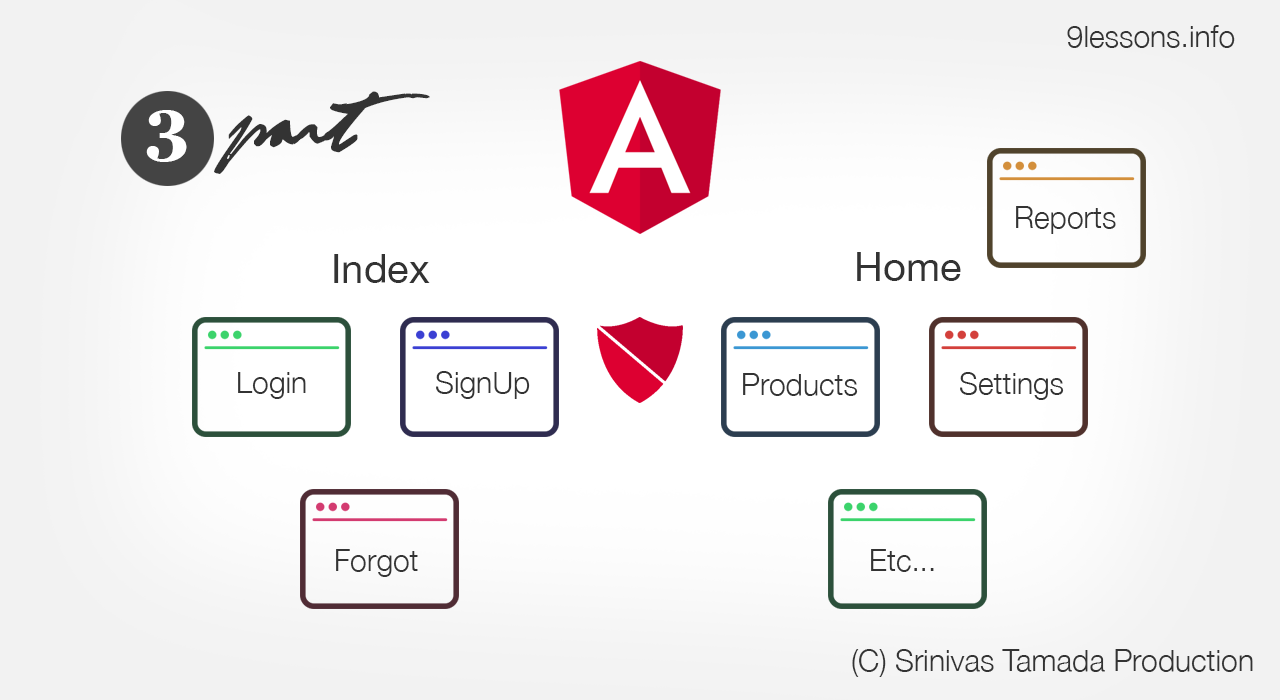
Live Demo
Please login with some values and try the following URL.
Live Demo URL: https://routing.9lessons.info/#/user/srinivas
Live Demo URL2: https://routing.9lessons.info/#/user/srinivas/1
GIT Checkout
Checkout the GIT branch and switch the branch to elector for upcoming steps.
$git clone https://github.com/srinivastamada/angular-routing
$cd angular-routing
$git checkout electron
$npm install
$ng serve
Note: GIT master branch updated with dynamic routes. Please try to checkout old branch and try these steps to practice more.$cd angular-routing
$git checkout electron
$npm install
$ng serve
Previous Parts
Please follow the previous parts for better understanding.
Part Angular Routing using Route Guards
Angular Route Guards with Child Components
Create a User Component for Dynamic Routes
Use Angular command line to generate a new component.
ng generate component home/user
Generates following file.
CREATE src/app/home/user/user.component.scss (0 bytes)
CREATE src/app/home/user/user.component.html (23 bytes)
CREATE src/app/home/user/user.component.spec.ts (614 bytes)
CREATE src/app/home/user/user.component.ts (262 bytes)
UPDATE src/app/home/home.module.ts (580 bytes)
CREATE src/app/home/user/user.component.html (23 bytes)
CREATE src/app/home/user/user.component.spec.ts (614 bytes)
CREATE src/app/home/user/user.component.ts (262 bytes)
UPDATE src/app/home/home.module.ts (580 bytes)
home.routes.ts
Import the component and enable the route under the Home child route.
import { Route } from '@angular/router';
import { AuthGuard } from './../guards/auth.guard';
import { DashboardComponent } from './dashboard/dashboard.component';
import { HomeComponent } from './home.component';
import { ProductsComponent } from './products/products.component';
import { SettingsComponent } from './settings/settings.component';
import { UserComponent } from './user/user.component';
export const HomeRoutes: Route[] = [
{
path: '',
component: HomeComponent,
canActivate: [AuthGuard],
children: [
{ path: '', component: DashboardComponent },
{ path: 'settings', component: SettingsComponent },
{ path: 'products', component: ProductsComponent },
{ path: 'user', component: UserComponent }
]
}
];
Access User Route
Login and test the following user route. Give some values for username and password eg: username: test password: test.
http://localhost:4200/user
Introduce the Dynamic Parameter
Enable the route parameter key. Here username is the key
{ path: 'user/:username', component: UserComponent }
user.component.ts
Using ActivateRoute method, you can subscribe the route parameter value. When you leave the page unsubscribe the event.
import { Component, OnDestroy, OnInit } from '@angular/core;
}
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-user',
templateUrl: './user.component.html',
styleUrls: ['./user.component.scss']
})
export class UserComponent implements OnInit, OnDestroy {
username: string;
private sub: any;
constructor(private activatedRoute: ActivatedRoute) {}
ngOnInit() {
this.sub = this.activatedRoute.paramMap.subscribe(params => {
this.username = params['username'];
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
Login and try accessing the following URL.
http://localhost:4200/user/srinivas
Working with Multiple Dynamic Parameters
Sometime you may need multiple parameters for pagination or etc.
home.routes.ts
Follow the same and include new path for multiple parameters.
import { Route } from '@angular/router';
];
import { AuthGuard } from './../guards/auth.guard';
import { DashboardComponent } from './dashboard/dashboard.component';
import { HomeComponent } from './home.component';
import { ProductsComponent } from './products/products.component';
import { SettingsComponent } from './settings/settings.component';
import { UserComponent } from './user/user.component';
export const HomeRoutes: Route[] = [
{
path: '',
component: HomeComponent,
canActivate: [AuthGuard],
children: [
{ path: '', component: DashboardComponent },
{ path: 'settings', component: SettingsComponent },
{ path: 'products', component: ProductsComponent },
{ path: 'user/:username', component: UserComponent },
{ path: 'user/:username/:id', component: UserComponent }
]
}
user.component.ts
Same way you have to subscribe and get the params values. Here the id is the second parameter.
import { Component, OnDestroy, OnInit } from '@angular/core;
}
import { ActivatedRoute } from '@angular/router';
@Component({
selector: 'app-user',
templateUrl: './user.component.html',
styleUrls: ['./user.component.scss']
})
export class UserComponent implements OnInit, OnDestroy {
username: string;
id: number;
private sub: any;
constructor(private activatedRoute: ActivatedRoute) {}
ngOnInit() {
this.sub = this.activatedRoute.params.subscribe(params => {
this.username = params['username'];
this.id = params['id'];
});
}
ngOnDestroy() {
this.sub.unsubscribe();
}
user.component.html
Bind the values to the HTML.
<h1 class="cap">Welcome {{ username }}</h1>
<p *ngIf="id">Page: {{ id }}</p>
<p *ngIf="id">Page: {{ id }}</p>
Working with Live Domain
When you deploy this on live domain. Direct parameter URLs may not work, because of single page application.
app.module.ts
Enable Angular hash(#) true for route. Now try https://yourdomain.com/#/login
RouterModule.forRoot(routes)
To
To
RouterModule.forRoot(routes, { useHash: true })
Create a Validate Service
Use ng command to generate a new service under services folder(if not just create one). For more information Understanding Regular Expression
ng generate service services/validate
You will find the following files.
CREATE src/app/services/validate.service.spec.ts (386 bytes)
CREATE src/app/services/validate.service.ts (137 bytes)
CREATE src/app/services/validate.service.ts (137 bytes)
validate.service.ts
Here you have to add all the validation rules for path parameters to protect the pages.
import { Injectable } from '@angular/core';
}
@Injectable({
providedIn: 'root'
})
export class ValidateService {
constructor() {}
checkUsername(data: string) {
const pattern = /^[a-zA-Z0-9]{3,25}$/i;
return pattern.test(data);
}
checkID(id: string) {
const pattern = /^[0-9]$/i;
return pattern.test(id);
}
user.component.ts
Included the validate methods to protect the path parameters, if anything wrong just redirect to no page found.
import { Component, OnDestroy, OnInit } from '@angular/core';
import { ActivatedRoute, Router } from '@angular/router';
import { ValidateService } from './../../services/validate.service';
@Component({
selector: 'app-user',
templateUrl: './user.component.html',
styleUrls: ['./user.component.scss']
})
export class UserComponent implements OnInit, OnDestroy {
username: string;
id: string;
private sub: any;
constructor(
private activatedRoute: ActivatedRoute,
private validateService: ValidateService,
private router: Router
) {}
ngOnInit() {
this.sub = this.activatedRoute.paramMap.subscribe(params => {
this.verifyUsername(params.get('username'));
this.verifyID(params.get('id'));
});
}
verifyUsername(data: string) {
if (data && this.validateService.checkUsername(data)) {
this.username = data;
} else {
this.router.navigate(['noPage']);
}
}
verifyID(data: string) {
if (data) {
if (this.validateService.checkID(data)) {
this.id = data;
} else {
this.router.navigate(['noPage']);
}
}
}
ngOnDestroy() {
this.sub.unsubscribe();
}
}
0 comments: