I received a tutorial request from one of my blog readers to implement Ionic Split Pane with the login system. Ionic has been improving and releasing new desktop layout features. This post is an enhancement to my previous application. SplitPane is the new component introduced in Ionic 2.2.0. This targets to create apps of any screen size like desktops and tablets. With this, it is easy to show a side menu with side-by-side navigation controllers. Let’s see how we do this, and follow the demo below for more details.
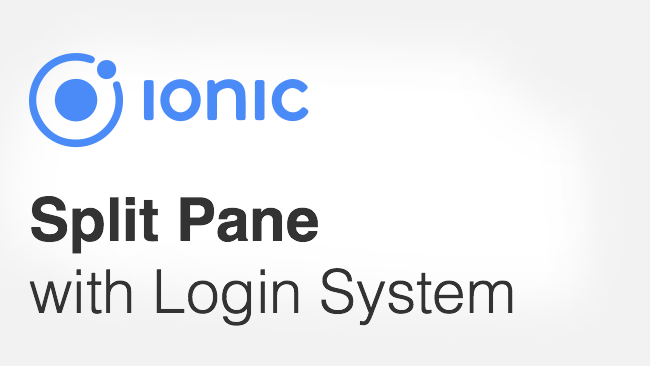
Part 1
Ionic 3 and Angular 4: Create a Welcome Page with Login and Logout.
Video Tutorial
Ionic 3 and Angular 4: Split Pane with Login and Logout System.
Implementing Split Pane
app.html
Go to this file and modify following way.
<ion-nav [root]="rootPage" main #content></ion-nav>
to
<ion-split-pane>
<ion-menu [content]="content">
<ion-header>
<ion-toolbar>
<ion-title>Menu Title</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list> Link 1</ion-list>
<ion-list> Link 2</ion-list>
</ion-content>
</ion-menu>
<!-- the main content -->
<ion-nav [root]="rootPage" main #content></ion-nav>
</ion-split-pane>
<ion-menu [content]="content">
<ion-header>
<ion-toolbar>
<ion-title>Menu Title</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list> Link 1</ion-list>
<ion-list> Link 2</ion-list>
</ion-content>
</ion-menu>
<!-- the main content -->
<ion-nav [root]="rootPage" main #content></ion-nav>
</ion-split-pane>
Modify headers for Home.html, About.html and Contact.html
<ion-header>
<ion-navbar>
<img ion-right src="assets/imgs/bananalogo.png" class="navbarLogo" />
<ion-title>About</ion-title>
</ion-navbar>
</ion-header>
<ion-navbar>
<img ion-right src="assets/imgs/bananalogo.png" class="navbarLogo" />
<ion-title>About</ion-title>
</ion-navbar>
</ion-header>
to
<ion-header>
<ion-navbar>
<button ion-button menuToggle>
<ion-icon name="menu"></ion-icon>
</button>
<img ion-right src="assets/imgs/bananalogo.png" class="navbarLogo" />
<ion-title>About</ion-title>
</ion-navbar>
</ion-header>
<ion-navbar>
<button ion-button menuToggle>
<ion-icon name="menu"></ion-icon>
</button>
<img ion-right src="assets/imgs/bananalogo.png" class="navbarLogo" />
<ion-title>About</ion-title>
</ion-navbar>
</ion-header>
Avoiding Split Pane for Welcome, Login and Signup Pages
Creating a new provider
$ionic g provider splitPane
split-pane.ts
Here we are checking the state that user data present in locat storge or not. Watch the video tutorial you will understand more.
import {Injectable} from '@angular/core';
import {Platform} from 'ionic-angular';
@Injectable()
export class SplitPane {
splitPaneState : boolean;
constructor(public platform : Platform) {
this.splitPaneState = false;
}
getSplitPane() {
if (localStorage.getItem('userData')) {
if (this.platform.width() > 900) {
this.splitPaneState = true;
} else {
this.splitPaneState = false;
}
} else {
this.splitPaneState = false;
}
return this.splitPaneState;
}
}
import {Platform} from 'ionic-angular';
@Injectable()
export class SplitPane {
splitPaneState : boolean;
constructor(public platform : Platform) {
this.splitPaneState = false;
}
getSplitPane() {
if (localStorage.getItem('userData')) {
if (this.platform.width() > 900) {
this.splitPaneState = true;
} else {
this.splitPaneState = false;
}
} else {
this.splitPaneState = false;
}
return this.splitPaneState;
}
}
app.module.ts
Importing new plugins in the application module. Now go to src/app/app.module.ts and import SplitPane for checking the Split Pane state .
import { NgModule, ErrorHandler } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpModule } from '@angular/http';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { AuthService } from '../providers/auth-service';
import { SplitPane } from '../providers/split-pane';
import { Welcome } from '../pages/welcome/welcome';
import { Login } from '../pages/login/login';
import { Signup } from '../pages/signup/signup';
import { AboutPage } from '../pages/about/about';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { LinkyModule } from 'angular-linky';
import { MomentModule } from 'angular2-moment';
@NgModule({
declarations: [
MyApp,
AboutPage,
ContactPage,
HomePage,
Welcome,
Login,
Signup,
TabsPage
],
imports: [
BrowserModule, HttpModule, LinkyModule, MomentModule,
IonicModule.forRoot(MyApp)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
AboutPage,
ContactPage,
HomePage,
Welcome,
Login,
Signup,
TabsPage
],
providers: [
StatusBar,
SplashScreen, AuthService, SplitPane,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
import { BrowserModule } from '@angular/platform-browser';
import { HttpModule } from '@angular/http';
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { AuthService } from '../providers/auth-service';
import { SplitPane } from '../providers/split-pane';
import { Welcome } from '../pages/welcome/welcome';
import { Login } from '../pages/login/login';
import { Signup } from '../pages/signup/signup';
import { AboutPage } from '../pages/about/about';
import { ContactPage } from '../pages/contact/contact';
import { HomePage } from '../pages/home/home';
import { TabsPage } from '../pages/tabs/tabs';
import { StatusBar } from '@ionic-native/status-bar';
import { SplashScreen } from '@ionic-native/splash-screen';
import { LinkyModule } from 'angular-linky';
import { MomentModule } from 'angular2-moment';
@NgModule({
declarations: [
MyApp,
AboutPage,
ContactPage,
HomePage,
Welcome,
Login,
Signup,
TabsPage
],
imports: [
BrowserModule, HttpModule, LinkyModule, MomentModule,
IonicModule.forRoot(MyApp)
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
AboutPage,
ContactPage,
HomePage,
Welcome,
Login,
Signup,
TabsPage
],
providers: [
StatusBar,
SplashScreen, AuthService, SplitPane,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
app.component.ts
Import the SplicPage provider.
import {Component} from '@angular/core';
import {Platform, NavController, App, MenuController} from 'ionic-angular';
import {StatusBar} from '@ionic-native/status-bar';
import {SplashScreen} from '@ionic-native/splash-screen';
import {SplitPane} from '../providers/split-pane';
import {Welcome} from '../pages/welcome/welcome';
@Component({templateUrl: 'app.html'})
export class MyApp {
rootPage : any = Welcome;
public userDetails : any;
constructor(platform : Platform, statusBar : StatusBar, splashScreen : SplashScreen, public splitPane : SplitPane) {
platform.ready().then(() => {
statusBar.styleDefault();
splashScreen.hide();
});
}
}
import {Platform, NavController, App, MenuController} from 'ionic-angular';
import {StatusBar} from '@ionic-native/status-bar';
import {SplashScreen} from '@ionic-native/splash-screen';
import {SplitPane} from '../providers/split-pane';
import {Welcome} from '../pages/welcome/welcome';
@Component({templateUrl: 'app.html'})
export class MyApp {
rootPage : any = Welcome;
public userDetails : any;
constructor(platform : Platform, statusBar : StatusBar, splashScreen : SplashScreen, public splitPane : SplitPane) {
platform.ready().then(() => {
statusBar.styleDefault();
splashScreen.hide();
});
}
}
app.html
Implementing SplitPane state. Here getSplitPane will return the state based on the user data present or not.
<ion-split-pane [when]="splitPane.getSplitPane()">
<!-- our side menu -->
<ion-menu [content]="content">
<ion-header>
<ion-toolbar>
<ion-title>Menu</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list>
<button ion-button color="primary" (click)="logout()">Logout</button>
</ion-list>
</ion-content>
</ion-menu>
<!-- the main content -->
<ion-nav [root]="rootPage" main #content></ion-nav>
</ion-split-pane>
<!-- our side menu -->
<ion-menu [content]="content">
<ion-header>
<ion-toolbar>
<ion-title>Menu</ion-title>
</ion-toolbar>
</ion-header>
<ion-content>
<ion-list>
<button ion-button color="primary" (click)="logout()">Logout</button>
</ion-list>
</ion-content>
</ion-menu>
<!-- the main content -->
<ion-nav [root]="rootPage" main #content></ion-nav>
</ion-split-pane>
Logout action for Menu
app.component.ts
Apply logout feature to the applicaiton menu.
import {Component} from '@angular/core';
import {Platform, NavController, App, MenuController} from 'ionic-angular';
import {StatusBar} from '@ionic-native/status-bar';
import {SplashScreen} from '@ionic-native/splash-screen';
import {SplitPane} from '../providers/split-pane';
import {Welcome} from '../pages/welcome/welcome';
@Component({templateUrl: 'app.html'})
export class MyApp {
rootPage : any = Welcome;
public userDetails : any;
constructor(platform : Platform, statusBar : StatusBar, splashScreen : SplashScreen, public splitPane : SplitPane, public app : App, public menu : MenuController) {
platform.ready().then(() => {
statusBar.styleDefault();
splashScreen.hide();
});
}
backToWelcome() {
const root = this.app.getRootNav();
root.popToRoot();
}
logout() {
//Api Token Logout
localStorage.clear();
this.menu.enable(false);
setTimeout(() => this.backToWelcome(), 1000);
}
}
import {Platform, NavController, App, MenuController} from 'ionic-angular';
import {StatusBar} from '@ionic-native/status-bar';
import {SplashScreen} from '@ionic-native/splash-screen';
import {SplitPane} from '../providers/split-pane';
import {Welcome} from '../pages/welcome/welcome';
@Component({templateUrl: 'app.html'})
export class MyApp {
rootPage : any = Welcome;
public userDetails : any;
constructor(platform : Platform, statusBar : StatusBar, splashScreen : SplashScreen, public splitPane : SplitPane, public app : App, public menu : MenuController) {
platform.ready().then(() => {
statusBar.styleDefault();
splashScreen.hide();
});
}
backToWelcome() {
const root = this.app.getRootNav();
root.popToRoot();
}
logout() {
//Api Token Logout
localStorage.clear();
this.menu.enable(false);
setTimeout(() => this.backToWelcome(), 1000);
}
}
Video Tutorial
Ionic 3 and Angular 4: Deploying an Ionic App to Firebase Free Hosting.
Hello,
ReplyDeletehow can I increase the performance of ionic 3 app?
White screen that comes after splash screen to hide that I've increased splash screen duration. Now the splash screen duration is about 10-12 seconds. What's the best solution for this?
run build for production it will improve alot
Deletecool thanks.
ReplyDeleteif i want put on split pane information about user login, how can i do it?
ReplyDeletevery informative article
ReplyDelete